Vigenere Encryptor problems
Author |
Message |
Aange10

|
Posted: Mon Nov 14, 2011 12:26 am Post subject: Vigenere Encryptor problems |
|
|
What is it you are trying to achieve?
A program that will take in text, Caeser cipher it, then Vigen?re cipher it.
What is the problem you are having?
It *looks* like my algorithm for the entire process is correct (which obviously it isnt. I've commented the every step the computer takes in the algorithm, and even gone through it myself with a calculator) but it's [once again obviously] not working.
Hopefully my problem is within a misstype or something >.< I earlier went and found I used Str.Upper where I should have used Str.Lower, which screwed up my ASCII values. But that's all fixed and the problem still persists.
Through my Debugging I've noticed that
1) When DeCrypting the encrypted text, I have it do the Vigen?re cipher first. However, instead of outputting what it should (heh) it outputs the key word I put in. (I've set debugging tools in the program to let me see the text after each encrypting step, and each decrypting step, so I know what it should look like)
Describe what you have tried to solve this problem
I went through and commented the program through every line to follow the algorithm I'm giving the computer. I've went through and followed some of the code with a ASCII chart and a calculator. I've set up various debugging tools throughout the program to give me the information the computer is computing.
I've gone through and made sure the Caeser Cipher Encryption/ Decryption works, and It does. So, it can't be the problem.. It's also a function, and I have no relevant global variables so It's not altering anything like that.
Please specify what version of Turing you are using
4.1
** Yes I did spell Vigen?re like 50 different ways in my program. Haha **
EDIT : The question marks in Vigenere is from the wierd character. http://en.wikipedia.org/wiki/Vigen%C3%A8re_cipher
Description: |
|
 Download |
Filename: |
VinegereEncryption.t |
Filesize: |
14.08 KB |
Downloaded: |
128 Time(s) |
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Aange10

|
Posted: Mon Nov 14, 2011 1:04 am Post subject: RE:Vigenere Encryptor problems |
|
|
ALSO*** I've gone through and made sure the key you are given holds the correct information, and it does.
|
|
|
|
|
 |
Aange10

|
Posted: Mon Nov 14, 2011 2:03 pm Post subject: Re: Vigenere Encryptor problems |
|
|
So, after my third night of working on this, I've found out that my math isn't correct.
If you look at my vinegere cipher when a letter goes in the equation to be encrypted it looks like this
code: |
Letter + (Letter - Vinegere_letter)
90 + (90 - 92)
% Which is = 88 %
|
so if I take the solution, and take it back through the decrypting equation it looks like this
code: |
88 - (88 - 92)
% Which is = 92 %
|
see the problem? ... So I found a solution to that, and it carries over for EVERY equation that I have tested
code: |
88 - ((88 - 92)/2)
% Which is = 90 %
|
Notice how it gives me the correct answer. I tested this on about 7 different problems.
Here's also the equation shown when an odd difference is used, instead of an even
code: |
101 + (101 - 104)
% Which is = 98 %
|
code: |
98 - ((98 - 104)/2)
% Which is = 101 %
|
But if while I'm Encrypting the word needs to wrap, the equation turns into.
code: |
((103 + (103 - 111))+26)
% Which is = 121 %
|
But, when i plug it back in, it uses the equation
code: |
121 - ((121 - 111)/2)
% Which is 116 %
|
The wrap around is screwing it up... But my problem is there is no way I could track everytime it wraps AND input that information into the key, so when it decrypts it knows when to use a different equation.
So my other guess is to take off the wrapping.. but is there a better way?
If it turns a value into one of the [blank] spots on the ASCII chart, will I lose the letter?
Any suggestions are appreciated, and thanks to all who have downloaded and looked over the code with me!
Here is the new code, with the fixed equations.
Description: |
|
 Download |
Filename: |
VinegereEncryption.t |
Filesize: |
14.1 KB |
Downloaded: |
100 Time(s) |
|
|
|
|
|
 |
Aange10

|
Posted: Mon Nov 14, 2011 2:05 pm Post subject: RE:Vigenere Encryptor problems |
|
|
*** Broke his Calculator ***
|
|
|
|
|
 |
Dreadnought
|
Posted: Wed Nov 16, 2011 2:04 am Post subject: Re: Vigenere Encryptor problems |
|
|
Well i was just about to go to bed... and then I saw this. And I had to have a look. lol
The problem is that multiple letters, when encrypted with the same key letter will produce the same output.
Example
Turing: |
121+(121-99) -26 % 'y' encrypted with 'c'
=117 % gives 'u'
% but
108+(108-99) % 'l' (lowercase L) encrypted with 'c'
=117 % also gives 'u'
|
So obviously we have a problem since there is no way, given 117 and 99 ('u' and 'c') to determine whether the original letter was 121 ('y') or 108 ('l').
So I would recommend changing systems.
Vignere ciphers, to my knowledge, shift each letter by an amount equal to the value of the corresponding letter in the key.
Something like
Turing: |
%Encrypt
Cipher_letter = Letter + (Vignere_Letter -97) % Since your alphabet starts at 97 (lowercase) we want to shift by the corresponding amount in alphabet values
% Now check if its in the range 97 to 122 for lowercase letters and subtract 26 accordingly (it shouldn't be smaller than 97)
%Decrypt
Letter = Cipher_letter - (Vignere_letter -97) % Just doing the reverse of encryption, shifting backwards
% now check if the letter is in range and add 26 accordingly (it shouldn't be bigger than 122)
|
You could do something equivalent, and perhaps slightly more elegant, by converting letters to 0 - 25 values then working mod 26 (and convert back to ASCII afterwards).
Alternatively, if you like your system, you could do something like adding or subtracting an odd number (rather than 26 which is even) and then when deciphering, you check if the Cipher_letter and the Vignere_letter have the same pairity. If they don't then you know your added or subtracted when ciphering. But that just seems messy and may not even work (I haven't thought about it).
|
|
|
|
|
 |
Aange10

|
Posted: Fri Nov 18, 2011 6:35 pm Post subject: RE:Vigenere Encryptor problems |
|
|
Thank you very much Dread! My program now functions and is pasted at the bottom of the reply.
I have one question, however. Could you inform me of the equation using mod 26? Insectoid was explaining it to me before, but I did not quite comprehend.
Thanks again!
ALSO: Pretending that you did not know the source code of the program, would this be easy to crack? Considering if one were to try to ceaser crack it, they wouldn't get the correct answer, and if one were to try to vigenere crack it, they would get a ceasered answer (AKA: a bunch of random letter that they would assume not be the answer).
Description: |
|
 Download |
Filename: |
VinegereEncryption.t |
Filesize: |
13.19 KB |
Downloaded: |
98 Time(s) |
|
|
|
|
|
 |
Tony
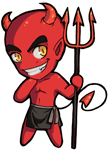
|
Posted: Fri Nov 18, 2011 6:52 pm Post subject: Re: RE:Vigenere Encryptor problems |
|
|
Aange10 @ Fri Nov 18, 2011 6:35 pm wrote: Pretending that you did not know the source code of the program, would this be easy to crack? Considering if one were to try to ceaser crack it, they wouldn't get the correct answer, and if one were to try to vigenere crack it, they would get a ceasered answer (AKA: a bunch of random letter that they would assume not be the answer).
Not knowing the encryption method is never a valid security assumption. Also, Ceaser text is trivially recognizable, as it retains all of the properties of the English plaintext.
Using two weak methods doesn't provide any additional security, beyond the obscurity. A relevant security joke here would be "double rot13 encryption".
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Aange10

|
Posted: Fri Nov 18, 2011 7:19 pm Post subject: RE:Vigenere Encryptor problems |
|
|
I see.
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Dreadnought
|
Posted: Sun Nov 20, 2011 11:02 pm Post subject: Re: Vigenere Encryptor problems |
|
|
Well, I tried it and its cool. Here's some interesting bugs I found on my first couple tries.
Turing: |
% Here's a run of your program
"Please insert your phrase (Maxx 255 characters.)"
MYphraseHERE
"Please give me an interesting word. (Maxx 5 letters)"
a1 % Note the number '1'
"You're new Message! Also saved to [NOT SAVED ANYWHERE]: "
S/9nb*yUKB
% This did not care that I put a number in the key
% Another run
"Please insert your phrase (Maxx 255 characters.)"
BBBBBBB % 7 'B'
"Please give me an interesting word. (Maxx 5 letters)"
i
"You're new Message! Also saved to [NOT SAVED ANYWHERE]: "
P
P
P
P
% Note that every second letter is omitted and replaced by a newline character
|
I could just let you find the problems on your own, and I'm sure you would. But seeing as I found them already, I'll just point you toward them.
The first run shows that you are not actually checking the whole key string. You have an else statement (line 274) that exits the for loop if the first character of the string is a letter.
The second run shows a sneakier bug. It will appear for any key string of less than 5 characters. My example changed letters to newline characters, but others will give non-letter symbols. Heres a short program I wrote to show the problem.
Turing: |
var str : string
put "Give me a string (5 characters):" ..
get str : 5
put skip
put "String: ", str, " - Length: ", length (str )
put skip
put "Here are character values for your string:"
for i: 1.. length(str )
put i, " - char: '" , str (i ), "' - value: ", ord(str (i ))
end for
|
Try this with simple strings. "abcde" first, then "abcd", or anything you want.
What you will find is that the get command will actually put the newline character (from hitting the ENTER key) at the end of the string. The newline character (\n) value is 10. To fix this, you'll need need to add a check for this character in your key checking for loop, and just shorten the string by one character.
But really its a nice program. I like that you put lots of comments, too few people do this.
As for the mod 26 stuff, its modular arithmetic. I wont bore you with all the definitions and other math stuff, but here's the basics of it.
The expression 10 mod 3 produces 1. This is the remainder when dividing 10 by 3 (by definition we say 3*3+1 = 10, so the remainder is 1). The result of applying "mod m" to any integer will produce an integer between 0 and m-1.
10 mod 5 = 0 (because 5*2+0 = 10).
-10 mod 3 = 2 (because 3x-4+2 = -10).
So if we convert all letters in the alphabet to values between 0 and 25, we can then use mod 26 to automatically make sure we don't go out of those bounds. So M + P looks like 13+16 which is 29, but 29 mod 26 is 3 (equivalent to 29-26). In the same way M - P is 13-16 = -3, but -3 mod 26 is 23 (-3+26). Basically what this does is simplify your if statement that checks if you need to add or subtract 26.
You get something like this
Turing: |
for i : start_ .. end_
key := str_lower (key)
% UPPERCASE
if PlainText (i) >= 65 and PlainText (i) <= 90 then
CipherText (i) := 65 + (((PlainText (i) - 65) + (key (i) - 97)) mod 26)
% lowercase
elsif PlainText <= 97 and PlainText (i) <= 122 then
CipherText (i) := 97 + (((PlainText (i) - 97) + (key (i) - 97)) mod 26)
% Other stuff
else
CipherText (i) := PlainText (i)
end if
end for
% Decryption is much the same but we subtact the key value
|
As for the difficulty in cracking this, as Tony said Ceaser ciphers are easy to crack. Only 25 possibilities (shifting by 0 would be too easy).
The vignere cipher is stronger since there is less information that can easily be extracted from the ciphertext but its still relatively easy to crack (there is a reason they don't encrypt government secrets in this way). But unless good money or someone's life is involved its unlikely that anyone would bother trying to crack your text.
|
|
|
|
|
 |
|
|