How to reverse a string by using recursion?
Author |
Message |
Fed
|
Posted: Sun Feb 24, 2013 3:34 pm Post subject: How to reverse a string by using recursion? |
|
|
I know how to reverse a string using loop
by taking the length of the string and reverse the characters
But i have a problem with recursion reverse
Anyone has any idea to help me plz ? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Insectoid

|
Posted: Sun Feb 24, 2013 4:30 pm Post subject: RE:How to reverse a string by using recursion? |
|
|
Your function should take a string as a parameter. From that string, select one character. You will then want to call your function and pass it the rest of the string without that letter. Add the result of that function call to the letter you picked (or add the letter you picked to the result of that function call) and return it. |
|
|
|
|
 |
Fed
|
Posted: Sun Feb 24, 2013 4:53 pm Post subject: Re: RE:How to reverse a string by using recursion? |
|
|
Insectoid @ Sun Feb 24, 2013 4:30 pm wrote: Your function should take a string as a parameter. From that string, select one character. You will then want to call your function and pass it the rest of the string without that letter. Add the result of that function call to the letter you picked (or add the letter you picked to the result of that function call) and return it.
Thank you for ur help! I understand the concept, but im new to Turing and recursion, i have some difficulties to do this step u mentioned: "You will then want to call your function and pass it the rest of the string without that letter" |
|
|
|
|
 |
Insectoid

|
Posted: Sun Feb 24, 2013 11:39 pm Post subject: RE:How to reverse a string by using recursion? |
|
|
Do you know how to remove a letter from a string?
Do you know how to call a function from itself? |
|
|
|
|
 |
btiffin

|
Posted: Mon Feb 25, 2013 6:04 am Post subject: RE:How to reverse a string by using recursion? |
|
|
Had to hit BRK, Insectoid posted a recursive. |
|
|
|
|
 |
Fed
|
Posted: Mon Feb 25, 2013 8:37 am Post subject: Re: RE:How to reverse a string by using recursion? |
|
|
Insectoid @ Sun Feb 24, 2013 11:39 pm wrote: Do you know how to remove a letter from a string?
Do you know how to call a function from itself?
I dont know how to remove a letter from a string, thats the problem im having, or else this would be easy lol
Can u teach me ? |
|
|
|
|
 |
Tony
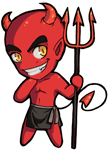
|
Posted: Mon Feb 25, 2013 12:28 pm Post subject: RE:How to reverse a string by using recursion? |
|
|
A string is an array of characters. You can access it's substrings by index or range
code: |
var text : string := "word"
put text(1)
put text(2..*)
|
see substring |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Fed
|
Posted: Mon Feb 25, 2013 5:23 pm Post subject: Re: RE:How to reverse a string by using recursion? |
|
|
Tony @ Mon Feb 25, 2013 12:28 pm wrote: A string is an array of characters. You can access it's substrings by index or range
code: |
var text : string := "word"
put text(1)
put text(2..*)
|
see substring
I know how to display, but i dont know how to remove a letter by recursion, i know how to use loop to do this, but not recursion, |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
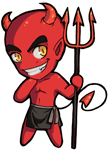
|
Posted: Mon Feb 25, 2013 7:08 pm Post subject: RE:How to reverse a string by using recursion? |
|
|
you could keep some counter to tell what step of the recursion you are on (at which point you are just implementing loops, and it's not very interesting), or... if you always just work with the first letter of whatever part of the strings hasn't been processed yet, then you wouldn't even need any explicit counting.
Ultimately Computer Science is not about memorizing a series of very specific tasks (as is unfortunately the case for many other courses), but learning the ability to figure out problems, by relating them to previous experiences. Start with a very basic example of recursion (e.g. how would you count down from 10 to 0 using recursion?) and build on that.
Saying "I don't know how to do <this specific assignment>" is along the same lines of saying "hey guys, does anyone here know how I can build Halo 5?". Obviously a stretch, although some kids do ask questions like that.
So once again -- get a hold of the basics of recursion. Get a hold of the basics of working with substrings. Then build on top of those two concepts to come up with a new solution to a new problem. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Fed
|
Posted: Tue Feb 26, 2013 5:14 pm Post subject: Re: RE:How to reverse a string by using recursion? |
|
|
Tony @ Mon Feb 25, 2013 7:08 pm wrote: you could keep some counter to tell what step of the recursion you are on (at which point you are just implementing loops, and it's not very interesting), or... if you always just work with the first letter of whatever part of the strings hasn't been processed yet, then you wouldn't even need any explicit counting.
Ultimately Computer Science is not about memorizing a series of very specific tasks (as is unfortunately the case for many other courses), but learning the ability to figure out problems, by relating them to previous experiences. Start with a very basic example of recursion (e.g. how would you count down from 10 to 0 using recursion?) and build on that.
Saying "I don't know how to do <this specific assignment>" is along the same lines of saying "hey guys, does anyone here know how I can build Halo 5?". Obviously a stretch, although some kids do ask questions like that.
So once again -- get a hold of the basics of recursion. Get a hold of the basics of working with substrings. Then build on top of those two concepts to come up with a new solution to a new problem.
Alright, thax, i got the question btw. I used procedure instead of function, i thought that the teacher only allowed us to use function, it was sort of misunderstood the question.
Ty for ur time. |
|
|
|
|
 |
Zren

|
Posted: Wed Feb 27, 2013 1:38 am Post subject: Re: RE:How to reverse a string by using recursion? |
|
|
Fed @ Tue Feb 26, 2013 5:14 pm wrote:
Alright, thax, i got the question btw. I used procedure instead of function, i thought that the teacher only allowed us to use function, it was sort of misunderstood the question.
Ty for ur time.
Mind posting the code you used to solve it? |
|
|
|
|
 |
Fed
|
Posted: Sat Mar 02, 2013 11:41 am Post subject: Re: How to reverse a string by using recursion? |
|
|
sure
var letters : string := "abcdefg"
var num : int := length (letters)
proc Reverse (letternum : int)
if letternum = 0 then
put " "
elsif letternum >= 1 then
put letters (letternum) ..
Reverse (letternum - 1)
end if
end Reverse
Reverse (num) |
|
|
|
|
 |
Tony
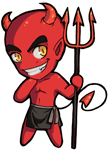
|
Posted: Sat Mar 02, 2013 3:42 pm Post subject: RE:How to reverse a string by using recursion? |
|
|
that's... not really in the spirit of recursion. You have constructed a for-loop using recursion, and then used that loop to step through the string. A better approach would be along the lines of
Quote:
ruby-1.9.3-p0 :001 > def reverse(text)
ruby-1.9.3-p0 :002?> return text if text.size < 2
ruby-1.9.3-p0 :003?> return text[-1] + reverse(text[0..-2])
ruby-1.9.3-p0 :004?> end
=> nil
ruby-1.9.3-p0 :005 > reverse("string")
=> "gnirts"
Which demonstrates the core recursive thinking:
- if text size is less than 2, then the string is already reversed. Easy!
- assumes that the "reverse" function is already implemented for inputs one less in size. Just grab the last letter and the result of that other "reverse" function
- putting the two together, you have implemented that "other reverse function". Done. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
|
|