Picture cut off when using Pic.Rotate + Moving a picture forward in the direction it is currently facing.
Author |
Message |
TrophyChase
|
Posted: Sun May 27, 2012 3:20 pm Post subject: Picture cut off when using Pic.Rotate + Moving a picture forward in the direction it is currently facing. |
|
|
What is it you are trying to achieve?
I am trying to create a program in which I can control the movements of a tank in the following ways:
-Rotate the tank's facing when I press "a" or "d" using Pic.Rotate
-Move the tank forward/backward in the direction it is currently facing when I press w/s.
What is the problem you are having?
I have 2 problems:
- When my tank rotates, part of it is cut off during the rotation.
- (bigger problem) I have absolutely no idea of how to approach the problem of moving the tank in the direction it is facing.
I have searched the turing forums and google, and have managed to glean that this will require the application of trigonometry, but I don't really know where to start.
Describe what you have tried to solve this problem
- for the first problem, I have tried changing the background, size of the tank itself, size of the picture, and using picCopy instead of picMerge with Pic.Draw
- for the second problem, as previously stated, I have puzzled a bit, done quite a bit of research, but am thoroughly stymied.
Post any relevant code (You may choose to attach the file instead of posting the code if it is too long)
Turing: |
setscreen ("offscreenonly")
% variable + constants
const colourtank : int := 12
const turretcentreX := 36 div 2 + 5
const turretcentreY := 36 div 2
const turretradius := 8
var pangle := 0
var key : array char of boolean
var tank : array 0 .. 359 of int
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
% draws tank body
drawfillbox (0, 0, 36, 36, blue)
% draws tracks
drawfillbox (0, 0, 36, 8, black)
drawfillbox (0, 36, 36, 36 - 8, black)
% draws turret
drawfilloval (turretcentreX, turretcentreY, turretradius, turretradius, colourtank )
% draws gun
drawfillbox (turretcentreX, turretcentreY + 4, turretcentreX + 20, turretcentreY - 4, colourtank )
% draws turretborder for accentuation
drawoval (turretcentreX, turretcentreY, turretradius, turretradius, black)
tank (0) := Pic.New (0, 0, 44, 36)
% creates 360 pictures of tank, one for each degree rotated
for angles : 0 .. 359
tank (angles ) := Pic.Rotate (tank (0), angles, 18, 18)
end for
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
% This section determines if the user is pressing "a" or "d" and increases/decreases the
% player angle respectively
loop
cls
Input.KeyDown (key )
if key ('a')
then
pangle := pangle + 1
end if
if key ('d')
then
pangle := pangle - 1
end if
% wraps around pangle
if pangle = - 1 then
pangle := 359
end if
if pangle = 360 then
pangle := 0
end if
% draws background
drawfillbox (0, 0, maxx, maxy, green)
% draws appropriate tank picture, based on pangle.
Pic.Draw (tank (pangle ), maxx div 2 - 36, maxy div 2 - 36, picCopy)
View.Update
delay (5)
end loop
|
Please specify what version of Turing you are using
I am using Turing 4.1.1
If you need any more information I will be happy to provide it.
BTW This is my first post on compsci.ca, so I hope I'm doing everything right. I've seen how friendly and helpful everyone here is, so thanks in advance for your input.
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
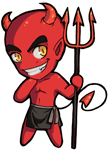
|
Posted: Sun May 27, 2012 3:44 pm Post subject: RE:Picture cut off when using Pic.Rotate + Moving a picture forward in the direction it is currently facing. |
|
|
the documentation on the use of Pic.Rotate talks about just that
Quote:
You can avoid losing parts of the picture by making certain there is a margin of background color on the left and bottom sides of the picture.
and shows an example picture with parts being cut off.
Second question involves breaking the movement into two components: move along X and move along Y. Every direction can be expressed as a combination of those two parts. Figuring out how large each of those parts is, for a constant total, involves trigonometry. You know one of the sides of a triangle, and all the angles; there are formulas for figuring out the rest.
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
TrophyChase
|
Posted: Sun May 27, 2012 4:36 pm Post subject: Re: Picture cut off when using Pic.Rotate + Moving a picture forward in the direction it is currently facing. |
|
|
I read the details about Pic.Rotate, but I still don't understand why I have the problem, since when I used picMerge as the mode, I did have background to the left and bottom sides of the picture, and my picture was still cut off. Am I missing something really obvious, or just not understanding correctly?
Thanks SO much for your response to my second question. I totally understand what I should be doing now!
|
|
|
|
|
 |
evildaddy911
|
Posted: Sun May 27, 2012 6:46 pm Post subject: RE:Picture cut off when using Pic.Rotate + Moving a picture forward in the direction it is currently facing. |
|
|
you need background color on ALL FOUR sides of the picture, and you will want the mode set to picMerge to avoid the white showing.
For the second problem, use the primary trig ratios/functions: sine, cosine and tangent.
ps, once you figure out the trig part, its generally easier to just skip the picture part and use a few drawpolygon calls instead of Pic.Draw/Pic.New/Pic.Rotate
|
|
|
|
|
 |
turinggirl
|
Posted: Sun May 27, 2012 8:55 pm Post subject: Re: Picture cut off when using Pic.Rotate + Moving a picture forward in the direction it is currently facing. |
|
|
try using maxx and maxy
|
|
|
|
|
 |
TrophyChase
|
Posted: Sun May 27, 2012 9:12 pm Post subject: Re: Picture cut off when using Pic.Rotate + Moving a picture forward in the direction it is currently facing. |
|
|
So this is what I have so far ... I am using picMerge, and have background on all 4 sides of the tank, but Turing is still cutting off sections of the tanks. I'm very confused. What am I doing wrong?
Description: |
Turing file + pics and map file. |
|
 Download |
Filename: |
Tank Game.zip |
Filesize: |
2.24 KB |
Downloaded: |
77 Time(s) |
|
|
|
|
|
 |
Tony
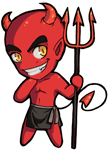
|
Posted: Mon May 28, 2012 1:35 am Post subject: Re: Picture cut off when using Pic.Rotate + Moving a picture forward in the direction it is currently facing. |
|
|
TrophyChase @ Sun May 27, 2012 4:36 pm wrote: Am I missing something really obvious, or just not understanding correctly?
The missing part is that the size of the rotated picture will be the same as of the original picture, meaning that some of the original will almost always be cut off. The suggested solution is to add enough margin around the image, such that the cutoff margin wouldn't matter.
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
evildaddy911
|
Posted: Mon May 28, 2012 2:56 pm Post subject: RE:Picture cut off when using Pic.Rotate + Moving a picture forward in the direction it is currently facing. |
|
|
what i usually do is:
take the width of the picture WITHOUT padding, then use that for the left/right padding. then do the exact same thing for the height/top/bottom.
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
|
|