Small Engine
Author |
Message |
Brewah
|
Posted: Mon Apr 07, 2003 10:12 pm Post subject: Small Engine |
|
|
Not too good at explaining but here I go....
Okay, I'm working on a flying/shooting game or whatever you want to call it, for it I'm working on a basic engine that i can use for different bitmap levels and etc, anyways the problem is with bullets. I can get it to fly around and shoot, but if you shoot, then turn around the bullet swtiches over the pixels on the side that you just flipped over too.
Heres the code...
code: |
%Vetox Engine
%Mucho help from spacegame, and COMPSCI
%Various Constants
const jet_radius := 26 %Your character's radius
const missle_radius := 3 %Bullet radius
const margin_move : int := 140
const margin : int := margin_move + 10
%Various Variables
var keys : array char of boolean
var jetX : int := 300 %Start point for your character
var jetY : int := 300 %Start point for your character
var jet : int
var jet_left : int
var jet_left_boolean : boolean := false
var level : int
var levelX : int := 0 %Where you want BG to be placed
var levelY : int := 0 %Where you want BG to be placed
var jet_missle : int
var jet_missle_left : int
var jet_missleX : int := 5 %Temp. pos for missle (won't show up, must be 5 for code to flow proper)
var jet_missleY : int := 5 %Temp. pos for missle (won't show up, must be 5 for code to flow proper)
var jet_missleX_left : int := 5 %Temp. pos for missle (won't show up, must be 5 for code to flow proper)
var jet_missleY_left : int := 5 %Temp. pos for missle (won't show up, must be 5 for code to flow proper)
var jet_missle_fire : boolean := false %Check to see if you have hit fire button
var jet_missle_fire_left : boolean := false %Check to see if you have hit fire button
var jet_missle_fire_loc : boolean := false %Check to see if you have hit fire button and locate where you are
%Image declares
jet := Pic.FileNew ("images/jet.BMP")
Pic.SetTransparentColour (jet, white)
jet_left := Pic.FileNew ("images/jet_left.BMP")
Pic.SetTransparentColour (jet_left, white)
level := Pic.FileNew ("images/level.JPG")
jet_missle := Pic.FileNew ("images/missle.BMP")
Pic.SetTransparentColour (jet_missle, white)
jet_missle_left := Pic.FileNew ("images/missle_left.BMP")
Pic.SetTransparentColour (jet_missle_left, white)
%Height/width of select images
var jet_width := Pic.Width (jet) %Gets the width of your character
var jet_height := Pic.Height (jet) %Geths the height of your character
var jet_left_width := Pic.Width (jet) %Gets the width of your character (left)
var jet_left_height := Pic.Height (jet) %Geths the height of your character (left)
var level_width := Pic.Width (level) %Gets the width of the level
var level_height := Pic.Height (level) %Gets the height of the level
%Initial Setscreen
setscreen ("graphics:800;550,position:middle;centre,nobuttonbar,title:Vetox Engine")
loop
%Sets screen for animation
setscreen ("offscreenonly")
%Key Input code, do what you want according to key
Input.KeyDown (keys)
if keys (KEY_LEFT_ARROW)
then
jetX -= 4
jet_left_boolean := true
elsif keys (KEY_RIGHT_ARROW)
then
jetX += 4
jet_left_boolean := false
end if
if keys (KEY_UP_ARROW)
then
jetY += 3
elsif keys (KEY_DOWN_ARROW)
then
jetY -= 3
end if
if keys (KEY_CTRL)
then
%Fire
if jet_missle_fire = false %Makes sure location of jet and bullet is set only once
then
jet_missle_fire_loc := true
end if
jet_missle_fire := true
jet_missle_fire_left := true
end if
%Restricts the character movement horizontally (so you can't go past background)
if jetX < margin
then
jetX := margin
end if
if jetX > level_width - margin
then
jetX := level_width - margin
end if
%Restricts the character movement vertically (so you can't go past background)
if jetY < margin
then
jetY := margin
end if
if jetY > level_height - margin
then
jetY := level_height - margin
end if
%Move the background according to where you are
if jetX - levelX < margin_move
then
levelX := round (jetX - margin_move)
end if
if levelX + maxx + 1 - jetX < margin_move
then
levelX := round (jetX + margin_move - (maxx + 1))
end if
if jetY - levelY < margin_move
then
levelY := round (jetY - margin_move)
end if
if levelY + maxy + 1 - jetY < margin_move
then
levelY := round (jetY + margin_move - (maxy + 1))
end if
%Set up character's missle location, crtl must be fired, so it gains your location only once
if jet_missle_fire_loc = true
then
jet_missleX := round (jetX) - levelX - jet_radius + 23
jet_missleY := round (jetY) - levelY - jet_radius + 2
jet_missleX_left := round (jetX) - levelX - jet_radius + 23
jet_missleY_left := round (jetY) - levelY - jet_radius + 2
jet_missle_fire_loc := false
end if
%Draw commands
Pic.Draw (level, -levelX, -levelY, picCopy) %Draw background
%If going left is false then draw this picture
if jet_left_boolean = false
then
%Draws character according to jetX, jetY
Pic.Draw (jet,
round (jetX) - levelX - jet_radius,
round (jetY) - levelY - jet_radius, picMerge)
%If missle is fired than draw it
if jet_missle_fire = true
then
jet_missleX += 8 %How much distance the missle gains each turn
Pic.Draw (jet_missle, jet_missleX, jet_missleY, picMerge)
%If character's missle goes outside of window, it disappears, so you can fire another missle
if jet_missleX > maxx
then
jet_missle_fire_left := false
jet_missle_fire := false
end if
end if
end if
%Left is true, therefore draws this picture
if jet_left_boolean = true
then
%Draws character according to jetX, jetY
Pic.Draw (jet_left,
round (jetX) - levelX - jet_radius,
round (jetY) - levelY - jet_radius, picMerge)
%If missle is fired than draw it
if jet_missle_fire_left = true
then
jet_missleX_left -= 8 %How much distance the missle gains each turn
Pic.Draw (jet_missle_left, jet_missleX_left, jet_missleY_left, picMerge)
%If character's missle goes outside of window, it disappears, so you can fire another missle
if jet_missleX_left < 0
then
jet_missle_fire_left := false
jet_missle_fire := false
end if
end if
end if
View.Update
end loop
|
Any ideas? (Optimization ideas wouldn't hurt either.) |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Dan
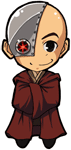
|
Posted: Mon Apr 07, 2003 11:40 pm Post subject: (No subject) |
|
|
your fomating and coding styel was a litte hrad for me to check on to at first but i got it wroking. your problem was that you where not checking wich way the plane was facing when the butle was shot but you where checking it constey. wich gave you the wired bulte thing. by adding an exrate veribale to keep trace of the direction of the palne when the bulte is fired and by moving some if's arowned and adding some to check the direction when fired it will wrok.
i made the modifactions to the code for you below. note that i chaged the loaction of the picher files so it whode wrok on my computer. just chage them back befor you run it.
code: |
%Vetox Engine
%Mucho help from spacegame, and COMPSCI, and Dan ;)
%Various Constants
const jet_radius := 26 %Your character's radius
const missle_radius := 3 %Bullet radius
const margin_move : int := 140
const margin : int := margin_move + 10
%Various Variables
var keys : array char of boolean
var jetX : int := 300 %Start point for your character
var jetY : int := 300 %Start point for your character
var jet : int
var jet_left : int
var jet_left_boolean : boolean := false
var level : int
var levelX : int := 0 %Where you want BG to be placed
var levelY : int := 0 %Where you want BG to be placed
var jet_missle : int
var jet_missle_left : int
var jet_missleX : int := 5 %Temp. pos for missle (won't show up, must be 5 for code to flow proper)
var jet_missleY : int := 5 %Temp. pos for missle (won't show up, must be 5 for code to flow proper)
var jet_missleX_left : int := 5 %Temp. pos for missle (won't show up, must be 5 for code to flow proper)
var jet_missleY_left : int := 5 %Temp. pos for missle (won't show up, must be 5 for code to flow proper)
var jet_missle_fire : boolean := false %Check to see if you have hit fire button
var jet_missle_fire_left : boolean := false %Check to see if you have hit fire button
var jet_missle_fire_loc : boolean := false %Check to see if you have hit fire button and locate where you are
var fire_left : boolean := false
%Image declares
jet := Pic.FileNew ("jet.BMP")
Pic.SetTransparentColour (jet, white)
jet_left := Pic.FileNew ("jet_left.BMP")
Pic.SetTransparentColour (jet_left, white)
level := Pic.FileNew ("level.JPG")
jet_missle := Pic.FileNew ("missle.BMP")
Pic.SetTransparentColour (jet_missle, white)
jet_missle_left := Pic.FileNew ("missle_left.BMP")
Pic.SetTransparentColour (jet_missle_left, white)
%Height/width of select images
var jet_width := Pic.Width (jet) %Gets the width of your character
var jet_height := Pic.Height (jet) %Geths the height of your character
var jet_left_width := Pic.Width (jet) %Gets the width of your character (left)
var jet_left_height := Pic.Height (jet) %Geths the height of your character (left)
var level_width := Pic.Width (level) %Gets the width of the level
var level_height := Pic.Height (level) %Gets the height of the level
%Initial Setscreen
setscreen ("graphics:800;550,position:middle;centre,nobuttonbar,title:Vetox Engine")
loop
%Sets screen for animation
setscreen ("offscreenonly")
%Key Input code, do what you want according to key
Input.KeyDown (keys)
if keys (KEY_LEFT_ARROW)
then
jetX -= 4
jet_left_boolean := true
elsif keys (KEY_RIGHT_ARROW)
then
jetX += 4
jet_left_boolean := false
end if
if keys (KEY_UP_ARROW)
then
jetY += 3
elsif keys (KEY_DOWN_ARROW)
then
jetY -= 3
end if
if keys (KEY_CTRL)
then
%Fire
if jet_missle_fire = false %Makes sure location of jet and bullet is set only once
then
jet_missle_fire_loc := true
end if
jet_missle_fire := true
jet_missle_fire_left := true
if jet_left_boolean = false then
fire_left := false
else
fire_left := true
end if
end if
%Restricts the character movement horizontally (so you can't go past background)
if jetX < margin
then
jetX := margin
end if
if jetX > level_width - margin
then
jetX := level_width - margin
end if
%Restricts the character movement vertically (so you can't go past background)
if jetY < margin
then
jetY := margin
end if
if jetY > level_height - margin
then
jetY := level_height - margin
end if
%Move the background according to where you are
if jetX - levelX < margin_move
then
levelX := round (jetX - margin_move)
end if
if levelX + maxx + 1 - jetX < margin_move
then
levelX := round (jetX + margin_move - (maxx + 1))
end if
if jetY - levelY < margin_move
then
levelY := round (jetY - margin_move)
end if
if levelY + maxy + 1 - jetY < margin_move
then
levelY := round (jetY + margin_move - (maxy + 1))
end if
%Set up character's missle location, crtl must be fired, so it gains your location only once
if jet_missle_fire_loc = true
then
jet_missleX := round (jetX) - levelX - jet_radius + 23
jet_missleY := round (jetY) - levelY - jet_radius + 2
jet_missleX_left := round (jetX) - levelX - jet_radius + 23
jet_missleY_left := round (jetY) - levelY - jet_radius + 2
jet_missle_fire_loc := false
end if
%Draw commands
Pic.Draw (level, -levelX, -levelY, picCopy) %Draw background
%If going left is false then draw this picture
if jet_left_boolean = false
then
%Draws character according to jetX, jetY
Pic.Draw (jet,
round (jetX) - levelX - jet_radius,
round (jetY) - levelY - jet_radius, picMerge)
%If missle is fired than draw it
elsif jet_left_boolean = true
then
%Draws character according to jetX, jetY
Pic.Draw (jet_left,
round (jetX) - levelX - jet_radius,
round (jetY) - levelY - jet_radius, picMerge)
end if
if jet_missle_fire = true
then
if fire_left = false then
jet_missleX += 8 %How much distance the missle gains each turn
Pic.Draw (jet_missle, jet_missleX, jet_missleY, picMerge)
%If character's missle goes outside of window, it disappears, so you can fire another missle
if jet_missleX > maxx
then
jet_missle_fire_left := false
jet_missle_fire := false
end if
elsif fire_left = true then
jet_missleX_left -= 8 %How much distance the missle gains each turn
Pic.Draw (jet_missle_left, jet_missleX_left, jet_missleY_left, picMerge)
%If character's missle goes outside of window, it disappears, so you can fire another missle
if jet_missleX_left < 0
then
jet_missle_fire_left := false
jet_missle_fire := false
end if
end if
end if
View.Update
end loop
|
|
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
Prince
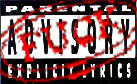
|
Posted: Tue Apr 08, 2003 9:55 am Post subject: (No subject) |
|
|
err... can sumbody explain y winoot 3.1.1 is so lacking that i cant run half these great programs (especially ones with View.Update)  |
|
|
|
|
 |
Asok

|
Posted: Tue Apr 08, 2003 10:27 am Post subject: (No subject) |
|
|
because winoot 3.1.1 is OLD lol, if you want to see programs that use NEW commands you need to get the NEW winoot version. |
|
|
|
|
 |
Prince
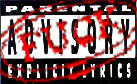
|
Posted: Tue Apr 08, 2003 10:28 am Post subject: (No subject) |
|
|
wher can i get winoot 4 without having to pay? or without askin my skool to get licensing (stupid skool) |
|
|
|
|
 |
Asok

|
Posted: Tue Apr 08, 2003 10:34 am Post subject: (No subject) |
|
|
we get that question a lot, and frankly, it'd be illegal for us to tell you so I'll keep my mouth shut on that one.  |
|
|
|
|
 |
Brewah
|
Posted: Tue Apr 08, 2003 5:53 pm Post subject: (No subject) |
|
|
Thanks for the help Dan. |
|
|
|
|
 |
|
|