jump with gravity
Author |
Message |
new
|
Posted: Fri Dec 17, 2004 7:40 am Post subject: jump with gravity |
|
|
how can i make a normal oval jump when a key i pressed with gravity and it should fall back vertically. but i want it to move left or right if right or left arrow keys are pressed, or they sud fall back vertically down.
can anyone help me with this..plzz
thx a lot in advance |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Cervantes

|
Posted: Fri Dec 17, 2004 10:19 am Post subject: (No subject) |
|
|
Here's the basic code for jumping, involving gravity. I hope it makes sense to you. If not, feel free to ask questions.
code: |
const gravity := 0.15
var x, y, vy : real
x := maxx / 2
y := 100
var jumping := false
var keys : array char of boolean
loop
Input.KeyDown (keys)
if keys (KEY_UP_ARROW) then
jumping := true
vy := 5
end if
if jumping then
vy -= gravity
y += vy
end if
if y < 100 then
y := 100
end if
cls
drawfilloval (round (x), round (y), 10, 10, black)
delay (10)
end loop
|
I'll leave it to you to add in the left/right movement, and to incorporate double buffers (offscreenonly). |
|
|
|
|
 |
new
|
Posted: Sat Dec 18, 2004 3:32 am Post subject: small problem |
|
|
hi.,,,thx a lot man...the gravity thingi works, but if we keep pressing the up arrow...it keeps going up and then when we realses it..it falls back
how to make it like, when one pressed it goes certain height only and falls bak...then we can press again to jump |
|
|
|
|
 |
Paul
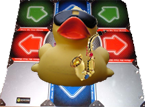
|
Posted: Sat Dec 18, 2004 11:32 am Post subject: (No subject) |
|
|
I'd prefer to keep my jumping in a loop of some kind, and it is activated as a key is pressed. |
|
|
|
|
 |
Cervantes

|
Posted: Sat Dec 18, 2004 12:05 pm Post subject: (No subject) |
|
|
Paul wrote: I'd prefer to keep my jumping in a loop of some kind, and it is activated as a key is pressed.
You mean, a loop within a loop? Or processes? Either way is bad. Former: nothing else will happen when you are jumping. Latter: ...
new wrote: how to make it like, when one pressed it goes certain height only and falls bak...then we can press again to jump
That's easily fixed. |
|
|
|
|
 |
Paul
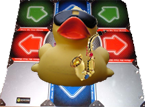
|
Posted: Sat Dec 18, 2004 12:49 pm Post subject: (No subject) |
|
|
hey, I didn't read his other mario thread. As far as jumping with gravity is concerned  |
|
|
|
|
 |
MihaiG

|
Posted: Sun Dec 19, 2004 2:02 pm Post subject: (No subject) |
|
|
i don't seem to get the left motion...of the ball.... and then going down... i can get it too go right but not left....but i also get a problem withwhen i do right it goes back to where i pressed right... i need help |
|
|
|
|
 |
Cervantes

|
Posted: Sun Dec 19, 2004 3:30 pm Post subject: (No subject) |
|
|
It may be just me, but I found that quite incoherant. Try posting your code. That should explain your problem much, much more effectively. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
MihaiG

|
Posted: Sun Dec 19, 2004 4:22 pm Post subject: (No subject) |
|
|
heres the code for right and up(notice: i can only get one direction of motion for one ball... one for each direction and each are attracted to one gravity.. look
code: |
const gravity := 0.1
var x, y, vy, vx, z, vz, q, x1, x2 : real
x := maxx / 2
x1 := 100
y := 100
z := maxx / 2
var jumping := false
var jump := false
var keys : array char of boolean
var key : array char of boolean
loop
Input.KeyDown (keys)
if keys (KEY_UP_ARROW) then
jumping := true
vy := 5
end if
if jumping then
vy -= gravity
y += vy
end if
if y < 100 then
y := 100
end if
cls
drawfilloval (round (x), round (y), 10, 10, black)
delay (10)
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
Input.KeyDown (key)
if key (KEY_RIGHT_ARROW) then
jump := true
vx := 5
end if
if jump then
vx -= gravity
z += vx
end if
if z < maxx / 2 then
z := maxx / 2
end if
cls
drawfilloval (round (z), round (x1), 10, 10, black)
delay (10)
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
end loop
|
|
|
|
|
|
 |
Martin

|
Posted: Sun Dec 19, 2004 4:26 pm Post subject: (No subject) |
|
|
Make two velocity variables for your character (assuming your game is 2d), an x and a y one.
When you press left or right, if you are on the ground, your x velocity is increased (if right is pressed) or decreased (if left is pressed).
When you press up, your y velocity is increased (ie, you jump). The acceleration due to gravity will slow this down. I suggest you use real world measures (metres, seconds), and you then translate them to pixel coordinates.
Don't ever use processes. They are bad, and very unpredictable. There is absolutely NO reason anyone should ever use one (except for Mazer, but we banned him because he was a jerk). |
|
|
|
|
 |
Cervantes

|
Posted: Sun Dec 19, 2004 5:19 pm Post subject: (No subject) |
|
|
Question: why are you using such strange and confusing variable names? You're using z to store the second ball's x position, and you're using x1 to store the second ball's y position. This isn't three dimensional.
Some general coding improvements:
You only need to use Input.KeyDown once inside your loop. You don't need two different arrays (char of boolean) to search for input from two different keys on the keyboard. Rather, just use one array char of boolean and just use one Input.KeyDown. Use if statements to determine if the key you are questioning is being pressed.
Try to keep all your drawing commands together. That means only have one cls; follow that by drawing your two ovals (though when the program is corrected you'll only have one ball); follow that by your delays.
I'm not sure if you've updated your code since Martin's post (I don't see a message edited by note, but I often don't see those when people have edited their posts. Dan?) but I think you may have, since you are using vx.
Right now, you've kinda got a program that simulates a person jumpin off of a black hole. The black hole is located at (maxx / 2, 100). Regardless of which direction the person jumps in, he is always pulled back to the black hole. If you are trying to make a program like what new was trying to do, you want to be on Earth. For the purposes of the program, we will assume that Earth is flat and that gravity pulls everything directly downwards with a constant acceleration regardless of location.
When you're on the surface of the Earth, you can move north, south, east, and west. For the purposes of this two-dimensional program, let's just say we can move east and west. We can, however, also jump up into the air. When we do this, we jump off the surface at a specific velocity. Gravity pulls back at us (it causes us to accelerate downwards).
Let's take a look at some code. It uses only one Input.KeyDown and only one section of drawing commands. It allows the player to jump and to walk around on the surface of the Earth.
code: |
setscreen ("graphics:400;300,offscreenonly")
const gravity := 0.1
var x, y, vy, vx : real
x := maxx / 2
y := 10
var jumping := false
var keys : array char of boolean
loop
Input.KeyDown (keys)
if keys (KEY_UP_ARROW) and ~jumping then
jumping := true
vy := 5
end if
if keys (KEY_RIGHT_ARROW) then
x += 1
end if
if keys (KEY_LEFT_ARROW) then
x -= 1
end if
if jumping then
vy -= gravity
y += vy
end if
if y < 10 then
y := 10
jumping := false
end if
cls
drawfilloval (round (x), round (y), 10, 10, black)
View.Update
delay (5)
end loop
|
Now, the only problem with this is that the ball moves instantly. ie. there is no acceleration. It's velocity is instantly set. For some types of games, this effect can be desired. But let's explore acceleration in the x plane.
It's essentially the same as jumping. Let's look at some more code:
code: |
setscreen ("graphics:400;300,offscreenonly")
const gravity := 0.1
var x, y, vy, vx : real
x := maxx / 2
y := 10
vx := 0
var jumping := false
var keys : array char of boolean
loop
Input.KeyDown (keys)
if keys (KEY_UP_ARROW) and ~jumping then
jumping := true
vy := 5
end if
if keys (KEY_RIGHT_ARROW) then
vx += 0.1
end if
if keys (KEY_LEFT_ARROW) then
vx -= 0.1
end if
if jumping then
vy -= gravity
y += vy
end if
if y < 10 then
y := 10
jumping := false
end if
x += vx
cls
drawfilloval (round (x), round (y), 10, 10, black)
View.Update
delay (5)
end loop
|
note that the ~jumping is the same as not jumping which is the same as jumping = false.
Simple enough, I hope. However, There are two problems with this. First, the ball rolls with the same volcity forever, unless the right / left arrow keys are pressed. We're not travelling in empty space here, so we'll add some friction to the object. To do this, create a constant for friction and assign it a value of 0.99. Then, just before (or after, it doesn't matter too much) you add vx to x, multiply vx by friction. However, since friction when jumping through the air is negligable, we need an if statement to make sure that the player is on the ground. Like this:
code: |
const Friction := 0.99
%----------
x += vx
if ~jumping then
vx *= Friction
end if
|
Good enough. The second problem is that the player can change directions in mid-air. Once again, this can be a desired feature of some games, but let's explore how to fix it so it's more like the real world.
To fix it, wrap an if statement around the keys (KEY_RIGHT_ARROW) and keys (KEY_LEFT_ARROW) if statements. The outer if statement should make sure that the ball is not jumping. If he's not, then it will enter the if statement and allow horizontal movement.
Like this:
code: |
if ~jumping then
if keys (KEY_RIGHT_ARROW) then
vx += 0.1
end if
if keys (KEY_LEFT_ARROW) then
vx -= 0.1
end if
end if
|
So, let's look at our final code:
code: |
setscreen ("graphics:400;300,offscreenonly")
const gravity := 0.1
const Friction := 0.99
var x, y, vy, vx : real
x := maxx / 2
y := 10
vx := 0
var jumping := false
var keys : array char of boolean
loop
Input.KeyDown (keys)
if keys (KEY_UP_ARROW) and ~jumping then
jumping := true
vy := 5
end if
if ~jumping then
if keys (KEY_RIGHT_ARROW) then
vx += 0.1
end if
if keys (KEY_LEFT_ARROW) then
vx -= 0.1
end if
end if
if jumping then
vy -= gravity
y += vy
end if
if y < 10 then
y := 10
jumping := false
end if
x += vx
if ~jumping then
vx *= Friction
end if
cls
drawfilloval (round (x), round (y), 10, 10, black)
View.Update
delay (5)
end loop
|
And there you have it.
It should be noted, however, that a Mario style game, where the player is a man and is walking / running around, would probably benefit most from immediate acceleration (ie. don't use vx). A racing game, on the other hand, where the player drives a car, would benefit most from using acceleration (ie. using vx). Mind you, it would be a minghty strange racing game, given that we're seeing it from the side and that the car can jump into the air .
-Cervantes |
|
|
|
|
 |
MihaiG

|
Posted: Mon Dec 20, 2004 1:26 pm Post subject: (No subject) |
|
|
heres the code for boundries.. code: |
if x < 15 then
x := 15
end if
if x > maxx - 15 then
x := maxx - 15
end if
|
put it before the cls
draw circle... :-)otherwise it will be slow |
|
|
|
|
 |
new
|
Posted: Tue Dec 21, 2004 3:54 am Post subject: (No subject) |
|
|
hi guys..thx for your help.....
i 'm adding this jump to ma game mario...in that game...how to make :when he jumps under a block and when he hit it..he sud fall back and not go up any more using wat dot colour..? any ideas??
i'm using this code for the jump part
setscreen ("offscreenonly,graphics:600;350")
var jumping := false
var up : int := 0
const gravity := .15
var value : real
var x, y : real := 33
var chars : array char of boolean
loop
Input.KeyDown (chars)
if chars (KEY_UP_ARROW) and y <= 40 then
up := 1
end if
if up = 1 then
jumping := true
value := 7
end if
if jumping then
value := value - gravity
y := y + value
up := 0
end if
Draw.FillBox (round (x), round (y), round (x + 10), round (y + 10), yellow)
View.Update
end loop |
|
|
|
|
 |
Cervantes

|
|
|
|
 |
|
|