Animations and Image handling help!
Author |
Message |
Nathan4102
|
Posted: Tue Mar 19, 2013 3:16 pm Post subject: Animations and Image handling help! |
|
|
What is it you are trying to achieve?
An animation without wiping previously printed images.
What is the problem you are having?
In my animation procedure, I use cls to clear the screen inbetween frames, but that wipes all my other images that I've printed.
Describe what you have tried to solve this problem
I have tried looking for an alternative for cls that only clears a certain image (The image we are animating) but I haven't found one. I also have a very messy solution, explained below.
Post any relevant code (You may choose to attach the file instead of posting the code if it is too long)
The code for the program is below. Note that the code is very messy, I'll clean it up later. The program does animations in proc ImageInAndOut, but it uses cls to clear the screen in between frames, which wipes other images. This occurs in proc NextSlide, when the animated point is coming across the screen, it wipes the title, WhatIsCPUImage. A messy solution would be to use an array of booleans and a large amount of if statements in my animation procedure, which would check where we are in the program, and display the appropriate images while animating, like I have it doing with the background image at the moment, but thats very messy, and there must be a better way. Thanks so much for whoever helps me!
Turing: |
import GUI
var imageX : int := - 700
var counter : int := 1
var Background1 : int := Pic.FileNew ("Background1.jpg")
var TitleImage : int := Pic.FileNew ("TitleImage.bmp")
var NameImage : int := Pic.FileNew ("NameImage.bmp")
var WhatIsCPUImage : int := Pic.FileNew ("WhatIsCPUImage.bmp")
var Slide1Point1 : int := Pic.FileNew ("Slide1Point1.bmp")
var half, done : boolean := false
var slideNumber, pointNumber : int := 0
var button1 : int
var button2 : int
procedure MoveTitleIn (image, imageY, waitTime, halfX, stopnum : int)
if counter = 0 or (imageX > stopnum - 5 and imageX < stopnum + 5) then
half := true
delay (waitTime )
elsif imageX < halfX then
counter := counter + 1
else
counter := counter - 1
end if
imageX := imageX + counter
cls
Pic.Draw (Background1, 0, 0, picCopy)
Pic.Draw (image, imageX, imageY, picMerge)
View.Update
end MoveTitleIn
procedure MoveTitleOut (image, imageY : int)
if imageX < 1265 then
counter := counter + 1
else
done := true
end if
imageX := imageX + counter
cls
Pic.Draw (Background1, 0, 0, picCopy)
Pic.Draw (image, imageX, imageY, picMerge)
View.Update
end MoveTitleOut
procedure ImageInAndOut (image, imageY, waitTime, halfX, stopat : int, exitathalf : boolean)
loop
if half = false then
MoveTitleIn (image, imageY, waitTime, halfX, stopat )
else
if exitathalf = true then
done := true
exit
else
MoveTitleOut (image, imageY )
end if
end if
if done = true then
exit
end if
delay (4)
end loop
imageX := - 900
counter := 1
half := false
done := false
end ImageInAndOut
procedure NextPoint
pointNumber := pointNumber + 1
case pointNumber of
label 1 :
cls
Pic.Draw (Background1, 0, 0, picCopy)
Pic.Draw ( WhatIsCPUImage, 216, 550, picMerge)
ImageInAndOut (Slide1Point1, 480, 0, - 400, 20, true)
button2 := GUI.CreateButton (880, 600, 0, "Next Point", NextPoint )
View.Update
end case
end NextPoint
procedure NextSlide
slideNumber := slideNumber + 1
case slideNumber of
label 1 :
cls
Pic.Draw (Background1, 0, 0, picCopy)
View.Update
ImageInAndOut (WhatIsCPUImage, 550, 0, - 330, 212, true)
imageX := - 900
counter := 1
half := false
done := false
GUI.Disable (button1 )
button2 := GUI.CreateButton (880, 600, 0, "Next Point", NextPoint )
View.Update
end case
end NextSlide
View.Set ("Graphics:1000;645, offscreenonly")
Pic.Draw (Background1, 0, 0, picCopy)
Pic.Draw (TitleImage, imageX, 600, picMerge)
delay (2000)
ImageInAndOut (TitleImage, 300, 2500, - 290, 999, false)
ImageInAndOut (NameImage, 300, 2500, - 380, 999, false)
button1 := GUI.CreateButton (880, 600, 0, "Next Slide", NextSlide )
View.Update
loop
exit when GUI.ProcessEvent
end loop
|
Please specify what version of Turing you are using
4.1 |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
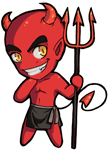
|
Posted: Tue Mar 19, 2013 3:20 pm Post subject: RE:Animations and Image handling help! |
|
|
cls is as good as Draw.FillBox(0,0,maxx,maxy,background_colour)
If you want to "clear" less area, you can draw a smaller box. Works for some cases, but it's typically simpler to just redraw everything. Read this recent thread on the details, it had the same question -- http://compsci.ca/v3/viewtopic.php?t=33353 |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Nathan4102
|
Posted: Tue Mar 19, 2013 3:41 pm Post subject: Re: Animations and Image handling help! |
|
|
Wow, thats awesome, thanks!
The only thing is though, that my background isn't just a plain color, its actually an image. Is there any alternative to changing my background to a solid color? This is my new code:
Turing: |
import GUI
var imageX : int := - 700
var counter : int := 1
var Background1 : int := Pic.FileNew ("Background1.jpg")
var TitleImage : int := Pic.FileNew ("TitleImage.bmp")
var NameImage : int := Pic.FileNew ("NameImage.bmp")
var WhatIsCPUImage : int := Pic.FileNew ("WhatIsCPUImage.bmp")
var Slide1Point1 : int := Pic.FileNew ("Slide1Point1.bmp")
var half, done : boolean := false
var slideNumber, pointNumber : int := 0
var button1 : int
var button2 : int
procedure MoveTitleIn (image, imageY, waitTime, halfX, stopnum : int)
if counter = 0 or (imageX > stopnum - 5 and imageX < stopnum + 5) then
half := true
delay (waitTime )
elsif imageX < halfX then
counter := counter + 1
else
counter := counter - 1
end if
imageX := imageX + counter
Draw.FillBox(imageX - 5, imageY - 5, imageX + 600, imageY + 20, 0)
% Pic.Draw (Background1, 0, 0, picCopy)
Pic.Draw (image, imageX, imageY, picMerge)
View.Update
end MoveTitleIn
procedure MoveTitleOut (image, imageY : int)
if imageX < 1265 then
counter := counter + 1
else
done := true
end if
imageX := imageX + counter
Pic.Draw (Background1, 0, 0, picCopy)
Pic.Draw (image, imageX, imageY, picMerge)
View.Update
end MoveTitleOut
procedure ImageInAndOut (image, imageY, waitTime, halfX, stopat : int, exitathalf : boolean)
loop
if half = false then
MoveTitleIn (image, imageY, waitTime, halfX, stopat )
else
if exitathalf = true then
done := true
exit
else
MoveTitleOut (image, imageY )
end if
end if
if done = true then
exit
end if
delay (4)
end loop
imageX := - 900
counter := 1
half := false
done := false
end ImageInAndOut
procedure NextPoint
pointNumber := pointNumber + 1
case pointNumber of
label 1 :
Pic.Draw ( WhatIsCPUImage, 216, 550, picMerge)
ImageInAndOut (Slide1Point1, 480, 0, - 400, 20, true)
button2 := GUI.CreateButton (880, 600, 0, "Next Point", NextPoint )
View.Update
end case
end NextPoint
procedure NextSlide
slideNumber := slideNumber + 1
case slideNumber of
label 1 :
Pic.Draw (Background1, 0, 0, picCopy)
ImageInAndOut (WhatIsCPUImage, 550, 0, - 330, 212, true)
imageX := - 900
counter := 1
half := false
done := false
GUI.Disable (button1 )
button2 := GUI.CreateButton (880, 600, 0, "Next Point", NextPoint )
View.Update
end case
end NextSlide
View.Set ("Graphics:1000;645, offscreenonly")
Pic.Draw (Background1, 0, 0, picCopy)
Pic.Draw (TitleImage, imageX, 600, picMerge)
delay (2000)
ImageInAndOut (TitleImage, 300, 2500, - 290, 999, false)
ImageInAndOut (NameImage, 300, 2500, - 380, 999, false)
button1 := GUI.CreateButton (880, 600, 0, "Next Slide", NextSlide )
View.Update
loop
exit when GUI.ProcessEvent
end loop
|
This is what the screen looks like after animations now: http://i0.simplest-image-hosting.net/picture/untitled307.png |
|
|
|
|
 |
evildaddy911
|
Posted: Tue Mar 19, 2013 5:55 pm Post subject: RE:Animations and Image handling help! |
|
|
one way would be to draw the background image instead of a rectangle, however, if you only want to clear a certain part of the screen, then your going to need several different pictures showing different parts of the background
EDIT: looking at the pictures, go with the first option |
|
|
|
|
 |
|
|