Grid detection in 2D Platformer
Author |
Message |
haayyeess
|
Posted: Sun Dec 13, 2015 9:04 am Post subject: Grid detection in 2D Platformer |
|
|
Hi guys,
I'm creating a 2D platformer game for a computer science project and I have ran into some difficulties and require support. I have a movement procedure that needs to know if the users movement will be ok through checking in a detection process, and if not the controls won't be allowed. I'm using a grid system for the checking, which works ok in the x direction where the user can only move one grid space at a time. In the y direction, when I allow the user to jump, sure the initial jump might be ok, and it must be for it to happen, but the path followed afterwards is not necessarily OK. is there a way I can check the users x and y coordinates constantly within detection and prevent movement if either is going to enter a grid where it shouldn't?
Thanks for the support guys.
I need to create a flow diagram for user controls, and understanding of this is crucial.
Also, can someone explain what the full code for a statement like this is? What does the '-=' represent? How should this be represented in a flow diagram? Thanks.
"PlayerYDirectionVelocity -= GRAVITY"
I have attached a picture of my rough Flowchart Algorithm for reference.
Description: |
|
Filesize: |
1.14 MB |
Viewed: |
2767 Time(s) |

|
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Jeffmagma
|
Posted: Sun Dec 13, 2015 9:36 am Post subject: RE:Grid detection in 2D Platformer |
|
|
PlayerYDirectionVelocity -= GRAVITY means set PlayerYDirectionVelocity to PlayerYDirectionVelocity - GRAVITY
Basivally: PlayerYDirectionVelocity :=PlayerYDirectionVelocity - GRAVITY
|
|
|
|
|
 |
haayyeess
|
Posted: Sun Dec 13, 2015 3:22 pm Post subject: Re: RE:Grid detection in 2D Platformer |
|
|
Jeffmagma @ Sun Dec 13, 2015 9:36 am wrote: PlayerYDirectionVelocity -= GRAVITY means set PlayerYDirectionVelocity to PlayerYDirectionVelocity - GRAVITY
Basivally: PlayerYDirectionVelocity :=PlayerYDirectionVelocity - GRAVITY
Ahh right. Thank you!
|
|
|
|
|
 |
haayyeess
|
Posted: Sun Dec 13, 2015 3:41 pm Post subject: Re: Grid detection in 2D Platformer |
|
|
I have updated the flow diagram...
Description: |
|
Filesize: |
1.1 MB |
Viewed: |
2744 Time(s) |

|
|
|
|
|
|
 |
Tony
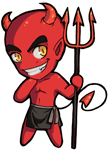
|
Posted: Sun Dec 13, 2015 4:38 pm Post subject: RE:Grid detection in 2D Platformer |
|
|
I imagine that your challenge might have to do with fast moving objects. We have a tutorial that covers the complexities of this at http://compsci.ca/v3/viewtopic.php?t=14897 but if you are using grids, then your specific case is much simpler.
In short, if the object is falling at velocity that's greater than 1 grid per frame, then it's not enough to check the end position, as grids in the middle will be missed, and object has chance to fall through the floor.
The simplest would be to calculate the line between start and end, and then check every grid that is crossed. In most cases that would happen to be just one grid anyway. The beautiful part is that the same code will be able to handle distances of 1, 2, and more grids.
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
haayyeess
|
Posted: Sun Dec 13, 2015 8:53 pm Post subject: Re: Grid detection in 2D Platformer |
|
|
Here is a more legible version of the flowchart algorithm... See any issues?
Description: |
|
Filesize: |
1.3 MB |
Viewed: |
2738 Time(s) |

|
|
|
|
|
|
 |
haayyeess
|
Posted: Sun Dec 13, 2015 9:13 pm Post subject: Re: RE:Grid detection in 2D Platformer |
|
|
Tony @ Sun Dec 13, 2015 4:38 pm wrote: I imagine that your challenge might have to do with fast moving objects. We have a tutorial that covers the complexities of this at http://compsci.ca/v3/viewtopic.php?t=14897 but if you are using grids, then your specific case is much simpler.
In short, if the object is falling at velocity that's greater than 1 grid per frame, then it's not enough to check the end position, as grids in the middle will be missed, and object has chance to fall through the floor.
The simplest would be to calculate the line between start and end, and then check every grid that is crossed. In most cases that would happen to be just one grid anyway. The beautiful part is that the same code will be able to handle distances of 1, 2, and more grids.
Circle detection would be a completely different style of detection and at this point, although I wish I would have used it, I must stay with the grid system.
I'm not sure what the frame rate will workout to be, the movement is user controlled, but jumping is where it would excel.
If i'm checking every grid crossed based on an equation, wouldn't this prevent their jump before it started, Tony?
How do I incorporate this into user controls in order to prevent the invalid movement while in air? Here's what I thought...
I added resulting flags for the three possible travel directions, back, forward, and upwards, rather than just one.
While in air, if detection is constantly checking the next grid space the user COULD enter (they can change direction in air currently, could remove this i suppose) and results a Boolean flag only allowing movement in the direction if the flag is true (movement is OK).
Only problem is they can still fall through objects, including ground.
I'll look into this more tomorrow.
|
|
|
|
|
 |
Tony
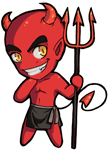
|
Posted: Sun Dec 13, 2015 9:31 pm Post subject: RE:Grid detection in 2D Platformer |
|
|
When I talk about frames, I'm talking about each time the game screen is refreshed to draw the current state of the game world. If an object's movement is updated as
code: |
position_x := position_x + velocity_x
|
then the implication is that the velocity is measured in units per frame (frame now becomes a unit of time).
Lets imagine that an object is traveling with a speed of 4 grid cells per frame. For the time to pass, and object to update its position from current frame to the following frame, you could plot out its path:
code: |
[A] -> [ ] -> [ ] -> [X] -> [ ]
|
Where "A" would be the start position, and "X" would be some obstacle.
If we look just at the end position, then the object will "teleport" over the wall. So each grid must be examined along the path. Then we could move as far as we can, along this path, until an obstacle is encountered (or we get to the end of the path).
code: |
[ ] -> [ ] -> [A] -> [X] -> [ ]
|
Something like a for-loop could be used to loop over a number of cells along the path, but you could also implement that detail in different ways.
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
|
|