Turing SideScrolling Zombie Game Help
Author |
Message |
TuringNoob10101
|
Posted: Sat Jan 19, 2013 8:35 pm Post subject: Turing SideScrolling Zombie Game Help |
|
|
What is it you are trying to achieve?
Trying to make a side scroller zombie game.
What is the problem you are having?
I have everything done i just need some help with learning how to shoot regular bullets/drawfillovals making the zombie image disappear/cls. Also another thing that im having trouble with is how to do Collision detection so when the Zombie Image touches my
character image it clears the screen and says game over! I dont know where to place these coding either..
Describe what you have tried to solve this problem
I have looked up tutorials and the coding from other submissions however since im new to turing it is really hard for me and could not find anything that could help my need. One problem was that Collision Detection tutorial wasnt helpful for people using images and i could not
find a tutorial on how to shoot.
Post any relevant code (You may choose to attach the file instead of posting the code if it is too long)
setscreen ("graphics:800;400")
%Variables
var zombielogo : int := Pic.FileNew ("Title.bmp")
var HumanRight : int := Pic.FileNew ("HumanSprite.bmp")
var HumanLeft : int := Pic.FileNew ("HumanSprite copy 1.bmp")
var Zombie : int := Pic.FileNew ("Zombie1.bmp")
var ArrowKeys : int := Pic.FileNew ("arrow-keys-vectors_637061.bmp")
var SpaceBar : int := Pic.FileNew ("computer_key_Space_bar.bmp")
var chars : array char of boolean
var x, y, x1, y1 : int
x := 100
y := 100
x1 := 450
y1 := 100
%Font
var font1 : int
font1 := Font.New ("Stencil:19")
var font2 : int
font2 := Font.New ("Stencil:22")
var font3 : int
font3 := Font.New ("Stencil:14")
var font4 : int
font4 := Font.New ("Stencil:12")
%Menu
loop
Input.KeyDown (chars)
Pic.Draw (zombielogo, 0, 0, picCopy)
if chars (' ') then
exit
end if
end loop
cls
%Story
delay (1800)
Font.Draw ("It all happened, 3 years ago.", 200, 250, font1, black)
delay (2000)
cls
Font.Draw ("The living began to fall, and the fallen began to rise.", 30, 250, font1, black)
delay (2000)
cls
Font.Draw ("Now humanity has to live in fear from the Walking Dead.", 25, 250, font1, black)
delay (2000)
cls
Font.Draw ("The fate of humankind lies on your shoulders...", 80, 250, font1, black)
Pic.Draw (HumanRight, maxx div 2.4, maxy div 4.5, 3)
delay (2000)
cls
loop
Input.KeyDown (chars)
Font.Draw ("Begin you journey.", 260, 100, font1, red)
Font.Draw ("(Press Space)", 320, 80, font3, black)
Font.Draw ("(Use Arrow keys to move)", 80, 200, font4, black)
Font.Draw ("(Use Space to shoot)", 490, 200, font4, black)
Pic.Draw (ArrowKeys, 100, 200, 3)
Pic.Draw (SpaceBar, 400, 250, 3)
if chars (' ') then
exit
end if
end loop
cls
% Street / Background
drawfillbox (800, 70, 00, 99, darkgrey)
drawfillbox (800, 00, 00, 70, black)
Font.Draw ("Kill the Zombie !", 282, 320, font2, red)
%Character Movement
process Human
loop
drawfilloval (160, 178, 3, 3, black)
Input.KeyDown (chars)
Pic.Draw (HumanRight, x, y, 0)
if chars (KEY_RIGHT_ARROW) then
x := x + 5
end if
if chars (KEY_LEFT_ARROW) then
Pic.Draw (HumanLeft, x, y, 0)
x := x - 5
end if
delay (15)
if x > 820
then
x := -150
end if
end loop
end Human
fork Human
% Zombie Movement
loop
loop
Pic.Draw (Zombie, x1, y1, 0)
delay (10)
x1 := x1 - 1
exit when x1 = 160
end loop
loop
Pic.Draw (Zombie, x1, y1, 0)
delay (10)
x1 := x1 + 1
exit when x1 = 500
end loop
end loop
Please specify what version of Turing you are using
4.1 |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
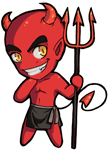
|
Posted: Sat Jan 19, 2013 9:04 pm Post subject: Re: Turing SideScrolling Zombie Game Help |
|
|
TuringNoob10101 @ Sat Jan 19, 2013 8:35 pm wrote: One problem was that Collision Detection tutorial wasnt helpful for people using images and i could not
find a tutorial on how to shoot.
You don't need to make your collision pixel perfect -- most games don't bother with that, unless it's _really_ important to handle close calls with much precision. Approximate your characters with a circle (or three) and the players are unlikely to notice.
There's nothing inherently special about bullets, they are like tiny player characters that you don't actually control (so simpler!). A typical approach is to have an array of active bullets on the screen, and a forloop to advance each bullet a step during the frame of the game. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
TuringNoob10101
|
Posted: Sat Jan 19, 2013 11:15 pm Post subject: RE:Turing SideScrolling Zombie Game Help |
|
|
I still dont know what you mean? Sorry im a beginner to turing and i didnt understand half the stuff you said. Can you give me a simple coding that can make my character shoot and zombie disappear? Like i dont expect you to finish this for me but atleast tell me what i need to do. Give me the coding and ill add in my characters |
|
|
|
|
 |
evildaddy911
|
Posted: Sun Jan 20, 2013 9:21 am Post subject: RE:Turing SideScrolling Zombie Game Help |
|
|
What he means about the circles is, for example, using 1 circle to represent the legs, another to represent the body and a third to represent the head. In your case, using zombies, a fourth is optional to represent the arms.
Tonys comment about bullets means that you pick an x and y value to move each bullet by. Each frame, you advance the bullet and check collision, you dont have to worry about input for them at all.
Basic bullet code:
Turing: | var bulletx : array 1 .. 200 of int
var bullety : array 1 .. 200 of int
var bulletalive: array 1 .. 200 of boolean
% initialize the arrays here
Loop
For i : 1 .. 200
if bulletalive (i) then
bulletx (i) := bulletx (i) + 2
bullety (i) := bullety (i) + 1
if (collision) then
% do something
end if
end if
end for
End loop
|
|
|
|
|
|
 |
TuringNoob10101
|
Posted: Sun Jan 20, 2013 12:36 pm Post subject: RE:Turing SideScrolling Zombie Game Help |
|
|
Do you mean i do drawfilloval to represent each body part and then do if what dot color and if they collide game over?? How would i use circles to represent them and make them move while the character moves? |
|
|
|
|
 |
evildaddy911
|
Posted: Sun Jan 20, 2013 4:58 pm Post subject: Re: Turing SideScrolling Zombie Game Help |
|
|
you don't necessarily need to use drawfilloval / whatdotcolor to do this, but yes, thats the really basic way to do it. Another way is to check collision with each of the circles; that way, you can give different amounts of points for a head, body or leg shot
For the circles, you would need to figure out their relationship to the character's x and y. for example,
playerX, playerY is where the character touches the ground
Turing: |
% legs
drawfilloval (playerX, playerY + 20, 20, 20, black)
% body
drawfilloval (playerX, playerY + 60, 25, 25, black)
% head
drawfilloval (playerX, playerY + 95, 10, 10, black)
|
|
|
|
|
|
 |
Tony
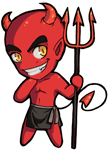
|
Posted: Sun Jan 20, 2013 7:46 pm Post subject: RE:Turing SideScrolling Zombie Game Help |
|
|
As evildaddy911 points out, you don't need to actually draw any circles to be able to figure out if they would collide or not.
If I tell you that there are two circles, each with radius of 1, and they are 1 unit of distance apart, then you should be able to tell me if they are colliding or not, without having to draw them on paper. Same thing for your game -- imaginary collision circles. Math! |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
TuringNoob10101
|
Posted: Sun Jan 20, 2013 8:47 pm Post subject: RE:Turing SideScrolling Zombie Game Help |
|
|
I was able to solve the problem where colliding the two characters would make it game over with Math.Distance but I still dont know how to make my character shoot... |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
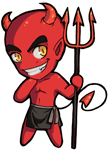
|
Posted: Mon Jan 21, 2013 1:11 am Post subject: RE:Turing SideScrolling Zombie Game Help |
|
|
Okay. What do you figure you will need to make a shot?
I find that it's often to think of such things in terms of a board game (that you play at 30 moves per second). If you wanted to introduce shooting into a board game, what would you need?
- token for a bullet (multiple tokens?)
- rules for advancing those tokens
- etc.
Describe that first. You can then express that system in code. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
|
|