Creating New Instances of a Procedure
Author |
Message |
Searing
|
Posted: Tue Jun 08, 2010 7:40 pm Post subject: Creating New Instances of a Procedure |
|
|
What is it you are trying to achieve?
I am trying to create a window with a top-bar menu which leads to an input screen, which gets the input and goes back to the main screen
What is the problem you are having?
To call the procedure that creates the pop-up window, that procedure must be above the procedure that creates the menu, but for that pop-up window to go back to the main screen, it has to have THAT procedure above it, which creates the paradox in which both procedures need to be above one another.
Describe what you have tried to solve this problem
I tried to think if I could use a loop, but because I am using buttons, I already have a GUI.ProcessEvent loop, so I have thought about creating another instance of a procedure, which I don't really know how to do
Post any relevant code (You may choose to attach the file instead of posting the code if it is too long)
Here is all my code:
Turing: |
%***** *****
%Create-A-Car!
%30/05/10
%Ms.****
%This program allows the user to create a car using components of their choice and view the car they made
%Imports all the needed GUI
import GUI
%Decleration Section
var buildWin : int := 0
var mainWin := Window.Open ("position:320;300, graphics:640;400")
var mainMenuButton, exitButton, buildButton : int := 0
var titlePictureID : int := Pic.FileNew ("title.jpg")
var licensePlateCode : string
%Set up screen
setscreen ("noecho")
%Program Title
procedure title
cls
Pic.Draw (titlePictureID, 183, 338, picMerge)
end title
%Program Intro Screen
procedure introduction
Window.Hide (buildWin )
var introPictureID : int := Pic.FileNew ("IntroPic.bmp")
title
Pic.Draw (introPictureID, 100, 73, picMerge)
GUI.Show (mainMenuButton )
Pic.Free (introPictureID )
end introduction
%Program Main Menu
procedure mainMenu
var whiteCarID : int := Pic.FileNew ("whiteCar.jpg")
Window.Hide (buildWin )
Window.Show (mainWin )
GUI.Hide (mainMenuButton )
title
Pic.Draw (whiteCarID, 100, 100, picMerge)
GUI.Show (exitButton )
GUI.Show (buildButton )
end mainMenu
procedure licensePlateInput
var inputWindow : int := Window.Open ("position:800;800, graphics:100;100")
var key : string (1)
title
locate (6, 1)
put "Enter a license plate code you want 6 characters in length: " ..
get licensePlateCode
if length (licensePlateCode ) not= 6 then
put "Error! License plate must be 6 characers in length!"
put "Press any key to continue: " ..
getch (key )
licensePlateInput
else
buildCar
end if
end licensePlateInput
%Program build screen
procedure buildCar
%Declares variables
buildWin := Window.Open ("position:100;100, graphics:800;600")
var carMake, carColour, carModel, carDoors, carBumper, licensePlate, hubCap, start : int
var item : array 1 .. 20 of int
%Hides GUI and other windows
Window.Hide (mainWin )
Window.Show (buildWin )
GUI.Hide (buildButton )
GUI.Hide (exitButton )
%Menu Creation
carMake := GUI.CreateMenu ("Make")
item (1) := GUI.CreateMenuItem ("Cadillac", GUI.Quit)
item (2) := GUI.CreateMenuItem ("Honda", GUI.Quit)
item (3) := GUI.CreateMenuItem ("Toyota", GUI.Quit)
carColour := GUI.CreateMenu ("Colour")
item (4) := GUI.CreateMenuItem ("Black", GUI.Quit)
item (5) := GUI.CreateMenuItem ("Red", GUI.Quit)
item (6) := GUI.CreateMenuItem ("Green", GUI.Quit)
carModel := GUI.CreateMenu ("Model")
item (7) := GUI.CreateMenuItem ("Sedan", GUI.Quit)
item (8) := GUI.CreateMenuItem ("SUV", GUI.Quit)
item (9) := GUI.CreateMenuItem ("Convertible", GUI.Quit)
carDoors := GUI.CreateMenu ("Doors")
item (10) := GUI.CreateMenuItem ("2", GUI.Quit)
item (11) := GUI.CreateMenuItem ("4", GUI.Quit)
carBumper := GUI.CreateMenu ("Bumper")
item (12) := GUI.CreateMenuItem ("Heavy", GUI.Quit)
item (13) := GUI.CreateMenuItem ("Light", GUI.Quit)
item (14) := GUI.CreateMenuItem ("Bling", GUI.Quit)
licensePlate := GUI.CreateMenu ("License Plate")
item (15) := GUI.CreateMenuItem ("Enter a license plate...", licensePlateInput )
hubCap := GUI.CreateMenu ("Hub Cap")
item (16) := GUI.CreateMenuItem ("Plain", GUI.Quit)
item (17) := GUI.CreateMenuItem ("Bling", GUI.Quit)
item (18) := GUI.CreateMenuItem ("Golden", GUI.Quit)
start := GUI.CreateMenu ("Start")
item (19) := GUI.CreateMenuItem ("Main Menu", mainMenu )
item (20) := GUI.CreateMenuItem ("Exit", GUI.Quit)
%Display Box Titles
locate (6, 24)
put "Side View"
locate (6, 68)
put "Front View"
%Display Box Creation Left
drawline (75, 100, 75, 500, 1)
drawline (75, 100, 375, 100, 1)
drawline (375, 100, 375, 500, 1)
drawline (375, 500, 75, 500, 1)
%Display Box Creation Right
drawline (425, 100, 425, 500, 1)
drawline (425, 100, 725, 100, 1)
drawline (725, 100, 725, 500, 1)
drawline (725, 500, 425, 500, 1)
end buildCar
%Goodbye Screen
procedure goodBye
Window.Hide (buildWin )
Window.Show (mainWin )
Window.SetActive (mainWin )
var reply : string (1)
title
locate (6, 1)
put "This program was coded by ***** *****."
put "For more information call ***-***-****."
put ""
put "Press any key to continue..." ..
getch (reply )
Pic.Free (titlePictureID )
Window.Close (mainWin )
end goodBye
%Button Creation
mainMenuButton := GUI.CreateButtonFull (273, 40, 0, "M. Main Menu", mainMenu, 0, "^M", true)
exitButton := GUI.CreateButton (273, 10, 0, "Exit", GUI.Quit)
buildButton := GUI.CreateButton (273, 40, 0, "Build Your Car!", buildCar )
%MainProgram
introduction
loop
exit when GUI.ProcessEvent
end loop
goodBye
|
Please specify what version of Turing you are using
4.1.1 |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
chrisbrown

|
Posted: Tue Jun 08, 2010 7:54 pm Post subject: RE:Creating New Instances of a Procedure |
|
|
Does this thread answer your question? |
|
|
|
|
 |
Searing
|
Posted: Tue Jun 08, 2010 7:59 pm Post subject: RE:Creating New Instances of a Procedure |
|
|
Wow, yes actually, thanks a lot. I didn't really know what to search for, you've saved my ISP XD |
|
|
|
|
 |
chrisbrown

|
Posted: Tue Jun 08, 2010 8:08 pm Post subject: RE:Creating New Instances of a Procedure |
|
|
Glad to help (or guide, at least). It's not an easy thing to find if you don't know what you're looking for. |
|
|
|
|
 |
Searing
|
Posted: Tue Jun 08, 2010 8:19 pm Post subject: RE:Creating New Instances of a Procedure |
|
|
Umm, how do I get this thread deleted? I just noticed I have source code up, which while incomplete, was sort of stupid of me |
|
|
|
|
 |
Monduman11

|
Posted: Tue Jun 08, 2010 8:24 pm Post subject: Re: Creating New Instances of a Procedure |
|
|
umm i dont know i asked tony to delete one of my old ones cause i had a large portion of my game source code and he still hasnt deleted it.... its been almost a month lol |
|
|
|
|
 |
Searing
|
Posted: Tue Jun 08, 2010 8:25 pm Post subject: RE:Creating New Instances of a Procedure |
|
|
Not good, huh, well could I please get a link to the admin group so I could PM a few to delete my thread? |
|
|
|
|
 |
Monduman11

|
Posted: Tue Jun 08, 2010 8:26 pm Post subject: Re: Creating New Instances of a Procedure |
|
|
umm i dont think there is a link but if you go to the turing help or turing tutorial and look for all the the names that are red or blue you can pm them cause their either admin or mod |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Searing
|
Posted: Tue Jun 08, 2010 8:33 pm Post subject: RE:Creating New Instances of a Procedure |
|
|
Strange, most forums have an administrator or moderator group. Thank you for the advice though, I have PMed several moderators and administrators |
|
|
|
|
 |
USEC_OFFICER

|
Posted: Tue Jun 08, 2010 8:41 pm Post subject: RE:Creating New Instances of a Procedure |
|
|
PM Dan or Tony, they are the ones who can delete it. Though I have no idea why you would want to do that. |
|
|
|
|
 |
Tony
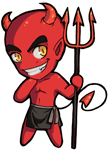
|
Posted: Tue Jun 08, 2010 9:24 pm Post subject: RE:Creating New Instances of a Procedure |
|
|
Dan and myself are administrators. Though we typically don't delete threads. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
copthesaint

|
Posted: Wed Jun 09, 2010 10:35 am Post subject: RE:Creating New Instances of a Procedure |
|
|
You won't get into any trouble posting SC here!
Alot of people with projects for culminating are worried that they will be accused of plagerism by posting SC here, but there is NOTHING to worry about #1 your game will never make it big if it's for a programing language like turing, and even if it's in a language like C+ or java even then your most likly not making a game that will become known at all. its for educational purposes and all for fun. #2 If you are acussed of steal anothers code, you can show the teacher that it is your account which you posted the source code, and there is the date which it is posted as well, incase someone has taken your code and used it else where. #3 Programing teachers don't look to see if you plagiarized IF YOU COMMENT AND UNDERSTAND WHAT YOUR DOING!
but anyways... lol to make a meaningful 400th post :p |
|
|
|
|
 |
|
|