Help with Time Calculator
Author |
Message |
Misha
|
Posted: Fri Oct 01, 2004 12:31 pm Post subject: Help with Time Calculator |
|
|
Hi, I am real noob and I know this is an easy question but I just can't figure this out.
My teacher told us to make a program that will take a number of seconds and convert them into days, hours, minutes, and the remaining seconds so for example 50000 seconds becomes o days, 13 hours, 53 minutes, and 20 seconds (thats the example my teacher gave).
so Far I have this code but I can't figure out how to make it so that it does not convert each thing seperatly e.g. 50000 equalling 0 days, 13 hours, 833 minutes, and 50000 seconds.
Here is my code so far:
code: |
% -------------------------------------------
%
% Date written: Sept 30
%
% Input: number of seconds
% Output: days, hours, minutes
% ---------------------------------------------
% declare variables
var seconds : real
var ans : string
put "please enter a number of seconds"
get seconds
put "minutes ", seconds/60
put "hours ", seconds/60/60
put "days ", seconds/60/60/24
|
any help would be greatly appreciated.
Thanx |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
josh
|
Posted: Fri Oct 01, 2004 12:35 pm Post subject: (No subject) |
|
|
What you need to do is convert all the dyas, hours, adn minutes back into seconds and subtract if from the total. Here is an example.
code: |
var amount : int %The number of seconds the user wishes to convert
var days : int %The number of days that the seconds add up to
var hours : int %The number of hours that the seconds add up to after the days
var minutes : int %The number of minutes that the seconds add up to after the hours
var seconds : int %The remaining amount of seconds
%Get the users input
put "Please input the number of seconds."
get amount
%Calcualte the number of days
days := amount div 86400
%calculate # of hours
hours := (amount - (days * 24 * 60 * 60)) div 3600
%Calcualte # of minutes
minutes := (amount - (hours * 60 * 60)) div 60
%Calculate the # of seconds
seconds := (amount - ((minutes * 60)+ (hours * 60 * 60)))
|
then output the results.
good luck |
|
|
|
|
 |
Paul
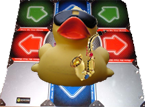
|
Posted: Fri Oct 01, 2004 8:16 pm Post subject: (No subject) |
|
|
the div and mod commands would help u here
code: |
var sec: int
put "Enter number of seconds: "..
get sec
put "this is ", sec div 86400, " days, "..
sec:= sec mod 86400
put sec div 3600, " hours, "..
sec:= sec mod 3600
put sec div 60, " minutes, "..
sec:= sec mod 60
put sec, " seconds."
|
that should work, if it doesn't then I am out of practice |
|
|
|
|
 |
josh
|
Posted: Fri Oct 01, 2004 9:27 pm Post subject: (No subject) |
|
|
Im tired the div and mod commands to but while I was doing itm I thjought if the other way and when I tried it I had less eroros (errors with the Div and Mod were probobly my fault) becuas you aren doing essentially the same thing.
I think I ran into problems with the remainder from dyas cconvertin properly into the hours. |
|
|
|
|
 |
gigaman
|
Posted: Thu Oct 07, 2004 8:13 am Post subject: I'm in this class too |
|
|
as far as the div and mod commands go it is all about the order you put them in in this program. if you put them in the wrong order your program doesn't work. |
|
|
|
|
 |
josh
|
Posted: Thu Oct 07, 2004 8:15 am Post subject: (No subject) |
|
|
exactly, which is why I found it easier to just convert everything back into seconds, but that is to complex for most of our class eh??? |
|
|
|
|
 |
|
|