Author |
Message |
varman
|
Posted: Sun Dec 11, 2011 4:54 pm Post subject: Turing and the infinite series |
|
|
What is it you are trying to achieve?
<Replace all the <> with your answers/code and remove the <>>
What is the problem you are having?
I am not able to answer this question. I don't really understand what it wants. Here is the question:
Write a program to find the sum of a number of terms of the
infinite series
1 + x + x**2 + x**3 + x**4 + ...
where the number of terms n to be evaluated and the value of
x are input before the summation begins. Experiment with
different values of n and x.
Describe what you have tried to solve this problem
I tried something but there were like syntax errors every other line.
Please specify what version of Turing you are using
<4.1> |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
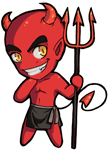
|
Posted: Sun Dec 11, 2011 5:17 pm Post subject: Re: Turing and the infinite series |
|
|
It's asking varman @ Sun Dec 11, 2011 4:54 pm wrote: to find the sum of some numbers.
varman @ Sun Dec 11, 2011 4:54 pm wrote:
I tried something but there were like syntax errors every other line.
Fix the errors, post the code. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
varman
|
Posted: Sun Dec 11, 2011 5:31 pm Post subject: RE:Turing and the infinite series |
|
|
@Tony
Here is some of the code:
var sum: int
for count: 1..n
sum:= sum + x**(count-1)
end for |
|
|
|
|
 |
Tony
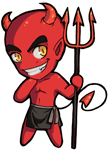
|
Posted: Sun Dec 11, 2011 5:34 pm Post subject: RE:Turing and the infinite series |
|
|
Well, that's a start in the right direction. Now, how about declaring all of the variables used, giving them some values, and making sure that types line up? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
varman
|
Posted: Sun Dec 11, 2011 5:44 pm Post subject: RE:Turing and the infinite series |
|
|
@Tony
I don't know what else I could do. I got the basic idea. Like n is basically the exponent on x and then you need to ask the person for the values. Could you please help me out? |
|
|
|
|
 |
Tony
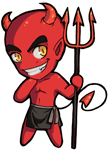
|
Posted: Sun Dec 11, 2011 5:53 pm Post subject: RE:Turing and the infinite series |
|
|
the compiler will tell you what to do next, until the code runs. I would imagine that the first one it will point out is
"variable n has not been declared" |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
varman
|
Posted: Sun Dec 11, 2011 6:05 pm Post subject: RE:Turing and the infinite series |
|
|
@Tony
Yeah, I found that out and forgot to update my code. Here is what I worked out so far:
var sum: int:= 0
var n, x:int
put "Enter the number of terms and then the value of x"
get n, x
for count: 1..n
sum:= sum + x**(count-1)
put sum
end for
How does this look? |
|
|
|
|
 |
Tony
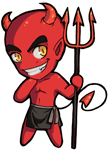
|
Posted: Sun Dec 11, 2011 6:14 pm Post subject: RE:Turing and the infinite series |
|
|
this looks much better
How well does this work for large values of N? (What _is_ a valid large value of N anyway?) |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
varman
|
Posted: Sun Dec 11, 2011 7:48 pm Post subject: RE:Turing and the infinite series |
|
|
It usually says 'overflow in operation' if n is to big. |
|
|
|
|
 |
Tony
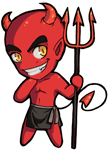
|
Posted: Sun Dec 11, 2011 7:53 pm Post subject: RE:Turing and the infinite series |
|
|
so now you get to learn an excellent lesson in real world software development -- how big is the requirement?
If your solution works for the largest N asked for, you're done. If you need to go higher, it gets much more complicated. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
varman
|
Posted: Sun Dec 11, 2011 8:07 pm Post subject: RE:Turing and the infinite series |
|
|
So how would I get it to go higher? |
|
|
|
|
 |
mirhagk
|
Posted: Sun Dec 11, 2011 8:10 pm Post subject: RE:Turing and the infinite series |
|
|
Isn't n infinity? If it's an infinite series, then n needs to get constantly get bigger. The solution is similar to what you have, but you need to make a couple changes in that case. |
|
|
|
|
 |
Tony
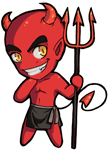
|
|
|
|
 |
varman
|
Posted: Sun Dec 11, 2011 9:47 pm Post subject: RE:Turing and the infinite series |
|
|
Like 9 digits higher. So a number like 999999999 |
|
|
|
|
 |
Dreadnought
|
Posted: Sun Dec 11, 2011 10:28 pm Post subject: Re: Turing and the infinite series |
|
|
The number of terms 'n' on its own is not necessarily the determining factor in whether or not the expression overflows.
If we asked for the sum of the 999,999,999 first terms of 1 + 1**1 + 1**2 + 1**3 + 1**4 .... 1**n (x=1 for your code) the program would run for a long time, but would eventually (a long eventually) output 999,999,999 as the final sum.
Perhaps what is more important to know is: How high do you want the sum (or any term) to go?
If the answer is less than or equal to 2,147,483,647 then your probably fine (except that it might take a long like my example). That value is the largest value you can store in an integer, basically its a 32 bit integer (4 bytes I guess?) with one bit reserved for indicating negative values and with one value subtracted for zero (this means you can actually go one further into the negatives, -2,147,483,648). |
|
|
|
|
 |
|