Revisiting your code.
Author |
Message |
Zren

|
Posted: Wed Dec 29, 2010 9:20 pm Post subject: Revisiting your code. |
|
|
Before I lose you in my reveling and disgust. I'm wondering how many other's out there (most likely all) have stories of true coding horror. Has there ever been a time that you look back at your code and shudder in fear. If you have, do tell (and give the gist of the err just in case).
~~~~~~~~~~~~~~
So. I pulled up my final project from grade 11 again. I've recently dabbled into Java thanks to a superly mindlessly awesome game and it's rather open moding of it on the server side (client too but that's wishy washy in the legalities). Anyways, that's not what this is about.
I pulled out this again: http://compsci.ca/v3/viewtopic.php?t=18598&highlight=polywars
For reference, here's a compiled version in a runnable jar: http://dl.dropbox.com/u/5094161/d/Polywars.zip
All I can say is that I'm shamefaced. The end result was superly awesome for 2 eleventh graders (or so I thought), but the sheer idiocy of the code. >.<'
A brief introduction to the game. It's a turn based battle on a 2D grid. There are a few tile types, but most are anesthetic. There are also neutral sheep (the CREATURES variable you'll see later) that move around the map creating randomness. You can change maps from preset to randomness and also edit them ingame. This is a project I made alongside another in my grade. We'd both agree I was a better code-ninja (though he'd argue about being the better ninja in general). Anyways...
Now why it sucks.
Let's start with this:
Java: |
static class Player {
String[] name = new String[PLAYER_ 1+PLAYER_ 2+CREATURES ];
int[] HP = new int[PLAYER_ 1+PLAYER_ 2+CREATURES ];
int[] str = new int[PLAYER_ 1+PLAYER_ 2+CREATURES ];
int[] def = new int[PLAYER_ 1+PLAYER_ 2+CREATURES ];
int[] moveRange = new int[PLAYER_ 1+PLAYER_ 2+CREATURES ];
int[] attackRange = new int[PLAYER_ 1+PLAYER_ 2+CREATURES ];
int[] type = new int[PLAYER_ 1+PLAYER_ 2+CREATURES ];
int[] acc = new int[PLAYER_ 1+PLAYER_ 2+CREATURES ];
int[] dodge = new int[PLAYER_ 1+PLAYER_ 2+CREATURES ];
boolean[] doneMove = new boolean[PLAYER_ 1+PLAYER_ 2+CREATURES ];
boolean[] doneAttack = new boolean[PLAYER_ 1+PLAYER_ 2+CREATURES ];
public void define (int i, String n, int h, int s, int d, int mR, int aR, int a, int dod, int t ) {
name [i ] = n;
HP [i ] = h;
str [i ] = s;
def [i ] = d;
moveRange [i ] = mR;
attackRange [i ] = aR;
type [i ] = t;
acc [i ] = a;
dodge [i ] = dod;
}
}
static Player unit = new Player ();
|
What? Did I really just make a class just to store, well, everything? God dammit. At least I learned a valuable lesson on finding a specific spot of memory in a memory that can store multiple amounts of data (memory at the lowest end). Albeit that probably wasn't my intention at the time. To do it better:
Java: |
class Game {
ArrayList<Player> players; // add both players + neutral into list
ArrayList<Unit> units;
Map map;
int turn;
}
class Map {
String name;
int[][] data;
public boolean load (File filepath ) {
//read file
}
public boolean save (File filepath ) {
//save to file
}
}
class Player {
ArrayList<Unit> units;
String name;
public Player (String name ) {
this. name = name;
//initialize
}
//getters and setters + list control
}
class Unit {
Player owner;
int HP, str, def, acc, dodge
moveRange, attackRange,
type,
x, y;
boolean doneMove, doneAttack;
public Unit (Player owner ) {
this. owner = owner;
//initialize
}
//getters and setters
//turn reset function
}
|
* So much static o.O. Mind you this was solved by putting more stuff in other classes above.
* The random maps have a tendency to trap units. Would require sweeping all walkable tiles after seeding the map to check if they are connected to another walkable tile.
* Redrawing everything every frame. Sure there small animation that changes every frame, but targeting out those tiles would be better. I slightly remember my teacher mentioning this. Mind you, I would of made a crap ton of functions instead of breaking it into objects with a similar function (probably a good reason to use an interface heh). So the gain would of been even worse.
*GUI is hardcoded. Menu's could probably have been an object.
I should also probably mention that each of the unit types are hardcoded vector drawings. That's why the download has no image files. We attempted at trying to blit images and failed easily. So we stuck to what we knew (we both knew images from Turing, but it wasn't that easy this time). So, bad.
I'll be picking at this a bit more for my failures. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Insectoid

|
Posted: Wed Dec 29, 2010 11:19 pm Post subject: RE:Revisiting your code. |
|
|
I tried to make Pacman in grade 10. While pretty cool at the time, it fails miserably now.
I tried to do pathfinding AI but of course did not succeed even remotely. I also tried to develop my own sorting algorithm, which was hilariously bad (More of a hard-code-every-case thing than a real algorithm). I'm too scared to actually look at the code, but I know it's really, really bad. |
|
|
|
|
 |
DtY

|
Posted: Wed Dec 29, 2010 11:34 pm Post subject: Re: RE:Revisiting your code. |
|
|
My favourite line: "int[] str = new int[PLAYER_1+PLAYER_2+CREATURES]; "
I'm sure "int str" made perfect sense at the time
Also, what are PLAYER_1, PLAYER_2 and CREATURES?
Insectoid @ Wed Dec 29, 2010 11:19 pm wrote: I tried to make Pacman in grade 10. While pretty cool at the time, it fails miserably now.
I tried to do pathfinding AI but of course did not succeed even remotely. I also tried to develop my own sorting algorithm, which was hilariously bad (More of a hard-code-every-case thing than a real algorithm). I'm too scared to actually look at the code, but I know it's really, really bad. Speaking of Pacman, you'll want to read this if you ever do it again (or you might just want to read it). |
|
|
|
|
 |
Insectoid

|
Posted: Wed Dec 29, 2010 11:39 pm Post subject: RE:Revisiting your code. |
|
|
I did read about that when I was making the game actually. I never got around to actually implementing the ghosts, but I DID want to get their 'personalities' right. |
|
|
|
|
 |
TerranceN
|
Posted: Thu Dec 30, 2010 2:46 am Post subject: Re: Revisiting your code. |
|
|
Lets see...
First of all, lets start with my grade 10 computer science class (I was in grade 9 though). We were told to make a spaceship game with specific requirements. Our teacher did not teach us functions, so I found myself repeating/copypasta-ing the same code and just changing a number, or having sections of code copy and pasted throughout the code. And yes, I had to make changes, multiple times. And yes, I learned my lesson. Only at the end of the course, and on my own time did I find how to use subroutines.
In grade 10, first semester (before my computer science class that year, and in my own time), before we were taught trigonometry in math class, I used the cosine law to find angles because I read that it could find angles online. Here is the function I used for angles, where opposite is the vector (I thought vectors were some 'space' separate from Cartesian space at the time, lol) from one point to another, and the two 'sides' as I called them, were the components. Also note # means float
code: |
Function Angle_To_180# (opposite#,leg1#,leg2#)
a# = ACos ((Square(leg1) + Square(leg2) - Square(opposite))/(2*leg1*leg2))
Return a#
End Function
|
Other things when I used Blitz3D included anonymous objects with global list for all of them, this obviously led to really confusing syntax. (I don't quite remember how the syntax works, but I think player.player allows you to specify a specific object when you create it or when its in a loop of all objects, and I believe they are all added to some global list for that type of object automatically.
code: |
player.player = New player
player\xcenter = screen_width/2
player\ycenter = screen_height/2
player\waitframes = 2
player\bps = 5
|
Also, there was no inheritance/polymorphism, so I would add any attributes specific only to a few of a certain type to all of that type. And just to make it worse, I was really lazy with variable names. Resulting in code like this
code: |
Type enemy
Field xcenter
Field ycenter
Field xoffset
Field yoffset
Field xstrech
Field ystrech
Field dir
Field angle#
Field aangle#
Field gotoangle#
Field p1x
Field p1y
Field p2x
Field p2y
Field p3x
Field p3y
Field p4x
Field p4y
Field p5x
Field p5y
Field lowx
Field highx
Field lowy
Field highy
Field name$
Field shield
Field shieldstartx
Field shieldstarty
Field shieldendx
Field shieldendy
Field shieldm#
Field shieldb#
Field radius
Field mx#
Field my#
Field scale#
Field cx#
Field cy#
End Type
|
Next came the Turing era in my programming history.
This was my first real attempt to learn objects, and to make a geometry wars clone. Unfortunately I didn't know that classes could not have circular dependence, which my whole design relied on, so I eventually gave up trying to refactor my code into a workable design.
Then came my attempts at making 3d projection, which at the time absolutely blew my mind. Once I made a pseudo 3d projection, I tried to do rotations, which blew my mind even further. Up to this point I always knew rotations as x = cos(A) * r, y = sin(A) * r, so how could I rotate something in 3d?
Nothing really notable happened until the next computer science class, where I made a Geometry Wars clone, the one I posted here, where I did not know what the heap and stack were, and was used to garbage collection in Java. As I have stated in the thread for my game, it has a constant memory leak (the memory for enemies is never deleted).
When I look back at it I really stumbled to the point where I am today, with no real direction from anyone else. I never had a skilled computer science teacher, and I never had a coding buddy, so my code has been left the way it is, cause I never knew it was wrong. At least I am a good independent coder (as in I know how to use Google, a surprising number of people I know do not), and had a long enough time to realize many of my mistakes. So cheers for the internet  |
|
|
|
|
 |
Tony
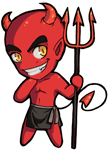
|
|
|
|
 |
Helldemon80
|
Posted: Thu Dec 30, 2010 2:38 pm Post subject: Re: Revisiting your code. |
|
|
LMAO, revisiting my code is a nightmare.
I recently looked back at my gr 11 comp sci summative. I made a Legend of Zelda game, but never finished. All i had was the maps running and the attacking functions with some horrible enemy AI. I wanted to maybe try and fix it. But i honestly am so confused looking at my code. It's so messy and there's no way I can fix it. Ill just leave it there for funny memories.
That is the only sort of full game I ever made. RPG's are a nightmare. |
|
|
|
|
 |
Dan
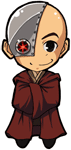
|
Posted: Thu Dec 30, 2010 7:38 pm Post subject: Re: Revisiting your code. |
|
|
Zren @ 29th December 2010, 9:20 pm wrote: Before I lose you in my reveling and disgust. I'm wondering how many other's out there (most likely all) have stories of true coding horror. Has there ever been a time that you look back at your code and shudder in fear. If you have, do tell (and give the gist of the err just in case).
V3..... |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
Sponsor Sponsor

|
|
 |
ProgrammingFun

|
Posted: Thu Dec 30, 2010 9:31 pm Post subject: Re: Revisiting your code. |
|
|
Dan @ Thu Dec 30, 2010 7:38 pm wrote: V3.....
is V3 that bad? Off-topic but: what did V1 and V2 look like?
-------------------------------------------------------------------------------------------------------------------------
My example of terrible and messy code is here: http://compsci.ca/v3/viewtopic.php?t=23967
This was a project we had to do last year after only half a semester of learning Turing (the rest was comprised for Computer Engineering and HTML)...my school drops Turing after that...we are now doing Java + Computer Engineering. |
|
|
|
|
 |
jcollins1991
|
|
|
|
 |
ProgrammingFun

|
Posted: Fri Dec 31, 2010 9:14 am Post subject: RE:Revisiting your code. |
|
|
lol, was V1 an integration of the forum and the blog?
I must say, V3 looks much better  |
|
|
|
|
 |
Zren

|
Posted: Mon Jan 03, 2011 11:03 pm Post subject: Re: RE:Revisiting your code. |
|
|
DtY @ Wed Dec 29, 2010 11:34 pm wrote: My favourite line: "int[] str = new int[PLAYER_1+PLAYER_2+CREATURES]; "
I'm sure "int str" made perfect sense at the time
Also, what are PLAYER_1, PLAYER_2 and CREATURES?
Total sense man D:
and those constants are the amount of units to their respective groups. Once again, hardcoded ungodlyness.
and after reading that Pacman article, I totally want to make it now lol, but meh.
@TerranceN:
Terrible naming schemes are evil. Soon as I learned about camel case and word_spacing-ness I mixed around a little. Then I started reading here and noticed how most of the senior coders wrote code like a prose, or as damn near elegant enough for human speech as possible.
@"as in I know how to use Google, a surprising number of people I know do not"
Oh google, where would I be without you. Probably more social. :/
@Tony:
http://www.w3schools.com/browsers/browsers_stats.asp
At the time, "other" only had round a 14% market share. Mostly Mozilla fans. Plus your failure in grasping the web culture has nothing to do with your code. No you don't win.
@Helldemon80 "my first game was an RPG"
>.<'
@Dan: "v3"
xD If it ain't broke (stare's at Turing forum post error) .... much, don't fix it right.
@ProgrammingFun:
Wow that game was well put together. Never mind everything was hardcoded. The animations were spiffy and anything with sound always make's it 200x better.
v2 seems less homier than v1 :/
v3 is slowly feeling more and more like I'm using the Wayback machine though (far as the net goes) (phpBB ha!). There's so much jQuery in some of the new bb software. o.O But at least some skins don't feel like your probably using tables in the layout...
Oh, and when 3D goes horribly, horribly wrong. This is part of my Virus game. I faintly remember trying to recreate it in PyGame and unterly failing at trying to understand what was going through my mind when doing the math for this. I think this falls under "Does it work ? Make a program on it : Add a few more variables...".
Turing: |
Draw.Line (
ORIGIN_X + round (c_z * ((c_z * x1 + c_x ) / z1 ) + c_x + x1 ),
ORIGIN_Y + round (c_z * ((c_z * y1 + c_y ) / z1 ) + c_y + y1 ),
ORIGIN_X + round (c_z * ((c_z * x2 + c_x ) / z2 ) + c_x + x2 ),
ORIGIN_Y + round (c_z * ((c_z * y2 + c_y ) / z2 ) + c_y + y2 ),
c )
|
X is multiplied by the camera zoom. Shifted by the camera x. Divided by z. Shifted by camera and x (o.O' ...). Then multiplied by camera zoom.
The effect was it would zoom into the center of the screen to create pseudo perspective. |
|
|
|
|
 |
|
|