Author |
Message |
Blade
|
Posted: Mon Apr 07, 2003 8:15 pm Post subject: Decimal > Binary |
|
|
for school i'm supossed to make a program that converts decimal to binary... and vice versa... could someone suggest a method to do this? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
void

|
Posted: Mon Apr 07, 2003 8:33 pm Post subject: (No subject) |
|
|
i'd use a loop to check to see what the largest power of two is that can go into the enterd number....(like if num_entered <2**a(where a is now 5) then largest_power:=a-1..end if and exit loop) and then its simple to just chek, display a 1 or 0 and subtract....as for the binary to decimal...well...try loading the number as a string...then doing a check on it...find out how many numbers are in it...thats the largest power of two to multiply by...then you use a for a:1..lenght(binary_number) and check for what place all the ones are in and while your doing that....every time there is a one..then decimal_number:=decimal_number+2**a other wise do the hokey pokey ) i hope that helps you some what.. |
|
|
|
|
 |
Dan
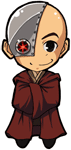
|
Posted: Mon Apr 07, 2003 11:48 pm Post subject: (No subject) |
|
|
DAN'S CHEP WAY OF BASE COVERSION
code: |
var num: int
put "put in a num: "..
get num
put ""
put intstr(num,0,2)
|
the intstr comand can cahge the base of the int being sent in to it. in thiis case it chages it to a base of 2 or binaey.
this method whode not be good for a school progject becuse it is not grat progaming and techers dont like it becuse it dose not giive them a lot to mark
for more help on intstr look it up in help in turing... |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
Asok

|
Posted: Mon Apr 07, 2003 11:54 pm Post subject: (No subject) |
|
|
dan you owned that assignment!  |
|
|
|
|
 |
Prince
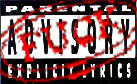
|
Posted: Tue Apr 08, 2003 9:52 am Post subject: (No subject) |
|
|
heres how i did it
code: |
function getBinary : int
var bin_num : int
loop
cls
put "Enter a number in binary form -> " ..
get bin_num
exit when bin_num >= 0
end loop
result bin_num
end getBinary
% ---------- Main ----------
var binary, decimal, digit, temp, x, sum, ibinary : int
sum := 0
x := 1
binary := getBinary
ibinary := binary
loop
digit := binary mod 10
binary := binary div 10
temp := digit * x
x := x * 2
sum := sum + temp
exit when binary = 0
end loop
put ""
put "The decimal equivalent of ", ibinary, " is ", sum
|
complete with a function (impressive isnt it.. lol) |
|
|
|
|
 |
Blade
|
Posted: Tue Apr 08, 2003 11:00 am Post subject: (No subject) |
|
|
lol, yea... thx... whats the loop for in the function? |
|
|
|
|
 |
Asok

|
Posted: Tue Apr 08, 2003 11:01 am Post subject: (No subject) |
|
|
because it handles it one digit at a time so it needs to loop it |
|
|
|
|
 |
Prince
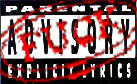
|
Posted: Tue Apr 08, 2003 5:32 pm Post subject: (No subject) |
|
|
well actually the one in the function jus makes sure that no idiots run it a start typin away negative numbers (tho i now realize there r idiots who'll enter non-binary numbers )... but good job on explainin the second loop asok |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Asok

|
Posted: Tue Apr 08, 2003 11:59 pm Post subject: (No subject) |
|
|
ohh... the loop IN the function. meh, I'm tired. |
|
|
|
|
 |
Blade
|
Posted: Wed Apr 09, 2003 12:16 am Post subject: (No subject) |
|
|
i honestly didnt think there would be anyone stupid enough to try to do that.... (enter negitive numbers)... but anotheer way to handle it would be to use natural variables... |
|
|
|
|
 |
Asok

|
Posted: Wed Apr 09, 2003 12:50 am Post subject: (No subject) |
|
|
well technically -101 is still the binary equivalent of -5 so you can get negative numbers in binary |
|
|
|
|
 |
|