Writing a null int to variable when reading file
Author |
Message |
cam1878
|
Posted: Thu Jun 10, 2010 9:55 am Post subject: Writing a null int to variable when reading file |
|
|
What is it you are trying to achieve?
Copy a file to another computer over the network
What is the problem you are having?
When using the put command, it attempts to put a null int into the netStream.
Describe what you have tried to solve this problem
I have tried initializing all the integers in the array to zero, but they are overwritten with null when the program reads a null character from the file.
Turing: |
var file, net : int
var data : flexible array 1 .. 0 of int
var count : int := 0
open : file, "file.exe", read, seek, mod
loop
exit when eof (file )
count + = 1
new data, count %Initializes each new array element to zero
read : file, data (count ) %Overwrites the initialization
end loop
close : file
for i : 1 .. count
put net, data (i ) %Attemps to put a null int, resulting in an error
end for
put "DONE"
|
Please specify what version of Turing you are using
4.0.5
I would like to point out that the following code successfully creates a new file which is identical to the first, by reading the data the same way, but it writes it rather than putting it to the netStream.
Turing: |
var file : int
var data : flexible array 1 .. 0 of int
var count : int := 0
open : file, "file.exe", read, seek, mod
loop
exit when eof (file )
count + = 1
new data, count
data (count ) := 0 %Initializes every new element in the array to zero
read : file, data (count ) %Reads a null character causing an error
end loop
close : file
open : file, "file2.exe", write
for i : 1 .. count
write : file, data (i ) %Error appears at some point while sending the file
end for
put "DONE"
close : file
|
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
DemonWasp
|
Posted: Thu Jun 10, 2010 10:12 am Post subject: RE:Writing a null int to variable when reading file |
|
|
Not sure whether this is your problem, but your put line doesn't have a colon ( : ) indicating that it's supposed to output to the net stream. Instead of saying "put data(i) to net" it's saying "put net, then put data(i)". |
|
|
|
|
 |
cam1878
|
Posted: Thu Jun 10, 2010 10:16 am Post subject: Re: Writing a null int to variable when reading file |
|
|
Sorry that was just a typo, I must have hit backspace it by mistake. |
|
|
|
|
 |
chrisbrown

|
Posted: Thu Jun 10, 2010 10:51 am Post subject: Re: Writing a null int to variable when reading file |
|
|
I tried doing the same thing a few years ago and ran into the exact same problem. I don't know if it's possible given Turing's limited capabilities, but a workaround would be to test for a null int, and send an alternate, non-null int instead. The problem is, you have to make sure that alternate is not something that might be sent intentionally as actual data.
I vaguely recall hearing rumours of another fix that involved modifying the libraries, but I never saw it in action.
On another note, resizing large arrays is costly and should be minimized. You will get better performance if you replace Turing: | var data : flexible array 1 .. 0 of int
% AND
new data, count | with Turing: | var data : flexible array 1 .. 1 of int
% AND
if count > upper(data ) then
new data, upper(data ) * 2
end if |
I noticed a 20% speedup for a 3 MB file. |
|
|
|
|
 |
Tony
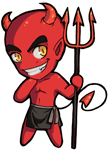
|
Posted: Thu Jun 10, 2010 12:58 pm Post subject: RE:Writing a null int to variable when reading file |
|
|
There is indeed a problem with sending NULLs. If both the client and the server code is your own, then you can send encoded data to avoid NULLs and decode it on the receiving end. Keep in mind the overhead of size and complexity in transmission when designing an appropriate scheme. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
cam1878
|
Posted: Fri Jun 11, 2010 5:14 am Post subject: Re: Writing a null int to variable when reading file |
|
|
That's what I was planning on doing, but I don't know how to check if an integer is indeed null or not without getting an error in the process of checking. |
|
|
|
|
 |
|
|