Author |
Message |
Bege
|
Posted: Wed Apr 23, 2003 5:06 pm Post subject: Reading a file: checking to see if a file exists. |
|
|
Does anyone know what the code is to check if a file exists?
Ex: If the user was asked to enter the name of a file to load, then the program could check if the file exists. Then the program wouldn't crash when the user enters a file name which does not exist, but rather just tell the user that that file does not exist. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Mazer

|
Posted: Wed Apr 23, 2003 5:11 pm Post subject: (No subject) |
|
|
File.Exists (pathName : string) : boolean
it's a function that takes a path name for a file and returns true or false depending on whether or not the file exists.
eg
code: |
var filename : string
get filename
if File.Exists (filename) then
put "So, there you go."
end if
|
well, that should do it. |
|
|
|
|
 |
Tony
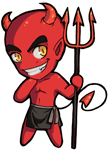
|
Posted: Wed Apr 23, 2003 5:12 pm Post subject: (No subject) |
|
|
well you could use assert, but I think that will terminate the program.
when you open the file, the pointer to it is assigned a possitive integer. If that variable is 0 or a negative, it means file was not opened so you should display an appropriate message.
code: |
var file:int
open : file, "test.txt", get
if file <=0 then
put "file not found"
end if
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Bege
|
Posted: Wed Apr 23, 2003 5:15 pm Post subject: (No subject) |
|
|
Thanks, apreciate it very much. |
|
|
|
|
 |
Mazer

|
Posted: Wed Apr 23, 2003 6:13 pm Post subject: (No subject) |
|
|
yeah, assert is pretty stinking useless, i don't know why people ever used it in programs. |
|
|
|
|
 |
junkpro11
|
Posted: Mon May 24, 2004 10:20 pm Post subject: (No subject) |
|
|
i used File.Exist cuz i found it in the turing reference but when i use it in my program it doesnt detect the file exist
this is my code for file.exists
code: |
var sname:string
loop
get sname
if File.Exists (sname) then
Music.Play ("a")
end if
exit when File.Exists (sname)=true
end loop
|
|
|
|
|
|
 |
s_climax
|
Posted: Tue May 25, 2004 3:59 pm Post subject: (No subject) |
|
|
It works for me, but only if my filename has one word only in the title. |
|
|
|
|
 |
junkpro11
|
Posted: Wed May 26, 2004 4:15 pm Post subject: (No subject) |
|
|
i put the checking of file exists in a loop
and even when the file exist it doesnt exit......so weird
is my code correct though? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
guruguru

|
Posted: Wed May 26, 2004 4:24 pm Post subject: (No subject) |
|
|
Yours worked for me... so did mine 8) . Remember to add the extension (eg. unamed.txt). And make sure its in the same folder me thinks.
code: |
var sname : string
loop
get sname
if File.Exists (sname) then
Music.Play ("a")
exit
end if
end loop
put "Got it!"
|
|
|
|
|
|
 |
beard0

|
Posted: Thu May 27, 2004 10:18 am Post subject: (No subject) |
|
|
s_climax wrote: It works for me, but only if my filename has one word only in the title.
To allow for sname with spaces, must use:
get sname : *
this gets everything up to enter as opposed to everything up to white space. |
|
|
|
|
 |
junkpro11
|
Posted: Fri May 28, 2004 8:36 am Post subject: (No subject) |
|
|
Quote: Yours worked for me... so did mine 8) . Remember to add the extension (eg. unamed.txt). And make sure its in the same folder me thinks.
where do i add the .t extensions? i tried adding in bracket it didnt work...and when i tried (sname)+".t" it doesnt allow me to do that
so if i want to see if (sname) .t file exist wat do i do??? |
|
|
|
|
 |
beard0

|
Posted: Fri May 28, 2004 11:38 am Post subject: (No subject) |
|
|
This should work for you junkpro11:
code: | var sname:string
loop
get sname
if File.Exists (sname) or File.Exists(sname+".t") then
Music.Play ("a")
exit
end if
end loop
|
Note:
the =true on your exit statement is uneccesary. Whenever you hav a condition, it's checking to see if it's true. You don't need to tell it that. Also, your exit condition is the same as your if condition - just put "exit" in your if condition. |
|
|
|
|
 |
junkpro11
|
Posted: Sat May 29, 2004 5:53 pm Post subject: (No subject) |
|
|
my teacher wants onli "exit when" 's in loop, no double exits and no just "exit" |
|
|
|
|
 |
SuperGenius
|
Posted: Sat May 29, 2004 6:04 pm Post subject: (No subject) |
|
|
junkpro11 wrote: my teacher wants onli "exit when" 's in loop, no double exits and no just "exit"
that is stupid, as sometimes you don't need a condition. Some teachers should get bent. |
|
|
|
|
 |
guruguru

|
Posted: Sat May 29, 2004 7:25 pm Post subject: (No subject) |
|
|
Well there is always condition. The exit is always in an if statment. But I agree its stupid .
code: |
var sname:string
loop
get sname
exit when File.Exists (sname) or File.Exists(sname+".t")
end loop
|
Or if you want the sound... it gets worse...
code: |
var sname:string
loop
get sname
if File.Exists (sname) or File.Exists(sname+".t") then
Music.Play ("a")
end if
exit when File.Exists (sname) or File.Exists(sname+".t")
end loop
|
|
|
|
|
|
 |
|