Can you make a Roguelike in turing?
Author |
Message |
Sicarius
|
Posted: Sun Dec 08, 2013 3:28 pm Post subject: Can you make a Roguelike in turing? |
|
|
Hello everyone, I'm in a computer science class right now and for our FSE we have to make a game, (pretty sure its like that for everyone) I'm a pretty big fan of roguelikes so me and my ultimate wisdom decided that i would like to make one for my FSE. Unfortunately i haven't got the slightest clue if this is a reasonable type of game to make in Turing now do i have the slightest clue where to start. I know I'm going to need some kinda of grid that i can make and destroy to randomly generate a map but after that I'm clueless.
I know you cant just give me whole codes or anything, but if there is someone who has done a rougelike before could you give me some help?
I'm using Turing 4.1.1 by the way |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Insectoid

|
Posted: Sun Dec 08, 2013 3:37 pm Post subject: RE:Can you make a Roguelike in turing? |
|
|
You can absolutely do it. It's just gonna take a whole lot of work. Break the game down into basic mechanics and work on making each of those mechanics work. Presumably, your character can move. Make that work first. Then work on the rest of the game. |
|
|
|
|
 |
Tony
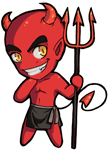
|
|
|
|
 |
Sicarius
|
Posted: Sun Dec 08, 2013 4:56 pm Post subject: RE:Can you make a Roguelike in turing? |
|
|
for this rogue like I'm not really going for aesthetics, so I'm going to make it with ASCII symbols which, as far as i know a common way of going bout this.
Does anyone know how to make a random dungeon generator with ASCII symbols?
It would basically be a random maze generator and then i would add code in after to make rooms or make it mainly rooms. |
|
|
|
|
 |
Insectoid

|
Posted: Sun Dec 08, 2013 5:27 pm Post subject: RE:Can you make a Roguelike in turing? |
|
|
It sounds like you have no idea what you're getting into. The visuals are arguably the easiest part of the assignment, and doing it in ASCII is probably more complicated than using sprites.
Quote: Does anyone know how to make a random dungeon generator with ASCII symbols?
It's questions like this that say you aren't ready for this project. A random dungeon generator alone will require several hundred lines of code at least, and the code to actually do something with that dungeon is hundreds or even thousands more. |
|
|
|
|
 |
evildaddy911
|
Posted: Sun Dec 08, 2013 8:26 pm Post subject: Re: Can you make a Roguelike in turing? |
|
|
if you are still wanting to do this, i would suggest just a linear, "beat this room then move on" instead of a maze-style. The way i would do it is using classes/polymorphism. create a "room" class, then sub classes for the different types of rooms. then decide on how many of each type of room (for more random dungeons, make it a range, ie. 2-4 skeleton rooms, 1-3 zombie rooms etc.). then all you need to do is make an array of "room" objects, and using random # generators, decide what order the rooms are in.
some pseudocode:
code: | class Room % base class for the rooms
cleared: boolean
monsters: Monster
end class
class TreasureRoom, parent: Room
/* contains data for treasure rooms, subclass of Room */
end class
class monsterRoom, parent: Room
/* contains data for monster rooms, subclass of Room */
end class
/* im only showing 2 rooms in this example, you should have more than 2 in a finished product */
numTreasure = Rand.Int (minTreasure, maxTreasure)
/* decide how many of each room to use */
numMonster = Rand.Int (minMonster, maxMonster)
/* the total number of rooms */
numRooms = numTreasure + numMonster
/* the LINEAR array for all the rooms */
rooms: Room array (numRooms)
for i: 1 to numRooms
/* this is the part where it decides which rooms are where */
r = Rand.Int (0, numRooms - i) /* randomly pick a number from 0 to the number of rooms left -1 */
/* numTreasure and numMonster are the # of treasure rooms and monster rooms (respectively) left to place */
if r < numTreasure
/* place a treasure room in this position */
rooms(i) = new Treasure
numTreasure -= 1 /* 1 down, X left to go */
else if r < numTreasure + numMonster
/* if you only have 2 types of rooms, use "else" instead of "elsif". repeat adding the next room type until you get to the end */
rooms(i) = new Monster
numMonster -= 1
end if
end for |
as you can see, a really basic dungeon generator doesnt take that much coding, it just takes some creativity to make each room unique. note that this just room placement. it doesnt make rooms harder as you progress, or generate treasure in the treasure rooms. that would require a few more lines of code, but nothing major as long as you stick to the basics; to make things harder as you progress, just make the monsters' levels equal to their room #, and to generate treasure, simply say something like "treasure = Rand.Int(1, numItems)" |
|
|
|
|
 |
Tony
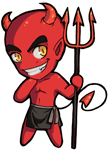
|
Posted: Sun Dec 08, 2013 8:45 pm Post subject: Re: RE:Can you make a Roguelike in turing? |
|
|
Sicarius @ Sun Dec 08, 2013 4:56 pm wrote: Does anyone know how to make a random dungeon generator with ASCII symbols?
The dungeon generation and "ASCII art" are two completely independent systems. Actually, there are at least 3 parts involved here.
- dungeon data: this can be either static, or randomly/procedurally generated
- an engine that drives that dungeon (loading data, keeping track of states, executing events, etc.)
- a graphics system that would display a representation of the current state of the dungeon. This could be ASCII, sprite, 3D, etc. Dwarf Fortress is an example that has mods for all 3 graphics modes. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Raknarg

|
Posted: Sun Dec 08, 2013 9:51 pm Post subject: RE:Can you make a Roguelike in turing? |
|
|
dwarf fortress has a 3d mod? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
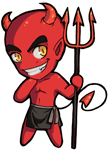
|
|
|
|
 |
|
|