Author |
Message |
JR
|
Posted: Thu Feb 26, 2004 9:01 am Post subject: Help finding the median number in a program |
|
|
ok, this is my program and i need to find the median(middle) of the numbers. heres my code:
code: | var count : int
var num : int
put "How many integers are in the list? " ..
get count
var list : array 1 .. count of int
for i : 1 .. count
get list (i)
end for
var sortList : array 1 .. count of int
for i : 1 .. count
var smallest := 999
var where : int
for j : 1 .. count
if list (j) < smallest then
smallest := list (j)
where := j
end if
end for
sortList (i) := smallest
list (where) := 999
end for
for i : 1 .. count
put sortList (i) ..
put " ; " ..
end for
|
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
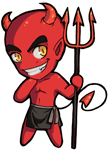
|
Posted: Thu Feb 26, 2004 2:03 pm Post subject: (No subject) |
|
|
what you do is you sort your array in decending/accending order (doesn't matter... should be same answer) using something like a bubble sort for example...
then just pick the number in the middle of the array by dividing it's upper limit into half.
code: |
put myArray(round(upper(myArray)/2))
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
nis
|
Posted: Thu Feb 26, 2004 5:16 pm Post subject: (No subject) |
|
|
to sort your list use this code
code: |
for decreasing i : count - 1 .. 1
for j : 1 .. i
if list(j) < list(j+1) then
temp := list(j)
list(j) := list(j+1)
list(j+1) := temp
end if
end for
end for
|
then just add the code
code: |
put "The median is ", list(count div 2)
|
|
|
|
|
|
 |
Cervantes

|
Posted: Thu Feb 26, 2004 5:27 pm Post subject: (No subject) |
|
|
he's already got the sorting part. It's in his post...
Tony, what's a bubble sort? Is it what he has there? I haven't learned the ways of sorting in class yet, only on my own. |
|
|
|
|
 |
JR
|
Posted: Thu Feb 26, 2004 5:43 pm Post subject: (No subject) |
|
|
got it to work, thx alot |
|
|
|
|
 |
Tony
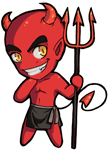
|
Posted: Thu Feb 26, 2004 8:52 pm Post subject: (No subject) |
|
|
Cervantes - yeah, that's whats posted up... you check variables with each other and if one is larger then the other, you switch them |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
|