Program is stuck at "Executing."
Author |
Message |
gayden
|
Posted: Mon Mar 02, 2015 10:36 am Post subject: Program is stuck at "Executing." |
|
|
Not sure if the purpose of the program is really relevant or not, but basically it's meant to complete Shawnar's Process, which dictates: "Given any positive number, you can perform the following operations on it:
-If even, divide by two
-If odd, multiply by three and then add one
and eventually you will reach the number one.
Example: 13
13 * 3 + 1 = 40
40 / 2 = 20
20 / 2 = 10
10 / 2 = 5
5 * 3 + 1 = 16
16 / 2 = 8
8 / 2 = 4
4 / 2 = 2
2 / 2 = 1
Anyway, when I run the program it sort of pauses at line 13. The Turing window says "Executing." I am given no error.
code: | var font1 := Font.New ("Times:12")
Font.Draw ("Shawnar's Process", 230, 380, font1, red)
var posnum, xnum : real
var num, counter : int
var ans : string (1)
counter := 0
locate (3, 5)
put "How many numbers do you want to test? " ..
get num
for i : 1 .. num
put "Enter the positive number for test #", i, ": " ..
get posnum
if (posnum <= 0) then
put "Enter the positive number for test #", i, ": " ..
get posnum
else
loop
if (posnum mod 2 = 0) then
xnum := posnum / 2
else
xnum := (posnum * 3) + 1
end if
exit when xnum = 1
counter := counter + 1
end loop
end if
put "It took ", counter, " iterations for ", posnum, " to reach a 1."
end for
|
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Insectoid

|
Posted: Mon Mar 02, 2015 11:23 am Post subject: RE:Program is stuck at "Executing." |
|
|
code: | loop
if (posnum mod 2 = 0) then
xnum := posnum / 2
else
xnum := (posnum * 3) + 1
end if
exit when xnum = 1
counter := counter + 1
end loop |
This loop never exits under some condition. This is because posnum never changes, so unless xnum = 1 is satisfied after the first iteration of the loop, it will never exit. |
|
|
|
|
 |
gayden
|
Posted: Wed Mar 04, 2015 10:34 am Post subject: Re: RE:Program is stuck at "Executing." |
|
|
Insectoid @ Mon Mar 02, 2015 11:23 am wrote: This loop never exits under some condition. This is because posnum never changes, so unless xnum = 1 is satisfied after the first iteration of the loop, it will never exit.
So if I change every isntance of 'xnum' in the program to 'posnum,' so that posnum does now change, it should work right?
edit: Now I run into more problems. I shall update when I figure it out.
edit2: Program now looks like:
code: | var font1 := Font.New ("Times:12")
Font.Draw ("Shawnar's Process", 230, 380, font1, red)
var posnum, originalnumber : real
var num, counter : int
locate (3, 1)
put "How many numbers do you want to test? " ..
get num
for i : 1 .. num
put "Enter the positive number for test #", i, ": " ..
get posnum
counter := 1
originalnumber := posnum
loop
if (posnum mod 2 = 0) then
posnum := posnum / 2
else
posnum := (posnum * 3) + 1
end if
exit when posnum = 1
counter := counter + 1
end loop
put "It took ", counter, " iterations for ", originalnumber, " to reach a 1."
end for
%TO DO
%Add negative fail-safe for get posnum
%Add 100 iteration continuation thing |
|
|
|
|
|
 |
Clayton

|
Posted: Thu Mar 05, 2015 3:43 pm Post subject: RE:Program is stuck at "Executing." |
|
|
What problems are you running into exactly? Not being able to test your code at the moment, so I might be missing something small, but at a glance, this appears like it should do what you're looking to do. |
|
|
|
|
 |
Tony
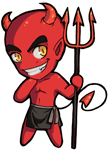
|
Posted: Thu Mar 05, 2015 4:07 pm Post subject: RE:Program is stuck at "Executing." |
|
|
Quote:
exit when posnum = 1
Does 1.0 equal to 1? Sometimes, but not always. real (known as float in other languages) are designed to for less-than and greater-than comparisons, not for equalness. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
gayden
|
Posted: Fri Mar 06, 2015 10:07 am Post subject: Re: RE:Program is stuck at "Executing." |
|
|
Tony @ Thu Mar 05, 2015 4:07 pm wrote: Quote:
exit when posnum = 1
Does 1.0 equal to 1? Sometimes, but not always. real (known as float in other languages) are designed to for less-than and greater-than comparisons, not for equalness.
I'm not even sure why posnum and originalnumber were declared as real numbers as opposed to integers. My mistake
Clayton @ Thu Mar 05, 2015 3:43 pm wrote: What problems are you running into exactly? Not being able to test your code at the moment, so I might be missing something small, but at a glance, this appears like it should do what you're looking to do.
I fixed it now.
Although now I have a question with the same program.
"For every one hundred iterations the program should ask the user if they want to continue."
Is there a way I can automate this, or do I have to do a separate if statement for multiple multiples (heh) of 100 |
|
|
|
|
 |
Dreadnought
|
Posted: Fri Mar 06, 2015 10:47 am Post subject: Re: Program is stuck at "Executing." |
|
|
There is a way you can automate this. rem and mod may be helpful, but are not necessary to accomplish what you are trying to do. |
|
|
|
|
 |
|
|