Passing array info into a procedure... again
Author |
Message |
Lucas

|
Posted: Mon Mar 28, 2011 7:11 pm Post subject: Passing array info into a procedure... again |
|
|
What is it you are trying to achieve?
Im trying to pass the information of an array to a procedure. The array is part of a record, and theres a problem when i declare what part of the record im trying to pass to the procedure
What is the problem you are having?
I get -'points' cannot be followed by a '.'- and it highlights the line:
calcthetaR (points.x, points.z)
specifically highliting the '.' after points
Turing: |
% variables for points
type pointsdata :
record
x, y, z, X, Y, Z, t, r : real
dx, dy : int
dix, diy : real
selected : boolean
end record
var points : flexible array 0 .. - 1 of pointsdata
var numpoints : int := - 1
procedure calcthetaR (A, B : array 0 .. * of real)
for a : 0 .. numpoints
if A (a ) > 0 and B (a ) > 0 then
points (a ).t := arctand (A (a ) / B (a ))
elsif A (a ) > 0 and B (a ) < 1 then
points (a ).t := arctand (-B (a ) / A (a )) + 90
elsif A (a ) < 1 and B (a ) < 1 then
points (a ).t := arctand (-A (a ) / -B (a )) + 180
else
points (a ).t := arctand (B (a ) / -A (a )) + 270
end if
points (a ).r := sqrt (A (a ) ** 2 + B (a ) ** 2)
end for
end calcthetaR
calcthetaR (points.x, points.z )
|
Please specify what version of Turing you are using
4.1.1 |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
chrisbrown

|
Posted: Mon Mar 28, 2011 7:29 pm Post subject: RE:Passing array info into a procedure... again |
|
|
points is an array, not a record. You would have to manually aggregate all the x and y fields into new arrays. However, since points is global, you can remove A and B altogether and simply access the fields directly. A(a) is the same as points(a).x.
edit: And if you're just trying to eliminate duplicate code, just create variables at the beginning of the for loop storing the current x and y. |
|
|
|
|
 |
Lucas

|
Posted: Mon Mar 28, 2011 8:47 pm Post subject: RE:Passing array info into a procedure... again |
|
|
K thx, is there anyway to make this work though? cause the reason i used A and B instead of points(a).x and points(a).z is because i do exactly the same thing three diff combos of points (a). x/y/z. possible or not? |
|
|
|
|
 |
Tony
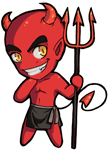
|
Posted: Mon Mar 28, 2011 8:49 pm Post subject: RE:Passing array info into a procedure... again |
|
|
Instead of an array of records, you could have a record of arrays. Of course that would make adding/removing individual elements a lot more complicated, but that's the tradeoff. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
chrisbrown

|
Posted: Mon Mar 28, 2011 9:30 pm Post subject: Re: Passing array info into a procedure... again |
|
|
Well, there's the way you want to hear, and there's the better way.
The short-term fix is to create two new arrays, let's call them A and B. For each element in points, assign the corresponding elements in A and B to the values of x and z respectively, for that particular entry. When finished, call calcthetaR(A, B).
The better way is to modify calctheta (drop the R) so that it returns the arctand of two real numbers. That way, you can do something like:
Turing: | for i ...
points(i).t := calcthetha(points(i).x, points(i).z)
points(i).r := ...
end for |
|
|
|
|
|
 |
Lucas

|
Posted: Tue Mar 29, 2011 8:18 pm Post subject: RE:Passing array info into a procedure... again |
|
|
@Tony: K thx, i might do that next time, but if i did that now id have to modify my whole code, so i dont think ill do it this time
@ chrisbrown: I thought about ur second idea, but it results in more lines of code, so i did it the way i did. and wouln't i just run into the same problem as before?
also, its called calcthetaR is cause theres a bit in there where calculates the radius |
|
|
|
|
 |
chrisbrown

|
Posted: Wed Mar 30, 2011 1:35 am Post subject: Re: RE:Passing array info into a procedure... again |
|
|
You say you run this calculation over the combinations of x, y and z? How are you saving the values of t and r each time? If you run it for x, y and then y, z, then t and r have been overwritten.
Assuming you have a way to get around this (or I'm just missing something), here's a more complete sketch of what I'm thinking:
Turing: | function calctheta(a, b : real) : real
% code to calculate points(i).t but use the result keyword instead of :=
end
function calcdistance(a, b : real) : real
% you already have this line somewhere, it just needs some tweaks
end
for i ...
% Three different combos. I've invented record fields to avoid overwriting data
points(i).t_xy := calctheta(...) % x, y
points(i).r_xy := calcdistance(...)
points(i).t_xz := calctheta(...) % x, z
points(i).r_xz := calcdistance(...)
points(i).t_yz := calctheta(...) % y, z
points(i).r_yz := calcdistance(...)
end for |
It may seem repetitive but it's much easier on the eyes than needless temporary arrays. You can look at that and immediately understand what's happening. |
|
|
|
|
 |
Lucas

|
Posted: Wed Mar 30, 2011 6:04 pm Post subject: RE:Passing array info into a procedure... again |
|
|
@chrisbrown: |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Lucas

|
Posted: Wed Mar 30, 2011 6:08 pm Post subject: RE:Passing array info into a procedure... again |
|
|
sry, accidentally hit the enter button a couple of times :/
thx, ill do it ur way then :p
and the reason i only have one variable for t and r is because i only ever need one r and t value at a time, i nvr actualy need seperate ones. just the way im doing it, i reset the t and r variables evrytime the user inputs the direction they want to spin the object. Im sure theres a better way to do this, i just do it this way because its the way that i figured out myself :p |
|
|
|
|
 |
|
|