BATTLESHIP - Computer Ship Placement Help
Author |
Message |
rgothmog
|
Posted: Sat Jan 17, 2004 1:37 pm Post subject: BATTLESHIP - Computer Ship Placement Help |
|
|
Hey I am making a battleship game and I'm trying to place the computer ships in random locations on a grid with 10 squares across and 10 down... so 10 x 10. I was planning on using arrays to do this and I was just wondering how this could be done. I'd appreciate it if anyone could help me on this. Thanks. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Thuged_Out_G
|
Posted: Sat Jan 17, 2004 2:15 pm Post subject: (No subject) |
|
|
just use Rand.Int for the coords
var x,y,x1,y1:int
x:=Rand.Int(1,somenum)
y:=Rand.Int(1,somenum)
y1:=Rand.Int(1,somenum)
x1:=Rand.Int(1,somenum)
then use those vars to draw your ships |
|
|
|
|
 |
rgothmog
|
Posted: Sat Jan 17, 2004 2:28 pm Post subject: (No subject) |
|
|
Yea i could use that within the array essentially with something like:
var shipplacement : array 1..10, 1..10 of int
and assign all values in the array to a X or something... and then when a random ship placement is generated it changed those values to O or something and then i can see if a ship is there when i click on a square to see if it's a hit or miss type thing. just not sure how do do the whole assigning properly since the ships are in boxes (x1,y1,x2,y2) and random coordinates for each ship still have to be in a row... if u know what i'm tyring to say lol  |
|
|
|
|
 |
Tony
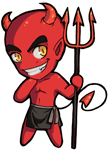
|
Posted: Sat Jan 17, 2004 4:46 pm Post subject: (No subject) |
|
|
what you do is you randomly pick a point, then randomly pick a direction from that point and see if you ship fits...
such as the random point is 1,1 random direction is north and ship is 4 boxes in length (battleship)... there for you check if
1,1
1,2
1,3
1,4
are abailable to place the ship |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
rgothmog
|
Posted: Sat Jan 17, 2004 5:49 pm Post subject: (No subject) |
|
|
thanks a lot. one more thing. How can i use the location of a picture to be put into an array so that if a part of the ship lies in a certain part of the grid then it's location is stored in an array. ex.. the battleship lies in 4 squares of the grid each 20 units in length.. and it goes from 200 to 280 on hte x axis, and 100 to 120 on the y axis. how could i put those coordinates into an array of 1..10, 1..10 of int? Thanks alot... hopefull that makes sence lol |
|
|
|
|
 |
Tony
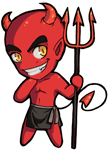
|
Posted: Sat Jan 17, 2004 6:58 pm Post subject: (No subject) |
|
|
well if for example each picture is 10x10 pixels, then its index*10 to get the location.
such as picture (2,3) would be drawn at 2*10, 3*10 (20,30) |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
rgothmog
|
Posted: Sun Jan 18, 2004 2:13 pm Post subject: (No subject) |
|
|
alrite so far i have something like this:
code: |
for i : 125 .. 325 by 20
count1 := 0
count := count + 1
for j : 125 .. 325 by 20
count1 := count1 + 1
if x1 < i and x1 > j and y1 < i and y1 > j then
shipalltaken (count, count1) := "D"
elsif x2 < i and x2 > j and y2 < i and y2 > j then
shipalltaken (count, count1) := "C"
end if
end for
end for
for i : 1 .. 10
for j : 1 .. 10
if shipalltaken (i, j) = "D" then
put "DESTROYER is located at: ", shipalltaken (i, j)
elsif shipalltaken (i, j) = "C" then
put "CRUISER is located at: ", shipalltaken (i, j)
end if
end for
|
Now the grid is located between 125 and 325 with each box 20 units in length. This code gives me an error... saying array subscript it out of range for this:
code: |
shipalltaken (count, count1) := "D"
|
I don't see what it is that I've done wrong... but obviously something lol. Now it's supposed to check teh box to see if part of the ship is located in that area.. and if so then it assigns a value of D to that location in the array (for destroyer) or C if the cruiser is at that location. If anyone knows how to do this I'd really appreciate the help... my ISU is due tomorrow . THANKS. |
|
|
|
|
 |
Tony
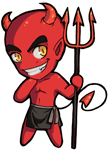
|
Posted: Sun Jan 18, 2004 2:31 pm Post subject: (No subject) |
|
|
gotta debug... stick a put counter, " ", counter1 statement in there to see what values are being passed.
and make sure your array is large enough (I dont see it decleared) |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
rgothmog
|
Posted: Sun Jan 18, 2004 2:32 pm Post subject: (No subject) |
|
|
hmm yea the array size might be the problem... but the array's are declared i just forgot to include them in that posted code... but they're:
var shipalltaken : array 1..10, 1..10 of string
i'll try that tho thanks |
|
|
|
|
 |
rgothmog
|
Posted: Sun Jan 18, 2004 3:13 pm Post subject: (No subject) |
|
|
well it wasn't the array size... but i did fix one problem with it... still not working tho ... do u have any idea at all what the code for this should look like??? |
|
|
|
|
 |
Tony
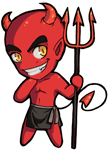
|
Posted: Sun Jan 18, 2004 5:47 pm Post subject: (No subject) |
|
|
you're just making everything too complicated. Instead of having for a ... b by c and having separate counters, just do
code: |
for row: 1..10
for column: 1..10
do whatever you need to do in that cell
|
it is just simpler that way. And when you draw, you just do your row*20, column*20 to get X/Y of the picture |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
TheXploder

|
Posted: Mon Jan 19, 2004 7:55 pm Post subject: (No subject) |
|
|
Good that I just have the thing for you...
I was working on a BattleShip game, and I actually finnished it...
Here is my enemy map generator...
code: |
var map : array 1 .. 10, 1 .. 10 of int
var r, r2 : int
loop
for c : 1 .. 10
for d : 1 .. 10
map (c, d) := 0
end for
end for
for i : 1 .. 4
randint (r, 1, 10)
randint (r2, 1, 10)
map (r, r2) := 1
end for
var dir : int
for i : 1 .. 3
randint (r, 1, 10)
randint (r2, 1, 10)
randint (dir, 1, 10)
map (r, r2) := 2
if dir = 1 then
if r < 10 and r2 < 10 then
map (r + 1, r2) := 2
end if
else
if r < 10 and r2 < 10 then
map (r, r2 + 1) := 2
end if
end if
end for
for i : 1 .. 2
randint (r, 1, 10)
randint (r2, 1, 10)
randint (dir, 1, 10)
map (r, r2) := 3
if dir = 1 then
if r < 9 and r2 < 10 then
map (r + 1, r2) := 3
map (r + 2, r2) := 3
end if
else
if r < 10 and r2 < 9 then
map (r, r2 + 1) := 3
map (r, r2 + 2) := 3
end if
end if
end for
for i : 1 .. 1
randint (r, 1, 10)
randint (r2, 1, 10)
randint (dir, 1, 10)
map (r, r2) := 4
if dir = 1 then
if r < 8 and r2 < 10 then
map (r + 1, r2) := 4
map (r + 2, r2) := 4
map (r + 3, r2) := 4
end if
else
if r < 10 and r2 < 8 then
map (r, r2 + 1) := 4
map (r, r2 + 2) := 4
map (r, r2 + 3) := 4
end if
end if
end for
var count : int := 0
for c : 1 .. 10
for d : 1 .. 10
if map (c, d) = 1 or map (c, d) = 2 or map (c, d) = 3 or map (c, d) = 4 then
count := count + 1
end if
end for
end for
if count = 20 then
exit
end if
end loop
for c : 1 .. 10
for d : 1 .. 10
put map (c, d) ..
end for
put ""
end for
|
That should do it...
If ya need help figuring it out just tell me...
Sorry that its not commented...
-=TheXploder=- |
|
|
|
|
 |
|
|