Creating a zone on a sprite?
Author |
Message |
TheCatsMeow
|
Posted: Thu Jan 06, 2011 3:46 pm Post subject: Creating a zone on a sprite? |
|
|
What is it you are trying to achieve?
What i'm trying to achieve here is, making a ball bounce off a paddle.. I am trying to recreate pong
What is the problem you are having?
I used a sprite to create my paddles in my game of pong, they work fine they don't go past the barriers i set for them, they stay within the limits. The only problem i am having, is actually making the ball bounce off the paddles
Describe what you have tried to solve this problem
I tried putting the ball to not go within the x or y of the sprite, but then i realised that, that didn't work because my x and y variables are made to set the position of the sprite.
Post any relevant code (You may choose to attach the file instead of posting the code if it is too long)
%Programmer: Trevor Khan
%Date: Jan 5 2010
%Program: Pong main game
%**********************************************************************************************************
%What i'm trying to create here is the left paddle to move up and down
%I'm also going to test making barriors for it
%**********************************************************************************************************
var win : int %declaring window variable
var lpaddle, lpaddlex, lpaddley : int %This is the variable that holds the left "paddle" sprite
var rpaddle, rpaddlex, rpaddley : int %This is the variable that holds the right "paddle" sprite
var chars : array char of boolean %This holds the characters variable
var key : boolean %This will be my Flag variable
var clr1, clr2, clr3, clr4, clr5, clr6, clr7, clr8 : int %These are the barrior variables for the left side
var clr9, clr10, clr11, clr12, clr13, clr14, clr15, clr16 : int %These are the barrior variables for the right side
var ball1, ball2, ball3, ball4, ball5, ball6, ball7, ball8 : int %These will be the barriers for the balls
var ballx, bally : int %The balls x and y variables
var changex, changey : int %The balls placement variables
var dirx, diry : int %The balls direction variables
%**********************************************************************************************************
%Creating the window
% -graphics 1268;956 (Comp. Sci. labs regular computer monitor size) NOTE: This is only for my test program
win := Window.Open ("graphics:1268;956")
%**********************************************************************************************************
%Initializing section
lpaddlex := 50 %left paddle x
lpaddley := 375 %left paddle y
rpaddlex := 1185 %right paddle x
rpaddley := 375 %right paddle y
ballx := 650 %Ball coordinants
bally := 450
changex := 3 %This controls how the ball changes its place on the coordinants grid
changey := 2
dirx := 1 %This controls the balls direction, wheter it's left or right, or up or down
diry := 1
%**********************************************************************************************************
Pic.ScreenLoad ("PongGame.bmp", 0, 0, picMerge) %This is the background image
%**********************************************************************************************************
%Now i'm gonna make the sprite move when its pressed by the keys W and S
%The w key will make the sprite move up and the s key will make it move down
lpaddle := Sprite.New (Pic.FileNew ("Paddle.bmp")) %The width is 17, and the length is 140
rpaddle := Sprite.New (Pic.FileNew ("Paddle.bmp")) %The width is 17, and the length is 140
%=========================================================================================================
process leftpaddle %This is going to be the process for the player one, or the left player
loop
clr1 := whatdotcolour (lpaddlex - 2, lpaddley - 2) %bottom left
clr2 := whatdotcolour (lpaddlex - 2, lpaddley + 70) %middle left
clr3 := whatdotcolour (lpaddlex - 2, lpaddley + 142) %top lef
clr4 := whatdotcolour (lpaddlex + 10, lpaddley - 2) %bottom middle
clr5 := whatdotcolour (lpaddlex + 10, lpaddley + 142) %top middle
clr6 := whatdotcolour (lpaddlex + 19, lpaddley - 2) %bottom right
clr7 := whatdotcolour (lpaddlex + 19, lpaddley + 70) %middle right
clr8 := whatdotcolour (lpaddlex + 19, lpaddley + 142) %top right
Input.KeyDown (chars)
key := false %reset key to false when loop restarts so it only moves if a key is pressed
if chars ('w') and (clr3 not= 10) and (clr5 not= 10) and (clr8 not= 10) then
%up
key := true
lpaddley := lpaddley + 1
Sprite.Show (lpaddle)
Sprite.SetPosition (lpaddle, lpaddlex, lpaddley, false)
delay (5)
elsif chars ('s') and (clr1 not= 10) and (clr4 not= 10) and (clr6 not= 10) then
%down
key := true
lpaddley := lpaddley - 1
Sprite.Show (lpaddle)
Sprite.SetPosition (lpaddle, lpaddlex, lpaddley, false)
delay (5)
else
Sprite.SetPosition (lpaddle, lpaddlex, lpaddley, false)
Sprite.Show (lpaddle)
delay (5)
end if
end loop
end leftpaddle
%=========================================================================================================
process rightpaddle %This is going to be the process for the player 2, or the right player
loop
clr9 := whatdotcolour (rpaddlex - 2, rpaddley - 2) %bottom left
clr10 := whatdotcolour (rpaddlex - 2, rpaddley + 70) %middle left
clr11 := whatdotcolour (rpaddlex - 2, rpaddley + 142) %top lef
clr12 := whatdotcolour (rpaddlex + 10, rpaddley - 2) %bottom middle
clr13 := whatdotcolour (rpaddlex + 10, rpaddley + 142) %top middle
clr14 := whatdotcolour (rpaddlex + 19, rpaddley - 2) %bottom right
clr15 := whatdotcolour (rpaddlex + 19, rpaddley + 70) %middle right
clr16 := whatdotcolour (rpaddlex + 19, rpaddley + 142) %top right
Input.KeyDown (chars)
key := false %reset key to false when loop restarts so it only moves if a key is pressed
if chars (KEY_UP_ARROW) and (clr11 not= 10) and (clr13 not= 10) and (clr16 not= 10) then
rpaddley := rpaddley + 1
Sprite.Show (rpaddle)
Sprite.SetPosition (rpaddle, rpaddlex, rpaddley, false)
delay (5)
elsif chars (KEY_DOWN_ARROW) and (clr9 not= 10) and (clr12 not= 10) and (clr14 not= 10) then
%down
rpaddley := rpaddley - 1
Sprite.Show (rpaddle)
Sprite.SetPosition (rpaddle, rpaddlex, rpaddley, false)
delay (5)
else
Sprite.Show (rpaddle)
Sprite.SetPosition (rpaddle, rpaddlex, rpaddley, false)
delay (5)
end if
end loop
end rightpaddle
%**********************************************************************************************************
process ball
loop
Draw.Oval (ballx, bally, 5, 5, red)
delay (20)
ballx := ballx + changex * dirx
bally := bally + changey * diry
if (ballx >= maxx - 10) or (ballx <= 10) then
dirx := dirx * -1
end if
if (bally >= maxy - 310) or (bally <= 240) then
diry := diry * -1
end if
end loop
end ball
%**********************************************************************************************************
%Forking the processes to work
fork leftpaddle
fork rightpaddle
fork ball
%**********************************************************************************************************
(Sorry if this code is to long, i thought it should be alright it's only around 164 lines of code, added that there are many of my dividers)
Turing: |
<Add your code here>
|
Please specify what version of Turing you are using
i am using 4.1.1 |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
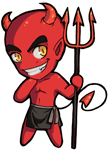
|
Posted: Thu Jan 06, 2011 3:49 pm Post subject: RE:Creating a zone on a sprite? |
|
|
How are you making the paddles not go off screen? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
TheCatsMeow
|
Posted: Thu Jan 06, 2011 3:52 pm Post subject: RE:Creating a zone on a sprite? |
|
|
What i did was, i used a technique that makes it so.. when my sprites touch a certain colour, they stop.
clr9 := whatdotcolour (rpaddlex - 2, rpaddley - 2) %bottom left
clr10 := whatdotcolour (rpaddlex - 2, rpaddley + 70) %middle left
clr11 := whatdotcolour (rpaddlex - 2, rpaddley + 142) %top lef
clr12 := whatdotcolour (rpaddlex + 10, rpaddley - 2) %bottom middle
clr13 := whatdotcolour (rpaddlex + 10, rpaddley + 142) %top middle
clr14 := whatdotcolour (rpaddlex + 19, rpaddley - 2) %bottom right
clr15 := whatdotcolour (rpaddlex + 19, rpaddley + 70) %middle right
clr16 := whatdotcolour (rpaddlex + 19, rpaddley + 142) %top right
What that does right there, is a create a little barrier around my paddles. and in a little down, it says if so and so not = to 10, then...
what that means is to continue unless it's on the colour 49 |
|
|
|
|
 |
Tony
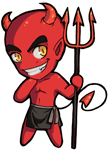
|
Posted: Thu Jan 06, 2011 4:04 pm Post subject: RE:Creating a zone on a sprite? |
|
|
Uhh.... you would need a lot of points to do the same for a ball. Have you considered using Math? It should be fairly simple to figure out if a circle intersects with a line. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
TheCatsMeow
|
Posted: Thu Jan 06, 2011 4:08 pm Post subject: RE:Creating a zone on a sprite? |
|
|
Well yeah i guess haha.
I guess what i'm really trying to ask is, what kind of code would i have to write to make a sort of... zone around my sprite. So, if i say that the ball touchs that zone, it will deflect.
and i'm talking zone like when your creating a zone for your menu. When your mouse goes over the letters and the letters turn yellow or something. But in this case, it makes the ball deflect |
|
|
|
|
 |
Tony
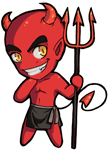
|
Posted: Thu Jan 06, 2011 4:25 pm Post subject: RE:Creating a zone on a sprite? |
|
|
You check if a geometric shape representing a paddle and a geometric shape representing a ball overlap (that is, there exists a point that is inside of both) |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
TheCatsMeow
|
Posted: Thu Jan 06, 2011 5:56 pm Post subject: RE:Creating a zone on a sprite? |
|
|
Hmmm, alright. how would i do that? |
|
|
|
|
 |
Tony
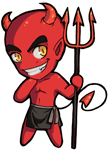
|
Posted: Thu Jan 06, 2011 6:06 pm Post subject: RE:Creating a zone on a sprite? |
|
|
collision detection
Rectangle vs. Circle is somewhere on the second page, but it would probably help to start out with simpler shape combinations first. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
TheCatsMeow
|
Posted: Thu Jan 06, 2011 8:05 pm Post subject: Re: RE:Creating a zone on a sprite? |
|
|
Tony @ Thu Jan 06, 2011 6:06 pm wrote: collision detection
it would probably help to start out with simpler shape combinations first.
Agreed. But since i'm doing this as a final project for my Comp. Sci. class, i decided i wanted to do something that was a little over my head.. Maybe it'd help me learn more about Turing  |
|
|
|
|
 |
Tony
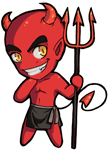
|
Posted: Thu Jan 06, 2011 8:19 pm Post subject: RE:Creating a zone on a sprite? |
|
|
If you want an extra challenge, you can try implementing pong in 20 or less lines of (Turing) code. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
TheCatsMeow
|
Posted: Sun Jan 09, 2011 12:56 pm Post subject: Re: Creating a zone on a sprite? |
|
|
Oh my, that sure would be hard. i mean, the way i've been taught, is really a more extended way of doing things. But, is there anywhere on the forums i could look for maybe already finished games? I just want to study how they did their ball bounce. i've studied other peoples programs, but their not commented enough. |
|
|
|
|
 |
Tony
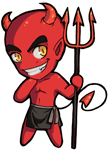
|
Posted: Sun Jan 09, 2011 2:46 pm Post subject: RE:Creating a zone on a sprite? |
|
|
Technically yes, but then it becomes way to tempting to copy that and other parts. Perhaps subconsciously.
Typical ball bounces are implemented as simply reversing the horizontal direction (if the ball was flying left, it is now flying right). This will be sufficient for a working game. If you have time after, you could experiment with other approaches; but the goal for right now is to get every part working in a reasonable way. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
RandomLetters
|
Posted: Sun Jan 09, 2011 6:07 pm Post subject: RE:Creating a zone on a sprite? |
|
|
The simplest collision would be with the walls
Here, all you would need is something like
if ballX > maxX
For colliding with paddles, you would need to check that the coordinates of the ball is within the coordinates of the rectangle. Then there is collision.
if ballX is between minX and maxX and ballY is between minY and maxY
To inverse direction you can use vectors. But for something like pong where all the surfaces are vertical or horizontal, you simply need to invert the horizontal/vertical direction, as Tony said. |
|
|
|
|
 |
TheCatsMeow
|
Posted: Mon Jan 10, 2011 2:18 pm Post subject: RE:Creating a zone on a sprite? |
|
|
Alright. i get it now, the only problem i'm haing a problem is is making the ball actually work. i need to re write the ball part of the code, thats probably my problem. I'll try changing that part of the code.. |
|
|
|
|
 |
|
|