Help with this Collision Detection
Author |
Message |
dffhkhksg
|
Posted: Sun Jan 01, 2012 5:59 pm Post subject: Help with this Collision Detection |
|
|
What is it you are trying to achieve?
I want to create a brick breaker game, with a moving ball and column/rows for the bricks
What is the problem you are having?
Various glitches where the ball passes through a portion of the brick before detecting a collision, but the main problem i need fixed is that on certain events (ie ball hits a brick while it is moving up and left) which cause the wrong brick to be drawn (well, erased)
Describe what you have tried to solve this problem
Tweaking the parameters on the draw command, but i think I need some if-statements to truly fix the problem
Post any relevant code (You may choose to attach the file instead of posting the code if it is too long)
I basically have 9 checks around the ball and use whatdotcolour, an example of this would be
Turing: |
procedure erasebrick (column, row : int) %, above : string)
if movey <= 0 then %Ball is moving down
drawfillbox (column * 20 + 1, row * 10 + 2, (column + 1) * 20, (row - 1) * 10 + 3, white)
bricksleft + = - 1
elsif movey > 0 then %Ball is moving up
if movex <= 0 then
drawfillbox (column * 20 - 1, row * 10 + 1, (column + 1) * 20, (row + 1) * 10 + 3, white)
bricksleft + = - 1
elsif movex > 0 then %Right
drawfillbox (column * 20 + 1, row * 10 + 3, (column + 1) * 20, (row + 1) * 10 + 2, white)
bricksleft + = - 1
end if
end if
locate (1, 1)
put bricksleft
end erasebrick
%<Other collision checks>
elsif (whatdotcolour (round (ballx ) + 20, round (bally ) - 21) not= white) then %Hits a brick below the ball
erasebrick ((ballx + 20) div 20, (bally - 21) div 10)
|
Please specify what version of Turing you are using
4.1 |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Dreadnought
|
Posted: Sun Jan 01, 2012 8:57 pm Post subject: Re: Help with this Collision Detection |
|
|
I believe that your problem comes from the fact that while the center of the ball may be in one column/row its edges can collide with rectangles in other rows.
I see what you're trying to do with the columns and rows, but I think you've complicated this more than its needs to be.
You say that you check the colour of 9 points for collision. Well if a dot is not white then that single point must be in a rectangle. So the coordinates of that point can tell you the rectangle you hit. Now you just have to figure out which way to make the ball bounce afterwards.
Good luck. |
|
|
|
|
 |
Tony
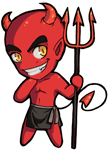
|
Posted: Sun Jan 01, 2012 9:49 pm Post subject: RE:Help with this Collision Detection |
|
|
- presumably a ball has more than 9 points to it, so some angles don't check for collision, until the ball is far enough into the block for other points to also "collide"
- if the ball is travelling "fast" (anything greater than 1pixel-per-frame really), it can make it up to (velocity - 1) pixels into the block before you do the check for "is the ball colliding right now" |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
chipanpriest

|
Posted: Mon Jan 02, 2012 12:32 pm Post subject: Re: Help with this Collision Detection |
|
|
I'm making a space invaders game and for the enemies, i just use a boolean variable to decide if theyre alive or not and all the enemies are in an array. Example:
Turing: | for i : 1 .. 10 % 10 enemies
if enemy (i) = true then % if theyre alive or not
if By + 20 >= E1y1 - 20 and Bx + 5 >= E1x (i) - 20 and Bx - 5 <= E1x (i) + 20 then % I use the x and y values of the bullet and the enemies to decide if they hit
enemy (i) = false %kills the enemy
end if
end if
end for
|
Instead of using whatdotcolour, I take the x and y values of the bullet (Bx & By) and the enemies (Ex & Ey) and that's how my program knows if there is a collision. I would also have a separate for statement drawing the enemies:
Turing: | for i : 1 .. 10 % 10 enemies
if enemy (i ) = true then % If the enemy is alive
Draw.FillBox (E1x (i ) - 20, E1y1 - 20, E1x (i ) + 20, E1y1 + 20, blue) % Draws the enemy
end if
end for
|
|
|
|
|
|
 |
Aange10

|
Posted: Mon Jan 02, 2012 2:04 pm Post subject: Re: RE:Help with this Collision Detection |
|
|
Tony @ 1/1/2012, 8:49 pm wrote: - if the ball is travelling "fast" (anything greater than 1pixel-per-frame really), it can make it up to (velocity - 1) pixels into the block before you do the check for "is the ball colliding right now"
Haha don't mean to be a Natzi but, it doesn't matter if the ball is traveling fast. The equation (velocity - 1) is still correct xD. 1 px per frame is just (1 - 1) = 0 |
|
|
|
|
 |
Dreadnought
|
Posted: Mon Jan 02, 2012 2:16 pm Post subject: Re: Help with this Collision Detection |
|
|
Aange10 wrote: Haha don't mean to be a Natzi but, it doesn't matter if the ball is traveling fast. The equation (velocity - 1) is still correct xD. 1 px per frame is just (1 - 1) = 0
Lol, "I don't mean to be a Natzi but," there is no 't' in Nazi.  |
|
|
|
|
 |
Tony
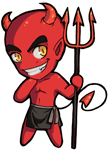
|
|
|
|
 |
Aange10

|
Posted: Mon Jan 02, 2012 3:39 pm Post subject: Re: RE:Help with this Collision Detection |
|
|
Tony @ 2/1/2012, 1:39 pm wrote: Aange10 @ Mon Jan 02, 2012 2:04 pm wrote: it doesn't matter if the ball is traveling fast
It most certainly does http://compsci.ca/v3/viewtopic.php?t=14897
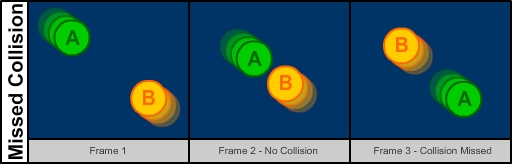
Hmm, I understand that. But I was saying that the equation is still true irrelevant to if it's fast or not.
"The ball can make it up to (velocity - 1) pixels into the block before you do the check for collision"
The ball is 1 px per frame:
"The ball can make it up to 0 pixels into the block before you do the check for collision"
The ball is 20 px per frame
"The ball can make it up to 19 pixels into the block before you do the check for collision"
The senario in the picture is just saying that if (Velocity - 1) > diameter of the ball + diameter of the other object collision could be missed. I.E: Velocity = 100, both balls are 20 px in diameter, 99 > 40, so collision could be missed |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
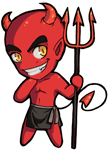
|
Posted: Mon Jan 02, 2012 4:20 pm Post subject: Re: Help with this Collision Detection |
|
|
Aange10 @ Mon Jan 02, 2012 3:39 pm wrote:
"The ball can make it up to 19 pixels into the block before you do the check for collision"
right, which is the problem being described
dffhkhksg @ Sun Jan 01, 2012 5:59 pm wrote:
What is the problem you are having?
Various glitches where the ball passes through a portion of the brick before detecting a collision...
Besides looking odd (bouncing off the insides instead of the edge), a bigger problem is that once the ball is far enough into the block, it now collides at multiple points. If both the left side and the right side of the ball is inside the block, which direction should the ball bounce back in? That depends on implementation details. In OP's case of if-elsif-elsif approach, it will be the first point on some predefined order.
Which could lead to an interesting situation where a fall ball jams itself into brick_one, but the first "collision point" that is found happens to be right at the edge of adjacent brick_two
 |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Aange10

|
Posted: Mon Jan 02, 2012 4:32 pm Post subject: Re: Help with this Collision Detection |
|
|
Tony @ 2/1/2012, 3:20 pm wrote: Aange10 @ Mon Jan 02, 2012 3:39 pm wrote:
"The ball can make it up to 19 pixels into the block before you do the check for collision"
right, which is the problem being described
I understand that x). I was just saying that the equation holds true no matter how fast the ball is going.
Emphasis added:
Tony wrote:
- if the ball is travelling "fast" (anything greater than 1pixel-per-frame really), it can make it up to (velocity - 1) pixels into the block before you do the check for "is the ball colliding right now"
My point was that it doesn't matter if the ball is traveling fast or not, it can always make it up to (velocity - 1) pixels into the block. It just so happens that 1 px velocity adds up to 0 pixels into the block. |
|
|
|
|
 |
Tony
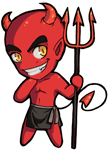
|
Posted: Mon Jan 02, 2012 4:48 pm Post subject: RE:Help with this Collision Detection |
|
|
Right. But it will also make that far into the block when travelling "fast".
Quote:
I used to do drugs. I still do, but I used to, too.
-- Mitch Hedberg
I said if, not iff ( http://en.wikipedia.org/wiki/Iff )  |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Aange10

|
Posted: Mon Jan 02, 2012 5:02 pm Post subject: Re: RE:Help with this Collision Detection |
|
|
Tony @ 2/1/2012, 3:48 pm wrote:
I was clearing that up for the general public.  |
|
|
|
|
 |
chipanpriest

|
Posted: Mon Jan 02, 2012 6:34 pm Post subject: Re: RE:Help with this Collision Detection |
|
|
Tony @ Mon Jan 02, 2012 4:48 pm wrote:
Quote:
I used to do drugs. I still do, but I used to, too.
-- Mitch Hedberg
lol thats amazing haha story of my life :p |
|
|
|
|
 |
|
|