Changing \ to / for location
Author |
Message |
badadvice
|
Posted: Mon May 06, 2013 2:09 pm Post subject: Changing \ to / for location |
|
|
What is it you are trying to achieve?
I am trying to get a file location I have in a txt and use it in my Turing program
What is the problem you are having?
The file location in the txt has "\" as in C:\windows\system32... and turing uses "/" for file locations. I cant seem able to find a way to convert all of the \ in the txt to /
Please specify what version of Turing you are using
4.1.1 |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Nathan4102
|
Posted: Mon May 06, 2013 2:20 pm Post subject: RE:Changing \ to / for location |
|
|
Once you've loaded the file location to a string, you can check, char by char, whether a character int he string is a \ or not. If it is, change it to a /. If you need help with the code, I can start you off. |
|
|
|
|
 |
badadvice
|
Posted: Mon May 06, 2013 2:23 pm Post subject: Re: Changing \ to / for location |
|
|
I tried that. When I wrote: if (randomstring) = "\" then ... 'then' had turned red also and it gave me an error when I tried running the program. can you please give me an idea of a code I could use. |
|
|
|
|
 |
Tony
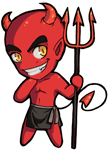
|
Posted: Mon May 06, 2013 2:29 pm Post subject: RE:Changing \ to / for location |
|
|
\ is an escape character. To understand it, consider the following:
1. String literals are enclosed in quotes -- var my_string : string := "some string"
2. Consider a case where you want to have a quote as part of that text -- "string with "quotes""
In the latter case, the string is taken as "string with " and the compiler doesn't know what to do with quotes"" part, as that is not valid code. Errors! \ escapes
var my_string : string := "string with \"quotes\""
What if you want \ inside of your string? Escape the escape character!
var path : string := "C:\\windows\\system32"
Which is what Turing "uses". |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Nathan4102
|
|
|
|
 |
badadvice
|
Posted: Mon May 06, 2013 7:35 pm Post subject: Re: Changing \ to / for location |
|
|
Tony's method does not work with what I am trying to do. I would appreciate if you could give me the code with ord(92) as I keep getting errors when I try it  |
|
|
|
|
 |
Nathan4102
|
Posted: Mon May 06, 2013 7:44 pm Post subject: RE:Changing \ to / for location |
|
|
A blend between my method and Tony's method.
Turing: | var astring : string := "C\\programfiles\\something"
var newstring : string := ""
for i : 1 .. length(astring )
if astring (i ) = chr(92) then
newstring := newstring + "/"
else
newstring := newstring + astring (i )
end if
end for
put newstring |
Output:
code: | C/programfiles/something |
|
|
|
|
|
 |
Zren

|
Posted: Mon May 06, 2013 8:58 pm Post subject: RE:Changing \ to / for location |
|
|
Homework: Make a generic function that will return a string with any character replace with another character.
Turing: | fcn stringReplace (str : string, oldChar : string, newChar : string) : string |
You could then use:
Turing: | stringReplace (filename, "\\", "/") |
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
badadvice
|
Posted: Mon May 06, 2013 9:35 pm Post subject: Re: Changing \ to / for location |
|
|
Thanks everyone for your help but I do not think you understand my problem. I have only single \, not doubles like \\. This is what my problem is, making Turing change them to /. |
|
|
|
|
 |
Tony
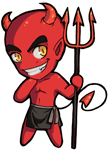
|
Posted: Mon May 06, 2013 9:46 pm Post subject: Re: Changing \ to / for location |
|
|
badadvice @ Mon May 06, 2013 9:35 pm wrote: I have only single \, not doubles like \\.
I don't think you were paying attention to my post above. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
jr5000pwp
|
Posted: Tue May 07, 2013 7:57 am Post subject: Re: Changing \ to / for location |
|
|
Wikipedia has a straight and to the point sub-article on escape characters in programming. http://en.wikipedia.org/wiki/Escape_character#Programming_and_data_formats
To explain, When you type in: Turing: | if myString = """ then
put "Quotation mark!"
end if |
you'll immediately notice by the syntax highlighting that something is wrong. Let's step through the if statement.
Turing encounters a quotation mark and opens a string. Then turing encounters a quotation mark and ends the string. Finally turing encounters a quotation mark and opens another string. Now everything after the third quotation mark is read as a string and not code!
To fix this we need to tell turing that our second quotation mark is to be read as a character and not as a string terminator, we can do this using escape characters.
In turing, and many other languages, the escape character is a backslash '\'. When you put that in a string, the next character is interpreted specially. In the case of our quotation mark, which would normally be a string terminator, it is now interpreted as any other character would and put into the string.
Here is the first example but fixed by using this escape character to tell turing not to end the string there: Turing: | if myString = "\"" then
put "Quotation mark!"
end if |
Now, what if we replace the middle quotation with a backslash? Turing: | if myString = "\" then
put "Backslash!"
end if |
You'll notice an issue with this one as well, for the same reason, let's step through.
Turing encounters a quotation mark and opens a string. Next it encounters a backslash and prepares to interpret the next character as special. This next character is a quotation mark, so it puts the quotation mark into the string. Oh no, we haven't closed our string and the rest is interpreted as a string!
To fix this we use the same approach, we want to interpret the backslash as a normal character and not an escape character. By putting a backslash before the backslash we tell turing to interpret it as a string rather than an escape character. Turing: | if myString = "\\" then
put "Backslash!"
end if | Don't be confused with how this is read, turing reads it as a string containing only one backslash.
When reading from a file, if I'm not mistaken, it doesn't need to have escape characters because things such as \n have their own character.
Now, what this means is that if you read in a string like so from a text file Quote: C:\Program Files\My Program\ then it will be read as exactly that in turing.
This still doesn't solve the issue of comparing the input string, but wait, we did that earlier with the "\\".
What Turing: | if myChar = "\\" then
put "Backslash"
end if | does is checks if the character you are passing is the same as a single backslash, even though it reads visually as a double backslash, it is read by turing as a single backslash.
With a modified version of that if statement, you should be able to step through your input string and replace all backslashes with forward slashes. |
|
|
|
|
 |
|
|