Exiting loops and going back to start
Author |
Message |
bwong
|
Posted: Wed Jun 15, 2011 2:58 pm Post subject: Exiting loops and going back to start |
|
|
What is it you are trying to achieve?
My issue comes at the ending of the quiz portion of my program. Once the user is done the quiz, I want the program to go back to the main page (start of program).
What is the problem you are having?
I do not know how to do this. I was told to make a large loop, and smaller loops for each section. I tried this, but it did not work. I also tried a more far-fetched idea which included using GUI.ShowWindow, and GUI.HideWindow, which not as much success.
Post any relevant code (You may choose to attach the file instead of posting the code if it is too long)
Turing: |
%%%%%%%%%%%%%%%%%%%%%%FORMATTING%%%%%%%%%%%%%%%%%%%%%%
import GUI
setscreen ("graphics: 800; 600")
%Question Header Box will be 640 * 044 pixels
%Question Answer Box will be 640 * 225 pixels
%Information Headers will be 640 * 044 pixels
%Information bodies will be 640 * 445 pixels
%%%%%%%%%%%%%%%%%VARIABLE DECLARATION%%%%%%%%%%%%%%%%%
var mousex, mousey, mousesel, mousedown : int
var fadecolour : int := 000
var userselection : string := ""
var picBlended : int
%IMAGE VARIABLES: The image ID is used for organization.
%Sample: pic(usage: char (f (formating), q (quiz), i (info), t (titles)), number: int)
var picf01 : int := Pic.FileNew ("picf001_mainpage.jpg")
var picf02 : int := Pic.FileNew ("picf002_quizpage.jpg")
var picf03 : int := Pic.FileNew ("picf003_infopage.jpg")
var picf04 : int := Pic.FileNew ("picf04_testcomplete.jpg")
var picq01 : int := Pic.FileNew ("picq001_question1_q1.jpg")
var picq02 : int := Pic.FileNew ("picq002_question1_answer1.jpg")
var picq03 : int := Pic.FileNew ("picq003_question1_q2.jpg")
var picq04 : int := Pic.FileNew ("picq004_question1_answer2.jpg")
var picq05 : int := Pic.FileNew ("picq005_question1_q3.jpg")
var picq06 : int := Pic.FileNew ("picq006_question1_answer3.jpg")
var picq07 : int := Pic.FileNew ("picq007_question1_q4.jpg")
var picq08 : int := Pic.FileNew ("picq008_question1_answer4.jpg")
var picq09 : int := Pic.FileNew ("picq009_question1_q5.jpg")
var picq10 : int := Pic.FileNew ("picq010_question1_answer5.jpg")
var picq_highlight : int := Pic.FileNew ("picq_highlight.jpg")
var pici01 : int := Pic.FileNew ("pici001_information_Blackholes.jpg")
var pici02 : int := Pic.FileNew ("pici002_information_Nebula.jpg")
var pici03 : int := Pic.FileNew ("pici003_information_Stars.jpg")
var pici04 : int := Pic.FileNew ("pici004_information_Galaxies.jpg")
var pici05 : int := Pic.FileNew ("pici005_information_Planets.jpg")
var pict01 : int := Pic.FileNew ("pict001_information_Blackholes.jpg")
var pict02 : int := Pic.FileNew ("pict002_information_Nebula.jpg")
var pict03 : int := Pic.FileNew ("pict003_information_Stars.jpg")
var pict04 : int := Pic.FileNew ("pict004_information_Galaxies.jpg")
var pict05 : int := Pic.FileNew ("pict005_information_Planets.jpg")
%End image variables
var font001 : int := Font.New ("MyriadPro-Cond:72")
var font002 : int := Font.New ("MyriadPro-Cond:32")
var q1n : int := 0
var q2n : int := 0
var q3n : int := 0
var q4n : int := 0
var q5n : int := 0
var Answer : array 1 .. 5 of int
var animatebutton : int
var resultsbutton : int
var quizbutton : int
var infobutton : int
var quitbutton : int
%%%%%%%%%%%%%%%%%%%%%%PROCEDURES%%%%%%%%%%%%%%%%%%%%%%
procedure donothing
end donothing
procedure introduction
Pic.DrawSpecial (picf01, 000, 000, picCopy, picFadeIn, 750)
for i : 0 .. 255
RGB.SetColor (fadecolour, i / 255, i / 255, i / 255)
Font.Draw ("Astronomy", 020, 500, font001, fadecolour )
View.Update
delay (05)
end for
animatebutton := GUI.CreateButton (010, 040, 140, "Animation", donothing )
resultsbutton := GUI.CreateButton (490, 040, 140, "Results", donothing )
quizbutton := GUI.CreateButton (170, 040, 140, "Quiz", donothing )
infobutton := GUI.CreateButton (330, 040, 140, "Information", donothing )
quitbutton := GUI.CreateButton (650, 040, 140, "Exit", donothing )
end introduction
procedure test (questionhead, questionansw : int, answer : int)
Pic.Draw (picf02, 000, 000, picCopy)
Pic.DrawSpecial (questionhead, 080, 525, picCopy, picBlend + 85, 000)
Pic.DrawSpecial (questionansw, 080, 200, picCopy, picBlend + 080, 000)
loop
Mouse.Where (mousex, mousey, mousedown )
Pic.DrawSpecial (questionansw, 080, 200, picCopy, picBlend + 04, 000)
if mousex >= 085 and mousex <= 715 and mousey >= 330 and mousey <= 365
then
Pic.DrawSpecial (picq_highlight, 085, 330, picCopy, picBlend + 4, 000)
if mousedown = 1 then
Answer (answer ) := 1
exit
end if
elsif mousex >= 085 and mousex <= 715 and mousey >= 290 and mousey <= 325
then
Pic.DrawSpecial (picq_highlight, 085, 290, picCopy, picBlend + 4, 000)
if mousedown = 1 then
Answer (answer ) := 2
exit
end if
elsif mousex >= 085 and mousex <= 715 and mousey >= 255 and mousey <= 290
then
Pic.DrawSpecial (picq_highlight, 085, 250, picCopy, picBlend + 4, 000)
if mousedown = 1 then
Answer (answer ) := 3
exit
end if
end if
end loop
delay (175)
end test
%%%%%%%%%%%%%%%%%%%%MAIN PROGRAMME%%%%%%%%%%%%%%%%%%%%
loop
introduction
loop
Mouse.Where (mousex, mousey, mousedown )
if mousedown = 1
then
if mousex >= 010 and mousey >= 040 and mousex <= 149 and mousey <= 062
then
userselection := "animation"
exit
elsif mousex >= 170 and mousey >= 040 and mousex <= 319 and mousey <= 062
then
userselection := "quiz"
exit
elsif mousex >= 330 and mousey >= 040 and mousex <= 479 and mousey <= 062
then
userselection := "info"
exit
elsif mousex >= 490 and mousey >= 040 and mousex <= 639 and mousey <= 062
then
userselection := "results"
exit
elsif mousex >= 650 and mousey >= 040 and mousey <= 789 and mousey <= 062
then
userselection := "exit"
exit
end if
end if
end loop
if mousedown = 1
then
if userselection = "animation"
then
loop
GUI.Hide (animatebutton )
put "Under Construction"
end loop
elsif userselection = "quiz"
then
loop
GUI.Hide (quizbutton )
cls
Pic.Draw (picf02, 000, 000, picCopy)
test (picq01, picq02, 1)
test (picq03, picq04, 2)
test (picq05, picq06, 3)
test (picq07, picq08, 4)
test (picq09, picq10, 5)
Pic.DrawSpecial (picf04, 025, 025, picCopy, picGrowCenterToEdgeNoBar, 750)
delay (1250)
exit
end loop
elsif userselection = "info"
then
loop
GUI.Hide (infobutton )
cls
Pic.Draw (picf03, 000, 000, picCopy)
for i : 0 .. 255
RGB.SetColor (fadecolour, i / 255, i / 255, i / 255)
Font.Draw ("Space Frontiers", 020, 500, font001, fadecolour )
View.Update
delay (05)
end for
var galaxybutton : int := GUI.CreateButton (010, 040, 140, "Galaxies", donothing )
var planetbutton : int := GUI.CreateButton (170, 040, 140, "Planets", donothing )
var nebulabutton : int := GUI.CreateButton (330, 040, 140, "Nebula", donothing )
var starbutton : int := GUI.CreateButton (490, 040, 140, "Stars", donothing )
var blackbutton : int := GUI.CreateButton (650, 040, 140, "Black Holes", donothing )
end loop
end if
end if
loop
exit when GUI.ProcessEvent
end loop
end loop
|
Please specify what version of Turing you are using
Turing 4.1.1
-------------------------------------UPDATE---------------------------------------------
This was my attempt using GUI.HideWindow, and GUI.ShowWindow
Turing: |
%%%%%%%%%%%%%%%%%%%%%%FORMATTING%%%%%%%%%%%%%%%%%%%%%%
import GUI
setscreen ("graphics: 800; 600")
%Question Header Box will be 640 * 044 pixels
%Question Answer Box will be 640 * 225 pixels
%Information Headers will be 640 * 044 pixels
%Information bodies will be 640 * 445 pixels
%%%%%%%%%%%%%%%%%VARIABLE DECLARATION%%%%%%%%%%%%%%%%%
var mousex, mousey, mousesel, mousedown : int
var fadecolour : int := 000
var userselection : string := ""
var picBlended : int
%IMAGE VARIABLES: The image ID is used for organization.
%Sample: pic(usage: char (f (formating), q (quiz), i (info), t (titles)), number: int)
var picf01 : int := Pic.FileNew ("picf001_mainpage.jpg")
var picf02 : int := Pic.FileNew ("picf002_quizpage.jpg")
var picf03 : int := Pic.FileNew ("picf003_infopage.jpg")
var picf04 : int := Pic.FileNew ("picf04_testcomplete.jpg")
var picq01 : int := Pic.FileNew ("picq001_question1_q1.jpg")
var picq02 : int := Pic.FileNew ("picq002_question1_answer1.jpg")
var picq03 : int := Pic.FileNew ("picq003_question1_q2.jpg")
var picq04 : int := Pic.FileNew ("picq004_question1_answer2.jpg")
var picq05 : int := Pic.FileNew ("picq005_question1_q3.jpg")
var picq06 : int := Pic.FileNew ("picq006_question1_answer3.jpg")
var picq07 : int := Pic.FileNew ("picq007_question1_q4.jpg")
var picq08 : int := Pic.FileNew ("picq008_question1_answer4.jpg")
var picq09 : int := Pic.FileNew ("picq009_question1_q5.jpg")
var picq10 : int := Pic.FileNew ("picq010_question1_answer5.jpg")
var picq_highlight : int := Pic.FileNew ("picq_highlight.jpg")
var pici01 : int := Pic.FileNew ("pici001_information_Blackholes.jpg")
var pici02 : int := Pic.FileNew ("pici002_information_Nebula.jpg")
var pici03 : int := Pic.FileNew ("pici003_information_Stars.jpg")
var pici04 : int := Pic.FileNew ("pici004_information_Galaxies.jpg")
var pici05 : int := Pic.FileNew ("pici005_information_Planets.jpg")
var pict01 : int := Pic.FileNew ("pict001_information_Blackholes.jpg")
var pict02 : int := Pic.FileNew ("pict002_information_Nebula.jpg")
var pict03 : int := Pic.FileNew ("pict003_information_Stars.jpg")
var pict04 : int := Pic.FileNew ("pict004_information_Galaxies.jpg")
var pict05 : int := Pic.FileNew ("pict005_information_Planets.jpg")
%End image variables
var font001 : int := Font.New ("MyriadPro-Cond:72")
var font002 : int := Font.New ("MyriadPro-Cond:32")
var q1n : int := 0
var q2n : int := 0
var q3n : int := 0
var q4n : int := 0
var q5n : int := 0
var Answer : array 1 .. 5 of int
var newwindow : int
var animatebutton : int
var resultsbutton : int
var quizbutton : int
var infobutton : int
var quitbutton : int
%%%%%%%%%%%%%%%%%%%%%%PROCEDURES%%%%%%%%%%%%%%%%%%%%%%
procedure restart
GUI.HideWindow (defWinID )
GUI.ShowWindow (newwindow )
end restart
procedure donothing
end donothing
procedure introduction
Pic.DrawSpecial (picf01, 000, 000, picCopy, picFadeIn, 750)
for i : 0 .. 255
RGB.SetColor (fadecolour, i / 255, i / 255, i / 255)
Font.Draw ("Astronomy", 020, 500, font001, fadecolour )
View.Update
delay (05)
end for
animatebutton := GUI.CreateButton (010, 040, 140, "Animation", donothing )
resultsbutton := GUI.CreateButton (490, 040, 140, "Results", donothing )
quizbutton := GUI.CreateButton (170, 040, 140, "Quiz", donothing )
infobutton := GUI.CreateButton (330, 040, 140, "Information", donothing )
quitbutton := GUI.CreateButton (650, 040, 140, "Exit", donothing )
end introduction
procedure test (questionhead, questionansw : int, answer : int)
Pic.Draw (picf02, 000, 000, picCopy)
Pic.DrawSpecial (questionhead, 080, 525, picCopy, picBlend + 85, 000)
Pic.DrawSpecial (questionansw, 080, 200, picCopy, picBlend + 080, 000)
loop
Mouse.Where (mousex, mousey, mousedown )
Pic.DrawSpecial (questionansw, 080, 200, picCopy, picBlend + 04, 000)
if mousex >= 085 and mousex <= 715 and mousey >= 330 and mousey <= 365
then
Pic.DrawSpecial (picq_highlight, 085, 330, picCopy, picBlend + 4, 000)
if mousedown = 1 then
Answer (answer ) := 1
exit
end if
elsif mousex >= 085 and mousex <= 715 and mousey >= 290 and mousey <= 325
then
Pic.DrawSpecial (picq_highlight, 085, 290, picCopy, picBlend + 4, 000)
if mousedown = 1 then
Answer (answer ) := 2
exit
end if
elsif mousex >= 085 and mousex <= 715 and mousey >= 255 and mousey <= 290
then
Pic.DrawSpecial (picq_highlight, 085, 250, picCopy, picBlend + 4, 000)
if mousedown = 1 then
Answer (answer ) := 3
exit
end if
end if
end loop
delay (175)
end test
%%%%%%%%%%%%%%%%%%%%MAIN PROGRAMME%%%%%%%%%%%%%%%%%%%%
procedure mainprogramme
loop
introduction
loop
Mouse.Where (mousex, mousey, mousedown )
if mousedown = 1
then
if mousex >= 010 and mousey >= 040 and mousex <= 149 and mousey <= 062
then
userselection := "animation"
exit
elsif mousex >= 170 and mousey >= 040 and mousex <= 319 and mousey <= 062
then
userselection := "quiz"
exit
elsif mousex >= 330 and mousey >= 040 and mousex <= 479 and mousey <= 062
then
userselection := "info"
exit
elsif mousex >= 490 and mousey >= 040 and mousex <= 639 and mousey <= 062
then
userselection := "results"
exit
elsif mousex >= 650 and mousey >= 040 and mousey <= 789 and mousey <= 062
then
userselection := "exit"
exit
end if
end if
end loop
if mousedown = 1
then
if userselection = "animation"
then
loop
GUI.Hide (animatebutton )
put "Under Construction"
end loop
elsif userselection = "quiz"
then
loop
GUI.Hide (quizbutton )
cls
Pic.Draw (picf02, 000, 000, picCopy)
test (picq01, picq02, 1)
test (picq03, picq04, 2)
test (picq05, picq06, 3)
test (picq07, picq08, 4)
test (picq09, picq10, 5)
Pic.DrawSpecial (picf04, 025, 025, picCopy, picGrowCenterToEdgeNoBar, 750)
delay (1250)
restart
end loop
elsif userselection = "info"
then
loop
GUI.Hide (infobutton )
cls
Pic.Draw (picf03, 000, 000, picCopy)
for i : 0 .. 255
RGB.SetColor (fadecolour, i / 255, i / 255, i / 255)
Font.Draw ("Space Frontiers", 020, 500, font001, fadecolour )
View.Update
delay (05)
end for
var galaxybutton : int := GUI.CreateButton (010, 040, 140, "Galaxies", donothing )
var planetbutton : int := GUI.CreateButton (170, 040, 140, "Planets", donothing )
var nebulabutton : int := GUI.CreateButton (330, 040, 140, "Nebula", donothing )
var starbutton : int := GUI.CreateButton (490, 040, 140, "Stars", donothing )
var blackbutton : int := GUI.CreateButton (650, 040, 140, "Black Holes", donothing )
end loop
end if
end if
loop
exit when GUI.ProcessEvent
end loop
end loop
end mainprogramme
mainprogramme
newwindow := Window.Open ("title:ICS Culminating,graphics:800;600,position:top;right")
mainprogramme
Window.Hide (newwindow )
|
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
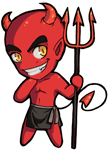
|
Posted: Wed Jun 15, 2011 3:17 pm Post subject: Re: Exiting loops and going back to start |
|
|
bwong @ Wed Jun 15, 2011 2:58 pm wrote: I was told to make a large loop, and smaller loops for each section.
That is how it's typically done. You'd need to remember to reset all of the state information to how it was before the game starts; mostly importantly you'd probably need GUI.ResetQuit (read the documentation on how it works). |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
bwong
|
Posted: Wed Jun 15, 2011 6:32 pm Post subject: Re: Exiting loops and going back to start |
|
|
Understanding what you said sir, how should I alter the code to make it run properly? |
|
|
|
|
 |
ProgrammingFun

|
Posted: Wed Jun 15, 2011 6:36 pm Post subject: RE:Exiting loops and going back to start |
|
|
This looks strangely like the culminating for ICS at VPCI.
However, back on topic:
You could do the loop way or you can just call back to the mainprogram procedure when an exit input is received (or the exit procedure if there is one).
Using the loop method, you would have everything in a nested loop and you would just exit the internal loop when the exit command is recieved. |
|
|
|
|
 |
bwong
|
Posted: Wed Jun 15, 2011 10:19 pm Post subject: Re: RE:Exiting loops and going back to start |
|
|
ProgrammingFun @ Wed Jun 15, 2011 6:36 pm wrote: This looks strangely like the culminating for ICS at VPCI.
It is...... lol.
i solved the issue. I should have put the large end loop before the
|
|
|
|
|
 |
Raknarg

|
Posted: Wed Jun 15, 2011 11:06 pm Post subject: RE:Exiting loops and going back to start |
|
|
or... you could put the whole game in a second loop? That's how I typically repeat my games. |
|
|
|
|
 |
|
|