Operators and Procedures Help
Author |
Message |
TuringBeast
|
Posted: Tue Dec 06, 2011 5:32 pm Post subject: Operators and Procedures Help |
|
|
What is it you are trying to achieve?
I am trying to achieve a problem that I am having in my calculator program.
What is the problem you are having?
Basically, i want to make my code more efficient so what i want to do is assign a value as an operand so it functions just as an operand.
Describe what you have tried to solve this problem
I dont know how to solve the problem, but the only solution i could think of is use separate procedures.
Post any relevant code (You may choose to attach the file instead of posting the code if it is too long)
This is just a segment of my program. Don't mind the coding, it's just the operand signs that I need to fix. As you can see, each of the four procedures are the same, except for the operand signs that have been commented out in the middle of the procedure.
What I want to do is make just one procedure with parameters instead of four separate procedures. I just don't know what i can incorporate in place of those operators and i don't know if I can declare an operator or not.
Turing: |
<proc AddCalc
if FlagDec = false and FlagNeg = false and NumLength > 1 then
if FlagEqual = false then
SaveTemp := SaveTemp div NumPlace
end if
SaveTotal := SaveTotal /*+*/ SaveTemp
elsif FlagDec = false and FlagNeg = true and NumLength > 1 then
if FlagEqual = false then
SaveTemp := SaveTemp div NumPlace
end if
SaveTotal := SaveTotal /*+*/ SaveTemp * (-1)
elsif FlagDec = true and FlagNeg = false and NumLength > 1 then
if FlagEqual = false then
SaveTempDec := SaveTempDec div NumPlace
end if
SaveTotal := SaveTotal /*+*/ SaveTemp + SaveTempDec / (1000000000 div NumPlace)
elsif FlagDec = true and FlagNeg = true and NumLength > 1 then
if FlagEqual = false then
SaveTempDec := SaveTempDec div NumPlace
end if
SaveTotal := SaveTotal /*+*/ (SaveTemp * (-1)) + (SaveTempDec * (-1)) / (1000000000 div NumPlace)
end if
end AddCalc
proc MinusCalc
if FlagDec = false and FlagNeg = false and NumLength > 1 then
if FlagEqual = false then
SaveTemp := SaveTemp div NumPlace
end if
SaveTotal := SaveTotal /*-*/ SaveTemp
elsif FlagDec = false and FlagNeg = true and NumLength > 1 then
if FlagEqual = false then
SaveTemp := SaveTemp div NumPlace
end if
SaveTotal := SaveTotal /*-*/ SaveTemp * (-1)
elsif FlagDec = true and FlagNeg = false and NumLength > 1 then
if FlagEqual = false then
SaveTempDec := SaveTempDec div NumPlace
end if
SaveTotal := SaveTotal /*-*/ SaveTemp + SaveTempDec / (1000000000 div NumPlace)
elsif FlagDec = true and FlagNeg = true and NumLength > 1 then
if FlagEqual = false then
SaveTempDec := SaveTempDec div NumPlace
end if
SaveTotal := SaveTota l/*-*/ (SaveTemp * (-1)) + (SaveTempDec * (-1)) / (1000000000 div NumPlace)
end if
end MinusCalc
proc TimesCalc
if FlagDec = false and FlagNeg = false and NumLength > 1 then
if FlagEqual = false then
SaveTemp := SaveTemp div NumPlace
end if
SaveTotal := SaveTotal /* * */ SaveTemp
elsif FlagDec = false and FlagNeg = true and NumLength > 1 then
if FlagEqual = false then
SaveTemp := SaveTemp div NumPlace
end if
SaveTotal := SaveTotal /* * */ SaveTemp * (-1)
elsif FlagDec = true and FlagNeg = false and NumLength > 1 then
if FlagEqual = false then
SaveTempDec := SaveTempDec div NumPlace
end if
SaveTotal := SaveTotal /* * */ (SaveTemp + SaveTempDec / (1000000000 div NumPlace))
elsif FlagDec = true and FlagNeg = true and NumLength > 1 then
if FlagEqual = false then
SaveTempDec := SaveTempDec div NumPlace
end if
SaveTotal := SaveTotal /* * */ ((SaveTemp * (-1)) + (SaveTempDec * (-1)) / (1000000000 div NumPlace))
end if
end TimesCalc
proc DivCalc
if FlagDec = false and FlagNeg = false and NumLength > 1 then
if FlagEqual = false then
SaveTemp := SaveTemp div NumPlace
end if
SaveTotal := SaveTotal /* / */ SaveTemp
elsif FlagDec = false and FlagNeg = true and NumLength > 1 then
if FlagEqual = false then
SaveTemp := SaveTemp div NumPlace
end if
SaveTotal := SaveTotal /* / */ (SaveTemp * (-1))
elsif FlagDec = true and FlagNeg = false and NumLength > 1 then
if FlagEqual = false then
SaveTempDec := SaveTempDec div NumPlace
end if
SaveTotal := SaveTotal /* / */ (SaveTemp + SaveTempDec / (1000000000 div NumPlace))
elsif FlagDec = true and FlagNeg = true and NumLength > 1 then
if FlagEqual = false then
SaveTempDec := SaveTempDec div NumPlace
end if
SaveTotal := SaveTotal /* / */ ((SaveTemp * (-1)) + (SaveTempDec * (-1)) / (1000000000 div NumPlace))
end if
end DivCalc>
|
Please specify what version of Turing you are using
I am using Turing 4.1.1 |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Insectoid

|
Posted: Tue Dec 06, 2011 5:36 pm Post subject: RE:Operators and Procedures Help |
|
|
There's no nice way to do this in Turing. You're going to need a wall of case/if statements. Basically a lot of "If char = '*' then a *= b". |
|
|
|
|
 |
Tony
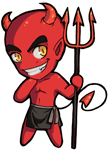
|
Posted: Tue Dec 06, 2011 6:10 pm Post subject: RE:Operators and Procedures Help |
|
|
array of function pointers, using characters in place of an index. Awww yeahhh.
You'd still need a wrapped function for each operator though. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Insectoid

|
Posted: Tue Dec 06, 2011 6:14 pm Post subject: RE:Operators and Procedures Help |
|
|
Does Turing support function pointers? |
|
|
|
|
 |
Dreadnought
|
Posted: Tue Dec 06, 2011 6:17 pm Post subject: Re: Operators and Procedures Help |
|
|
I think Tony means something like this (correct me if I'm wrong).
Turing: |
% Create an array of functions
var ops : array 1 .. 4 of fcn Operator (a, b : real) : real
% Create a binary function for each operator
fcn plus (x, y : real) : real
result x + y
end plus
fcn minus (x, y : real) : real
result x - y
end minus
fcn mult (x, y : real) : real
result x * y
end mult
fcn divide (x, y : real) : real
result x / y
end divide
% set the value of each function in the array to your binary operator functions
ops(1):=plus
ops(2):=minus
ops(3):=mult
ops(4):=divide
% Try them out!
put ops(1)(1,2)
put ops(2)(1,2)
put ops(3)(1,2)
put ops(4)(1,2)
|
|
|
|
|
|
 |
Tony
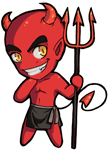
|
|
|
|
 |
|
|