Mouse
Author |
Message |
Unknown25
|
Posted: Thu Dec 29, 2011 1:53 pm Post subject: Mouse |
|
|
What is it you are trying to achieve?
I am trying to make a maze game like this http://www.fugly.com/flash/709/Scary_Maze_Game.html Just not the scary person that comes out after level 3, beware of that if you are going to play it, it will scare you.
So in the game there is no mouse for you to travel but a small box that you have to travel around. I would like to know how do I do that effect? Currently right now my game kinda works with the mouse and if the mouse goes out of the maze then you lose.
What is the problem you are having?
I can't really get it to work and don't know what to put to make it do that.
Describe what you have tried to solve this problem
I tried to add a box to make it move around but can't make it attach to the mouse.
Post any relevant code (You may choose to attach the file instead of posting the code if it is too long)
Please specify what version of Turing you are using
Newest version, whichever that is.
Also another quick question. How do I open a turing file in a turing program? Is it same as for example a text file cause I can't get it to work. Because one file is the main menu for my game and another is one level and wanted to make a separate file for each level to keep it more organized. So how do I open the file in actual turing. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Dreadnought
|
Posted: Thu Dec 29, 2011 4:24 pm Post subject: Re: Mouse |
|
|
Read the documentation on Mouse.Where this will allow you to know the x and y coordinates of the mouse pointer. If you want the box to follow the mouse you want it to have the same x and y coordinates as the mouse all the time.
For your second question include is what you want. |
|
|
|
|
 |
Unknown25
|
Posted: Thu Dec 29, 2011 9:00 pm Post subject: Re: Mouse |
|
|
Dreadnought @ Thu Dec 29, 2011 4:24 pm wrote: Read the documentation on Mouse.Where this will allow you to know the x and y coordinates of the mouse pointer. If you want the box to follow the mouse you want it to have the same x and y coordinates as the mouse all the time.
For your second question include is what you want.
First question: Yes but is there a way I can make the mouse disappear when it comes on the turing page? That way I can connect the square to the same location as mouse and the person can use that. So the mouse is still there but just cannot be seen like the game above. Not sure if it is possible in turing.
second question: Thanks for the include link, I will try it and see how it goes! |
|
|
|
|
 |
Tony
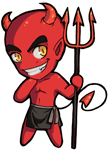
|
Posted: Thu Dec 29, 2011 9:14 pm Post subject: RE:Mouse |
|
|
changing/removing the mouse pointer -- not directly. The operating system handles that, and Turing doesn't have a build-in way to make OS API calls. You could technically wrap those calls in another language and make them executables, runnable with Sys.Exec. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Dreadnought
|
Posted: Fri Dec 30, 2011 12:32 am Post subject: Re: Mouse |
|
|
Like Tony said, there is no way to change/remove the mouse cursor directly in Turing. But here's something that's not too bad.
We can place the Turing window in one corner and move the mouse in a different corner of the screen (assuming the Turing window isn't too big). This way the mouse is not in the Turing window. In my example we "calibrate" the mouse to a different part of the screen. The area the mouse will be moving in is shown by a window. My example uses a simple game I saw used as an example for collision detection somewhere on the forum. My example is set up to allow you to move both the Turing window and the calibration window anywhere on the screen without problem.
Disclaimer: This is somewhat advanced code, I don't recommend using code you can't understand.
Turing: | module MyMouse
import Mouse, Math
export Calibrate, X, Y
var calibrated : boolean := false
% Calibrated x and y coordinates for the mouse
var MouseCalibratedX, MouseCalibratedY : int
% Return the calibrated x coordinate of the mouse
fcn X (mouseX : int) : int
if not calibrated then % Error if not yet calibrated
Error.Halt ("Mouse has not yet been calibrated.")
end if
result mouseX - MouseCalibratedX
end X
% Return the calibrated x coordinate of the mouse
fcn Y (mouseY : int) : int
if not calibrated then % Error if not yet calibrated
Error.Halt ("Mouse has not yet been calibrated.")
end if
result mouseY - MouseCalibratedY
end Y
% Create a new location to be 0,0 for mouse coordinates
proc Calibrate (winID : int)
% Declare Strings (for easy modification)
const MouseString1 := "Mouse Area: This window represents the area to which the mouse will be calibrated."
const MouseString2 := "Place windows in desired position and click on center of target."
% Put the current selected and active window in memory
var SelectedWindow, ActiveWindow : int
SelectedWindow := Window.GetSelect
ActiveWindow := Window.GetActive
Window.Select (winID ) % Select the window we are calibrating for so we can get its size with maxx and maxy
% Prepare a second window
var win2ID, win2Width, win2Height : int
win2Width := maxx
win2Height := maxy
win2ID := Window.Open ("graphics:" + intstr (win2Width ) + ";" + intstr (win2Height ) + ",offscreenonly,position:0;0")
% Print text if the window is big enough (unless your window is really small there should be no problem)
if (win2Width - Font.Width (MouseString1, defFontID )) > 0 and
(win2Width - Font.Width (MouseString2, defFontID )) > 0 and
win2Height > 100 then
locatexy ((win2Width - Font.Width (MouseString1, defFontID )) div 2, win2Height - 100)
put MouseString1
locatexy ((win2Width - Font.Width (MouseString2, defFontID )) div 2, win2Height - 120)
put MouseString2
end if
% Draw "Target" for calibration
Draw.FillOval (win2Width div 2, win2Height div 2, 30, 30, 12)
Draw.Line (win2Width div 2, win2Height div 2 - 10, win2Width div 2, win2Height div 2 + 10, 1)
Draw.Line (win2Width div 2 - 10, win2Height div 2, win2Width div 2 + 10, win2Height div 2, 1)
View.Update % Update Window
% Declare some variables for Mouse.ButtonWait
var mX_, mY_, mBn_, mB_ : int
loop
Mouse.ButtonWait ("down", mX_, mY_, mBn_, mB_ )
Window.Select (win2ID )
exit when Math.Distance (mX_, mY_, win2Width div 2, win2Height div 2) < 5 % Check if the user is within 5 pixels of the center of the target
View.Update
end loop
% Close calibration window and set windows back to the way they were
Window.Close (win2ID )
Window.SetActive (ActiveWindow )
Window.Select (SelectedWindow )
% Set the coordinates that will now be the mouse's 0,0 position
Mouse.Where (mX_,mY_,mB_ )
MouseCalibratedX := mX_ - (win2Width div 2)
MouseCalibratedY := mY_ - (win2Height div 2)
calibrated := true
end Calibrate
end MyMouse
% Now let's create a simple game! (I saw this used as an example for collision detection somwhere on the forum)
var PlayerX, PlayerY, EnemyX, EnemyY : int % Player and Enemy circle
const PlayerRad := 15 % Player Radius
const EnemyRad := 25 % Enemy Radius
var mX, mY, mB : int % Mouse variables
% Simple collision detection function
fcn Collision : boolean
result Math.Distance (PlayerX, PlayerY, EnemyX, EnemyY ) < PlayerRad + EnemyRad
end Collision
% I'm going to make the game window half the screen's size... almost (the borders on the windows and the taskbar screw it up)
const HalfScrnWdth := (Config.Display (cdScreenWidth ) + 1) div 2 % Half the screen's width
const HalfScrnHght := (Config.Display (cdScreenHeight ) + 1) div 2 % Half the screen's height
View.Set ("graphics:" + intstr (HalfScrnWdth ) + ";" + intstr (HalfScrnHght ) + ",offscreenonly,nobuttonbar,position:" + intstr (HalfScrnWdth ) + ";" + intstr (HalfScrnHght ))
% Set initial player position at center
PlayerX := maxx div 2
PlayerY := maxy div 2
% Calibrate mouse
MyMouse.Calibrate (defWinId ) % defWinId is the default window ID
loop
% Create a new enemy
loop
EnemyX := Rand.Int (0, maxx)
EnemyY := Rand.Int (0, maxy)
exit when not Collision
end loop
loop
% Move player circle to the calibrated x and y coordinates
Mouse.Where (mX, mY, mB )
PlayerX := MyMouse.X (mX )
PlayerY := MyMouse.Y (mY )
% Place player at edge of screen if mouse is outside the calibrated area
if PlayerX < PlayerRad then
PlayerX := PlayerRad
elsif PlayerX > maxx - PlayerRad then
PlayerX := maxx - PlayerRad
end if
if PlayerY < PlayerRad then
PlayerY := PlayerRad
elsif PlayerY > maxy - PlayerRad then
PlayerY := maxy - PlayerRad
end if
% Draw player and enemy
cls
put "Press any key to recalibrate the mouse"
Draw.FillOval (PlayerX, PlayerY, PlayerRad, PlayerRad, 12)
Draw.FillOval (EnemyX, EnemyY, EnemyRad, EnemyRad, 1)
View.Update
% If a key is pressed, recalibrate the mouse
if hasch then
MyMouse.Calibrate (defWinId )
Input.Flush % Empty the key buffer
end if
exit when Collision % Create a new enemy on collision
Time.DelaySinceLast (1000 div 60)
end loop
end loop |
PS : Sorry for the wall of code. |
|
|
|
|
 |
Unknown25
|
Posted: Sun Jan 01, 2012 3:49 pm Post subject: RE:Mouse |
|
|
Thanks for your help everyone but I guess I will skip the mouse thing because it is not worth all the trouble at all. It still will work perfectly fine the way it is.
But I still have some trouble with my second question about launching a turing file inside the turing file. I don't want to post the actual code but this is what I did so far: I have an if statement before the game starts to ask the person if they would like music in the game. If the person says yes or no the same file should open but I will add the music code to the yes one. I can't figure out exactly what to do, I tried mostly everything and nothing appears. |
|
|
|
|
 |
Unknown25
|
Posted: Tue Jan 03, 2012 12:46 pm Post subject: RE:Mouse |
|
|
can someone please help me with the include thing/ my second question. I keep trying to do it but nothing appears. |
|
|
|
|
 |
Tony
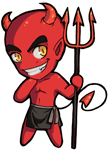
|
Posted: Tue Jan 03, 2012 1:03 pm Post subject: RE:Mouse |
|
|
well that just sounds like a bad idea -- you'll have twice the code, and you'd have to make every change in two places. Very easy to make mistakes.
Why not use the same if-statement to set a variable that remembers the preference, and use that to decide if music should be played or not (all in the same file). |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
Unknown25
|
Posted: Tue Jan 03, 2012 2:23 pm Post subject: Re: RE:Mouse |
|
|
Tony @ Tue Jan 03, 2012 1:03 pm wrote: well that just sounds like a bad idea -- you'll have twice the code, and you'd have to make every change in two places. Very easy to make mistakes.
Why not use the same if-statement to set a variable that remembers the preference, and use that to decide if music should be played or not (all in the same file).
oh I never thought of that, I just got a great idea. Thanks!
But mind you still helping me with opening file inside the turing file because it seems very easy but I can't get it to work. I got it to open text file but not turing file. |
|
|
|
|
 |
Dreadnought
|
Posted: Tue Jan 03, 2012 2:39 pm Post subject: Re: Mouse |
|
|
http://compsci.ca/holtsoft/doc/include.html wrote: An include is used to copy parts of files so that they become part of the Turing program. This copying is temporary, that is, no files are changed. The file name must be an explicit string constant such as "stdstuff".
The copying is temporary so you wont necessarily see anything different.
Example (make sure the files are in the same folder):
First file (I named it "MyExtraFile.t")
Second File (name it whatever you like)
Turing: | put "OMG"
include "MyExtraFile.t" |
When you run the second file, you get
|
|
|
|
|
 |
Unknown25
|
Posted: Tue Jan 03, 2012 2:51 pm Post subject: RE:Mouse |
|
|
oh its that easy? It works thanks a lot! I kept adding lots of other things besides include but I didn't know all I need is include.
I just came with another problem I am wondering if there is a solution to closing the include program after I am done with it. So in my case I have finished the level, then how do I close the level so I can start the next level?
Thanks for your help! |
|
|
|
|
 |
Dreadnought
|
Posted: Tue Jan 03, 2012 3:55 pm Post subject: Re: Mouse |
|
|
You don't "close" the file since it isn't really open. Include allows you to write several small files and have them work as one big file.
When you hit run, Turing compiles the code into stuff that the computer can run. When the compiler finds the line include "filename.t" it finds the file, copies the entire file and replaces the include line with that code. This means that although you have your program written as several small text files, the computer actually only runs one large program. So you are not actually opening the file while running your program.
This means that when my "OMG Hi!" is run, the compiler changes the code into Turing: | put "OMG"
put "Hi!" | and then compiles and runs that.
So you don't have to worry about closing files for include like you do for writing and reading text files. Think of your program as a many pages (your files). The compiler sticks all the pages together and makes a book (the program that is run). |
|
|
|
|
 |
Unknown25
|
Posted: Tue Jan 03, 2012 5:57 pm Post subject: RE:Mouse |
|
|
Oh I misunderstood how it works. So I just noticed that after the program is done, it will just go to the next one which is exactly what I needed, thanks for the help! |
|
|
|
|
 |
|
|