Problem with View.Update
Author |
Message |
Krol23
|
Posted: Wed Jun 08, 2011 11:00 am Post subject: Problem with View.Update |
|
|
What is the problem you are having?
While attempting to make frogger, I have run into a problem at the beginning with extreme flashing. Everthing flashes when i run the program. Please note that my I am just learning to use what.colour so that piece of code is irrelevant (just testing for now). If I get rid of some cls, the flashing gets better but leaves a trail behind my images. Please tell me if I'm usnig View.Update properly.
Describe what you have tried to solve this problem
I've tried adding more View.Upadates in different locations and getting rid of cls.
Post any relevant code (You may choose to attach the file instead of posting the code if it is too long)
Here is all my code for now.
Turing: |
var pic1 : int := Pic.FileNew ("car.gif")
var pic2 : int := Pic.FileNew ("car.gif")
var pic3 : int := Pic.FileNew ("car.gif")
var xcounter, ycounter : int := 0
var chars : array char of boolean
var frogpic : int := Pic.FileNew ("frog_edited.jpg")
var background : int := Pic.FileNew ("untitled.bmp")
var xfield : int := 1
var yfield : int := 1
View.Set ("graphics:800;600")
View.Set ("offscreenonly")
colourback (255)
process frog
loop
Input.KeyDown (chars )
if chars (KEY_RIGHT_ARROW) then
if whatdotcolour (xfield + 20, yfield + 20) = 255 then
exit
else
xcounter := xcounter + 2
end if
elsif chars (KEY_UP_ARROW) then
if whatdotcolour (xfield + 20, yfield + 20) = 255 then
exit
else
ycounter := ycounter + 2
end if
elsif chars (KEY_LEFT_ARROW) then
xcounter := xcounter - 2
elsif chars (KEY_DOWN_ARROW) then
ycounter := ycounter - 2
end if
cls
Pic.Draw (frogpic, xcounter, ycounter, picCopy)
View.Update
end loop
end frog
loop
fork frog
cls
for x : - 20 .. 640
Pic.Draw (background, 14, 11, picMerge)
View.Update
Pic.Draw (pic1, x, 340, picMerge)
View.Update
Pic.Draw (pic2, x + 40, 200, picMerge)
View.Update
Pic.Draw (pic3, x - 200, 200, picMerge)
View.Update
end for
end loop
|
Please specify what version of Turing you are using
Version 4.1.1 |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
DemonWasp
|
Posted: Wed Jun 08, 2011 11:19 am Post subject: RE:Problem with View.Update |
|
|
You have two major problems that are causing the flashing. The first is that you should only use ONE View.Update() per "frame" of your program. The idea is as follows:
1. Figure out where everything is.
2. Draw everything there.
3. View.Update()
4. Go to step 1.
The second problem you have is that you're abusing process, which means that you don't have any control over where things go and when. The first, and only, rule about processes in Turing is: do not use processes. They are ridiculously unsafe, and only useful for a couple of tasks, and even then they're generally more trouble than they're worth.
To avoid using processes, you need to switch your thinking from this:
"A car and a frog are moving at the same time."
to this:
"A car and a frog each move a little bit, then draw in their new positions. Then they do it again, really fast."
This leads you to the standard animation setup:
code: |
loop
% read user input here (Input.KeyDown, Mouse.Where)
% act on user input: set speed variables, change position variables
% draw all things in their new positions
View.Update()
end loop
|
This setup lets multiple objects appear to move "simultaneously", by updating fast enough and smoothly enough to fool our eyes into thinking they are actually moving independently. |
|
|
|
|
 |
Raknarg

|
Posted: Wed Jun 08, 2011 1:33 pm Post subject: RE:Problem with View.Update |
|
|
Im just going to explain a bit about View.Update.
So basically the reason you use View.Update is to make everything easier for the computer. Instead of having to draw every object you tell it to, it only calculates the coordinates (aka: offscreenonly, it does everything offscreen). Once it hits View.Update, it draws everything based on the coordinates it has already. That's why you DONT use more than one View.Update unless there's a specific reason to do so, because it's the same as what it was having to do before.
Just a bit of information, in case your wondering why with all the view.updates it's still slowing down. |
|
|
|
|
 |
Tony
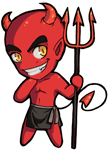
|
Posted: Wed Jun 08, 2011 1:48 pm Post subject: RE:Problem with View.Update |
|
|
More precisely, the technique is called double buffering. Here's an illustrative example:
- you draw box1 to screen
- you draw box2 to screen
Updating the screen takes some amount of time. In that time there exists a version of the screen that has box1 but not yet box2 -- this is perceived as "flashing" (especially if you also draw a blank screen and display that).
In "double buffering" both boxes are first written to memory ("buffer" / "offscreen") and then the entire frame is drawn to the screen just once (View.Update). This avoids intermediate frames with blank flashing spots.
DemonWasp has already pointed out, but this is worth repeating: multiple View.Updates break their own purpose, and use of processes breaks use of View.Update. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
|
|