How to get the direction (in degrees) between two points?
Author |
Message |
s_climax
|
Posted: Tue May 04, 2004 5:32 pm Post subject: How to get the direction (in degrees) between two points? |
|
|
ssia |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Mazer

|
Posted: Tue May 04, 2004 6:09 pm Post subject: Re: How to get the direction (in degrees) between two points |
|
|
s_climax wrote: ssia
What the hell?
Anyways...
you can use arctand to give you an angle in degrees by supplying it with a slope. Of course that's not enough (most likely); that will just give you something between -90 and 90. You'll need to figure out the actual angle by checking which quadrant the second point is in with relation to the first point. |
|
|
|
|
 |
s_climax
|
Posted: Tue May 04, 2004 6:18 pm Post subject: (No subject) |
|
|
ssia stand for "subject says it all"
Isn't there just some code I can use with sin/cos? |
|
|
|
|
 |
Mazer

|
Posted: Tue May 04, 2004 6:30 pm Post subject: (No subject) |
|
|
Why would you want that?
You wanted a way to get an angle between two points. Given that you have points A (x1, y1) and B (x2, y2) you can get the angle from A to B using arctand (a.k.a. "tan inverse").
code: |
angle := arctand ((y2 - y1) / (x2 - x1))
|
Angle, in this case should be a float. This is also assuming that B is above and to the right of A on a cartesian plane. If it's to the left you'll get a negative angle. And of course make sure to check if the x values are equal so you don't get a division by zero error. |
|
|
|
|
 |
Cervantes

|
Posted: Tue May 04, 2004 7:29 pm Post subject: (No subject) |
|
|
code: |
function find_angle (x1, y1, x2, y2 : real) : real
if x2 = x1 then
if y2 >= y1 then
result 90
elsif y2 < y1 then
result 270
end if
else
if x2 > x1 and y2 >= y1 then %QUAD 1
result (arctand ((y2 - y1) / (x2 - x1)))
elsif x2 > x1 and y2 < y1 then %QUAD 2
result 360 + (arctand ((y2 - y1) / (x2 - x1)))
elsif x2 < x1 and y2 < y1 then %QUAD 3
result 180 + (arctand ((y2 - y1) / (x2 - x1)))
elsif x2 < x1 and y2 >= y1 then %QUAD 4
result 180 + (arctand ((y2 - y1) / (x2 - x1)))
end if
end if
end find_angle
Mouse.ButtonChoose ("multibutton")
var x1, y1, x2, y2 : real
var mx, my, btn : int
var firsthit := false
loop
cls
mousewhere (mx, my, btn)
if btn = 1 and firsthit = false then
firsthit := true
x1 := mx
y1 := my
end if
if btn = 100 then
firsthit := false
end if
if firsthit = true then
x2 := mx
y2 := my
drawline (round (x1), round (y1), round (x2), round (y2), black)
locate (1, 1)
put find_angle (x1, y1, x2, y2)
end if
View.Update
delay (10)
end loop
|
that's how.  |
|
|
|
|
 |
s_climax
|
Posted: Tue May 04, 2004 8:24 pm Post subject: (No subject) |
|
|
If the line is horizontal and going to the right, shouldn't the angle be 360 or 0 not 270? |
|
|
|
|
 |
Cervantes

|
Posted: Thu May 06, 2004 4:48 pm Post subject: (No subject) |
|
|
no it shouldn't be.
and in this program and in normal cardinal directions, a horizontal line going to the right isn't 270, its 90. |
|
|
|
|
 |
Tony
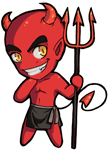
|
Posted: Thu May 06, 2004 4:53 pm Post subject: (No subject) |
|
|
umm... no...
horizontal line that is directly to the right is 0
up is 90
left is 180
down is 270
do you know why? because cos(0) = 1 |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
s_climax
|
Posted: Thu May 06, 2004 5:16 pm Post subject: (No subject) |
|
|
Thanks for clarifying that tony. I was right. |
|
|
|
|
 |
Cervantes

|
Posted: Thu May 06, 2004 5:19 pm Post subject: (No subject) |
|
|
Cervantes wrote: cardinal directions
anywho, a line going to the right still wasn't 270. |
|
|
|
|
 |
s_climax
|
Posted: Fri May 14, 2004 8:34 pm Post subject: (No subject) |
|
|
Will this work instead?
code: |
xDistance := personX - chaserX
yDistance := personY - chaserY
angle := tan (yDistance / xDistance)
|
|
|
|
|
|
 |
Tony
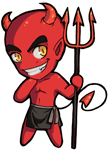
|
Posted: Fri May 14, 2004 9:45 pm Post subject: (No subject) |
|
|
no, lol... first of all if xDistance = 0 you get runtime error (div 0) and even if you don't, it's arctan, not tan... that returns the ratio based on the angle (in radians) |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
guruguru

|
Posted: Fri May 14, 2004 11:14 pm Post subject: (No subject) |
|
|
My example was simply that, and example. Clearly there are errors that can occur (dividing by zero), but they can be tested for and then dealt with.
Sorry, I thought that Turing did it in degrees... my bad. Anyways, to convert to degrees, simple multiply the answer by 180/Pi.
As well, I realize that it works for only one quadrant. Again, this was an EXAMPLE, so you can easily modify it!!! |
|
|
|
|
 |
|
|