Lowest amount of bills/coins
Author |
Message |
Slnj
|
Posted: Sat Mar 27, 2010 9:31 pm Post subject: Lowest amount of bills/coins |
|
|
What is the problem you are having?
Im stuck
Questions : Write a program that inputs a dollar amount and outputs how the amount can be represented using the fewest number of bills and coins. Hint: Use the mod and div commands.
Post any relevant code (You may choose to attach the file instead of posting the code if it is too long)
I have tried doing it on my own but got stuck beacuse say if i put $150 as the amount of money it will only says 1 hundred dollar bill and it won't say 1 fifty dollar bill.
Turing: |
var money, a , b, c, d: real
put "What is the amount of money? "
get money
if money >=100 then
a := money div 100 put money, " hundred dollar bill(s)"
elsif money <100 and money >=50 then
b := money div 50 put b," fifty dollar bill(s)"
elsif money <=50 then
c := money div 20 put c, " twenty dollar bill(s)"
elsif money <20 and money >=10 then
d := money div 10 put d, " ten dollar bill(s) "
end if
|
Please specify what version of Turing you are using
latest version 4.11 i think |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
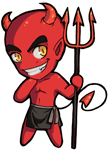
|
Posted: Sat Mar 27, 2010 9:38 pm Post subject: RE:Lowest amount of bills/coins |
|
|
One you take out that one $100 bill, how do you know that you have $50 more left? (That answer is not described in your code) |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Shah-Cuber

|
Posted: Sat Mar 27, 2010 9:48 pm Post subject: Re: Lowest amount of bills/coins |
|
|
A good way to learn Dynamic Programming (well-known problem).
I'll let you figure it out ... |
|
|
|
|
 |
Slnj
|
Posted: Sun Mar 28, 2010 7:28 am Post subject: RE:Lowest amount of bills/coins |
|
|
ok so tried again i thought i got it but then i almost got it =/
here's what my code looks like can guy's see what i did wrong or what should add in there?
Turing: |
var money, a , b, c, d, e, f, g, h, i, j , k: real := 0
put "What is the amount of money? "
get money
put " "
put "Change For : " : 10, money : 12
put " "
if money >=100.00 then
a := money div 100.00
money := money mod 100
end if
if money <100.00 and money >=50.00 then
b := money div 50.00
money := money mod 50.00
end if
if money <=50.00 then
c := money div 20.00
money := money mod 20.00
end if
if money <20.00 and money >=10.00 then
d := money div 10.00
money := money mod 10.00
end if
if money <10.00 and money >=5.00 then
e := money div 5.00
money := money mod 5.00
end if
if money <5.00 and money >=2.00 then
f := money div 2.00
money := money mod 2.00
end if
if money <=2.00 and money >=1.00 then
g := money div 1.00
money := money mod 1.00
end if
if money <1.00 and money >=0.25 then
h := money div 0.25
money := money mod 0.25
end if
if money <0.25 and money >=0.10 then
i := money div 0.10
money := money mod 0.10
end if
if money <0.10 and money >=0.05 then
j := money div 0.05
money := money mod 0.05
end if
if money <0.05 and money >=0.01 then
k := money div 0.01
end if
|
look at the image i put 0.15 but it give me 4 pennies and 1 dime? what did i do wrong
 |
|
|
|
|
 |
chrisbrown

|
Posted: Sun Mar 28, 2010 11:02 am Post subject: RE:Lowest amount of bills/coins |
|
|
Interesting, its caused by floating-point error. I haven't seen such a simple example of it.
Anyway, you didn't do anything wrong. Since decimals aren't exact, when you expect money to have the value 0.05, it might actually be more like 0.0499999, so the if condition is false.
After you get money, multiply it by 100, and change your values to compare based on cents, not dollars. |
|
|
|
|
 |
Slnj
|
Posted: Sun Mar 28, 2010 11:20 am Post subject: Re: RE:Lowest amount of bills/coins |
|
|
methodoxx @ Sun Mar 28, 2010 11:02 am wrote: Interesting, its caused by floating-point error. I haven't seen such a simple example of it.
Anyway, you didn't do anything wrong. Since decimals aren't exact, when you expect money to have the value 0.05, it might actually be more like 0.0499999, so the if condition is false.
After you get money, multiply it by 100, and change your values to compare based on cents, not dollars.
can't do that because i need to use dollars. so i guess i did it everything right? |
|
|
|
|
 |
chrisbrown

|
Posted: Sun Mar 28, 2010 11:34 am Post subject: Re: RE:Lowest amount of bills/coins |
|
|
Slnj @ Sun Mar 28, 2010 11:20 am wrote: can't do that because i need to use dollars. so i guess i did it everything right?
Well, it's right in the sense that it would work in an ideal world, but since it doesn't produce correct answers, it's not really right.
There's nothing stopping you from dividing by 100 afterwards to convert back into dollars. |
|
|
|
|
 |
Euphoracle

|
Posted: Sun Mar 28, 2010 1:57 pm Post subject: RE:Lowest amount of bills/coins |
|
|
Multiply all your values by 100, including your input value. This should eliminate the floating point errors.
eg. Checking for $100 is now checking for '10000'; checking for pennies, or $0.01, is now checking for '1'. Your input, if it was $15.99, would now be '1599' |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Slnj
|
Posted: Mon Mar 29, 2010 2:23 pm Post subject: RE:Lowest amount of bills/coins |
|
|
Thanks guy's i managed to do what you guy's said with some help from some people in the chat room.  |
|
|
|
|
 |
|
|