Help with LED please.
Author |
Message |
Lucky1
|
Posted: Fri Dec 31, 2010 1:50 pm Post subject: Help with LED please. |
|
|
What is it you are trying to achieve?
Create a program that randomly generates numbers to light up ONE of the four LEDs. Generate four random flashes where only one LED lights up at a time. Each flash must be separated by a quarter second pause.
What is the problem you are having?
I totaly don't understand when it means generate a number and suppose to light up each one?
Describe what you have tried to solve this problem
I got some of the code but im really lost. So do I need an if statement? or something? So I know I need to serpate each flash by quater sec but create four random flashes by generating 4 random numbers? Does that mean it has to be binary and the highest will by only 15?
Post any relevant code (You may choose to attach the file instead of posting the code if it is too long)
Turing: |
var num1: int
var num2: int
var num3: int
var num4: int
randint (num, 1, 15)
randint (num2, 1, 15)
randint (num3, 1, 15)
randint (num4, 1, 15)
put "Random Number ", num
put ""
put "Random Number ", num2
put ""
put "Random Number ", num3
put ""
put "Random Number ", num4
put ""
loop
parallelput (1)
delay (250)
parallelput (2)
delay (500)
parallelput (4)
delay (750)
parallelput (8)
delay (1000)
end loop
|
Please specify what version of Turing you are using
<Answer Here> |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
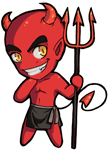
|
Posted: Fri Dec 31, 2010 1:54 pm Post subject: RE:Help with LED please. |
|
|
Sounds like the problem is with the interpretation of the question, rather than anything technical. Your teacher would be the best resource to clarify what the intention of the assignment was.
My personal guess is that you randomly pick which one of four lights you turn on. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Lucky1
|
Posted: Fri Dec 31, 2010 2:27 pm Post subject: RE:Help with LED please. |
|
|
So I would need an if statements? |
|
|
|
|
 |
TokenHerbz

|
Posted: Fri Dec 31, 2010 2:30 pm Post subject: RE:Help with LED please. |
|
|
almost all programs require it... |
|
|
|
|
 |
TerranceN
|
Posted: Fri Dec 31, 2010 2:50 pm Post subject: RE:Help with LED please. |
|
|
Since there are only four possible LEDs, you only need to get 4 different numbers from Rand.Int, then just use if statements to say 'if I got a number 1 from Rand.Int then turn on the first LED', and so on.
Another way, assuming the four parallelput statements you wrote are how to turn on each LED, you can see a pattern in the number you send to parallelput, they are all powers of 2. I'll leave the rest for you to figure out. |
|
|
|
|
 |
Lucky1
|
Posted: Fri Dec 31, 2010 2:58 pm Post subject: Re: RE:Help with LED please. |
|
|
TerranceN @ Fri Dec 31, 2010 2:50 pm wrote: Since there are only four possible LEDs, you only need to get 4 different numbers from Rand.Int, then just use if statements to say 'if I got a number 1 from Rand.Int then turn on the first LED', and so on.
Another way, assuming the four parallelput statements you wrote are how to turn on each LED, you can see a pattern in the number you send to parallelput, they are all powers of 2. I'll leave the rest for you to figure out.
For the " if statements if num2= 2 then parallelput (2) " ? That the problem I'm having how would I turn it on with an if statment
So for my binary/parallelput it should be 1-8. |
|
|
|
|
 |
TerranceN
|
Posted: Fri Dec 31, 2010 3:08 pm Post subject: RE:Help with LED please. |
|
|
The random number does not have to correspond to the number that goes into parallelput.
So for the first random number:
Turing: |
if (num1 = 1) then
% Turn on LED 1
elsif (num1 = 2) then
% Turn on LED 2
elsif (num1 = 3) then
% Turn on LED 3
elsif (num1 = 4) then
% Turn on LED 4
end if
|
You would just replace each comment with how you turn on that LED. |
|
|
|
|
 |
Dragon20942

|
Posted: Sat Jan 01, 2011 3:16 pm Post subject: RE:Help with LED please. |
|
|
I suppose you need a counted loop that performs a random flash each time through the loop.
Use RNG to determine which LED flashes in tandem Terrance's if statement.
I don't quite understand; are you using Turing to actually light up real LEDs? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Lucky1
|
Posted: Sun Jan 02, 2011 1:39 pm Post subject: Re: Help with LED please. |
|
|
TerranceN @ Fri Dec 31, 2010 3:08 pm wrote: The random number does not have to correspond to the number that goes into parallelput.
So for the first random number:
Turing: |
if (num1 = 1) then
% Turn on LED 1
elsif (num1 = 2) then
% Turn on LED 2
elsif (num1 = 3) then
% Turn on LED 3
elsif (num1 = 4) then
% Turn on LED 4
end if
|
You would just replace each comment with how you turn on that LED.
Would this be before the loop? What do you mean replace how to turn on LED? What do the brackets do?
Dragon20942 @ Sat Jan 01, 2011 3:16 pm wrote: I suppose you need a counted loop that performs a random flash each time through the loop.
Use RNG to determine which LED flashes in tandem Terrance's if statement.
I don't quite understand; are you using Turing to actually light up real LEDs?
Using a breadboard connected D-Sub Cable to the computer. The LED in the breadboard |
|
|
|
|
 |
Tony
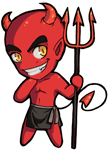
|
Posted: Sun Jan 02, 2011 2:58 pm Post subject: Re: Help with LED please. |
|
|
Lucky1 @ Sun Jan 02, 2011 1:39 pm wrote:
Would this be before the loop? What do you mean replace how to turn on LED? What do the brackets do?
- You can't just throw example code into your own program (at an arbitrary chosen location!) and expect it to do anything useful. Try to understand what the example is trying to demonstrate, then implement the idea on your own.
- The comments are placeholders for your parallelput and timing statements
- Brackets group expressions together to either set a particular priority or for style |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
|
|