Reading from a file help???
Author |
Message |
Jonny Tight Lips
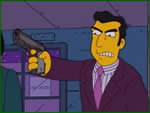
|
Posted: Wed Sep 15, 2004 6:19 pm Post subject: Reading from a file help??? |
|
|
I'm trying to read from a file and get a 2D array from it. IN the file its set up as such:
1 2 3 4 5
6 7 8 9 10
11 12 13 14 15
16 17 18 19 20
and so on and so on. here is the code I have so far:
code: | open : streamin, "map.txt", get
for p : 1 .. 20
for pp : 1 .. 20
get : streamin, maparry (p, pp)..
end for
get : streamin, "\n"
end for
close : streamin |
now I know there are several errors that will come up but I dunno how to fix them. it seems to not like my "\n" or my .. but I dunno whats going on. HELP! PLZ!
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Delos

|
Posted: Wed Sep 15, 2004 6:34 pm Post subject: (No subject) |
|
|
Ok...firstly, never try to extract data from a file and expect it to work smoothly...well, unless you designed the file yourself.
Secondly, extract the raw data alone. I.e., extract the file line by line storing it in a temporary variable (array).
Once that is done, you can then sort through the array, manipulating it as your heart sees fit, and end up with a new 2-D array that you started out to get.
|
|
|
|
|
 |
Jonny Tight Lips
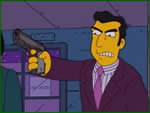
|
Posted: Wed Sep 15, 2004 6:42 pm Post subject: (No subject) |
|
|
now you see that sounds like a great idea. But there is one little problem, I dunno how to do that so if you could maybe explane to me how to do such a thing and maybe give a sample code I would apreciate it greatly.
|
|
|
|
|
 |
Andy
|
Posted: Wed Sep 15, 2004 6:53 pm Post subject: (No subject) |
|
|
hmmm way to complicated...
code: |
open : streamin, "map.txt", get
for p : 1 .. 20
for pp : 1 .. 20
get : streamin, maparry (p, pp)
end for
end for
close : streamin
|
that should do it
|
|
|
|
|
 |
Jonny Tight Lips
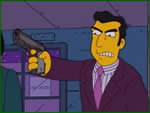
|
Posted: Wed Sep 15, 2004 7:18 pm Post subject: (No subject) |
|
|
tryed that I'm getting an error tho on the get : streamin, maparry (p,pp) line.
it says:
"Invalid interger input"
I dunno whats wrong
|
|
|
|
|
 |
Genesis

|
Posted: Wed Sep 15, 2004 8:50 pm Post subject: (No subject) |
|
|
If you post all your code, with variables and everything, I (or someone else) can probably tell you what's wrong.
|
|
|
|
|
 |
beard0

|
Posted: Thu Sep 16, 2004 7:39 am Post subject: (No subject) |
|
|
This code should be what you're looking for. It's done this way so that you don't have to know how many tokens there are on a line, the program figures that out.
code: | var data : char
var streamin : int
var maparry : array 1 .. 20, 1 .. 20 of string
for i : 1 .. 20
for j : 1 .. 20
maparry (i, j) := ""
end for
end for
var p, pp := 1
open : streamin, "map.txt", get
loop
exit when eof (streamin)
get : streamin, data
if data = " " then
p += 1
elsif data = chr (10) /*The enter character*/ then
pp += 1
p:=1
else
maparry (p, pp) += data
end if
end loop
close : streamin |
Cheers!
|
|
|
|
|
 |
Andy
|
Posted: Thu Sep 16, 2004 3:38 pm Post subject: (No subject) |
|
|
i know ur problem... ur text file is messed, make sure u dont have an extra line of blank at the end and no space after the last number and u do indeed have 400 numbers to input
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Jonny Tight Lips
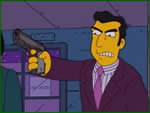
|
Posted: Fri Sep 17, 2004 2:59 pm Post subject: (No subject) |
|
|
I think dodge is right but I dunno how to fix it. I'll attach my map.txt file so you can see since I don't want to type out all 400 numbers. This is my code to make that file.
code: | open : streamout, "map.txt", put
for p : 1 .. 20
for pp : 1 .. 20
put : streamout, maparry (p, pp) ..
end for
if p < 20 then
put : streamout, "\n"
end if
end for
close : streamout |
I dunno why but it seems to be double spaced? I how do I fix that?
Also beard0 I tryed your code it seemed to work but when I tryed to out put the numbers just with 2 for loops and a put statment with the array it went really weird and the numbers flashed by and then were gone like it was inputing some more numbers. Another thing why did you use a string insted of int? They are numbers so shouldn't it be int? How would I get your code to output properly?
Description: |
|
 Download |
Filename: |
map.txt |
Filesize: |
476 Bytes |
Downloaded: |
132 Time(s) |
|
|
|
|
|
 |
Andy
|
Posted: Fri Sep 17, 2004 3:06 pm Post subject: (No subject) |
|
|
why do u need a space between each line???
code: |
open : streamout, "map.txt", put
for p : 1 .. 20
for pp : 1 .. 20
put : streamout, maparry (p, pp)," " ..
end for
put : streamout,""
end for
close : streamout
|
should work with my read code[/code]
|
|
|
|
|
 |
beard0

|
Posted: Fri Sep 17, 2004 3:07 pm Post subject: (No subject) |
|
|
Well, one problem is that you haven't output spaces between your numbers. Do this:
put : streamout, maparry (p, pp)," " ..
Also, your double line is because when you output your newline character, you're not using .. You can do either one of the following:
put : streamout, "\n" ..
-or-
put : streamout, ""
As for my code, since you didn't have spaces between your numbers, It read it all in as one number per line. That means that when you output my numbers, you output all of one line, 19 blank lines...
I used string because you can never asume you know what is in a file. You can later convert it to int using strintok and strint functions (if you don't know them, the reference manual explains them clearly enough)
Hope that helps!
|
|
|
|
|
 |
Jonny Tight Lips
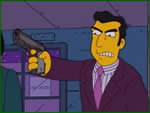
|
Posted: Fri Sep 17, 2004 4:04 pm Post subject: (No subject) |
|
|
That did it. Thanx. needed to spaces between the numbers and not double spaced stuff. You are both a great help!! Thanx
|
|
|
|
|
 |
|
|