shifting number in an array
Author |
Message |
sammi
|
Posted: Sat Oct 29, 2011 12:45 pm Post subject: shifting number in an array |
|
|
What is it you are trying to achieve?
<Make the first number 68 and get the rest of the numbers in my partially filled array to shift to the right>
What is the problem you are having?
<Can only get 2 of the number the move and the rest stay the same>
Describe what you have tried to solve this problem
<Answer Here>
Post any relevant code (You may choose to attach the file instead of posting the code if it is too long)
<Answer Here>
Turing: |
% Add the value 68 at the start of the filled section
% shifting everything else to the right
% and adjust variables so it will actually print
%
% Example
% Before: [1][2][3][4]
% After: [68][1][2][3][4]
var numSlots : int
numSlots := Rand.Int(8,14)
var numElements : int
numElements := Rand.Int (3,7)
var nums : array 1 .. numSlots of int
for i : 1 .. numElements
nums (i) := Rand.Int (10, 99)
end for
% Print Array (all on one line)
for i : 1 .. numElements
put "[", nums (i), "]" ..
end for
put "" % so it goes to a new line
% YOUR CODE HERE
var firstSlot : int := nums(1)
var thirdSlot : int := nums (3)
for i : 1 .. numElements
nums (1) := 68
nums (2) := firstSlot
nums (3) := nums (4)
end for
% Print array again
for i : 1 .. numElements
put "[", nums (i), "]" ..
end for
put ""
|
Please specify what version of Turing you are using
<Answer Here> |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Insectoid

|
Posted: Sat Oct 29, 2011 1:04 pm Post subject: RE:shifting number in an array |
|
|
Quote: Can only get 2 of the number the move and the rest stay the same
Maybe if you do this often enough, all of them will have moved. |
|
|
|
|
 |
Tony
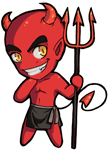
|
Posted: Sat Oct 29, 2011 1:52 pm Post subject: RE:shifting number in an array |
|
|
adding elements to the beginning of an array is incredibly expensive (performance) and complicated (shifting all the other elements). Why would you want to do this?
E.g. you can add the element at the end
code: |
before: [4][3][2][1]
after: [4][3][2][1][68]
|
All the elements preserve the same relative order as in your example, but the insert doesn't require any shifting of elements. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Beastinonyou

|
Posted: Sat Oct 29, 2011 2:28 pm Post subject: Re: shifting number in an array |
|
|
However, If you really need to do it like that, you could use something as simple as this:
Turing: |
var nums : flexible array 1 .. 0 of int
for i : 1 .. 4
new nums, upper (nums ) + 1 % Creates new place in array 'nums'
nums (i ) := i %Rand.Int (10, 99), I'm using 'i' to make it appear how you wanted
end for
% Display the original values in array 'nums'
for i : 1 .. upper (nums )
put "[", nums (i ), "]" ..
end for
put ""
% _____ Pay Attention Below _____
new nums, upper (nums ) + 1 % Create new place in array
for decreasing i : upper (nums ) .. 2 % Go from the end, to 2nd spot
nums (i ) := nums (i - 1) % Value becomes the value previous to it's spot in array
end for
nums (1) := 68 % Change first spot in array to 68, as you wanted
% Display array, which now has 68 in first spot, and the rest of the values after
for i : 1 .. upper (nums )
put "[", nums (i ), "]" ..
end for
|
|
|
|
|
|
 |
sammi
|
Posted: Sat Oct 29, 2011 4:19 pm Post subject: RE:shifting number in an array |
|
|
now im getting some error message that says "variable has no value" but i declared it above so i dont know what this means
Turing: |
% Add the value 68 at the start of the filled section
% shifting everything else to the right
% and adjust variables so it will actually print
%
% Example
% Before: [1][2][3][4]
% After: [68][1][2][3][4]
var numSlots : int
numSlots := Rand.Int (8, 14)
var numElements : int
numElements := Rand.Int (3, 7)
var nums : flexible array 1 .. numSlots of int
for i : 1 .. 4
new nums, upper (nums ) + 1 % Creates new place in array 'nums'
nums (i ) := i % Using 'i' to make it appear how I wanted
end for
% Display the original values in array 'nums'
for i : 1 .. upper (nums )
put "[", nums (i ), "]" ..
end for
put ""
% YOUR CODE HERE
new nums, upper (nums ) + 1 % Create new place in array
for decreasing i : upper (nums ) .. 2 % Go from the end, to 2nd spot
nums (i ) := nums (i - 1) % Value becomes the value previous to it's spot in array
end for
nums (1) := 68 % Change first spot in array to 68
% Display array, which now has 68 in first spot, and the rest of the values after
for i : 1 .. upper (nums )
put "[", nums (i ), "]" ..
end for
% Print array again
for i : 1 .. numElements
put "[", nums (i ), "]" ..
end for
put ""
|
|
|
|
|
|
 |
Insectoid

|
Posted: Sat Oct 29, 2011 4:44 pm Post subject: RE:shifting number in an array |
|
|
"variable has no value" means you're trying to do something with a variable that has no value yet. When you declare a variable it doesn't yet have a value.
This won't execute. What is i? How can I add to i, if I don't know what it is?
code: | var i : int
i := 0
i++ |
Now we know that i is 0, so we can add to it no problem.
Now, in your code you've created an array of random length between 8 and 14. So 'upper' is at least 8. You then go on to execute a for loop 4 times, filling in the first 4 values in the array and making the array 4 spaces bigger. The array is now between 12 and 18 slots in length.
Then, you iterate over the entire array and try to output the value at each index in the array. But...we have at least 12 elements, and we only assigned 4 values. That leaves six elements with no value, that you are trying to print. And there, sir, is your error. |
|
|
|
|
 |
sammi
|
Posted: Sat Oct 29, 2011 4:53 pm Post subject: RE:shifting number in an array |
|
|
so how would i input that into my code.....???? |
|
|
|
|
 |
Beastinonyou

|
Posted: Sat Oct 29, 2011 5:08 pm Post subject: Re: shifting number in an array |
|
|
This:
Turing: |
var nums : flexible array 1 .. numSlots of int
|
Is your Problem
You're starting your array with an upper bound of 8 to 12. Then later, you're adding 4 more.
You need to start with 0.
Turing: |
var nums : flexible array 1 .. 0 of int
|
And also, Why are you printing the array a third time?
Turing: |
% Print array again
for i : 1 .. numElements
put "[", nums (i), "]" ..
end for
put ""
|
fix your flexible array bounds, and run it. You'll see what I mean by the output window. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
evildaddy911
|
Posted: Mon Oct 31, 2011 3:07 pm Post subject: Re: shifting number in an array |
|
|
heres a way that i figures out, you just need to have the computer know what each element is:
Turing: | var aray : array 1 .. 5 of int
for i : 1 .. 5
aray (i) := i
end for
for j : 1 .. 5
put aray (j)
end for
put ""
for decreasing k : 5 .. 2
aray (k) := aray (k - 1)
put aray (k)
end for |
then you figure out what the new aray(1) is and your all set |
|
|
|
|
 |
|
|