Moving a sprite image diagonally?
Author |
Message |
threatbinder
|
Posted: Wed Dec 16, 2009 6:39 pm Post subject: Moving a sprite image diagonally? |
|
|
So to move a sprite image vertically or horizontally you just use one for loop
e.g., :
var pic1 := Pic.FileNew ("ball.bmp")
var sprite1 := Pic.FileNew (pic1)
for i: 100 .. 500
Sprite.SetPosition (sprite1, i, 300, true)
delay (10)
end for
this example shows the ball moving horizontally from 100 to 500, but I don't get how to do this for moving it diagonally.. because using two for loops don't work.
Here's what I mean (an example that proves my point of two for loops that won't work for moving a sprite diagonally)
for x: 100 .. 500
for y: 50 .. 100
Sprite.SetPosition (sprite1, x, y, true)
delay (10)
end for
end for
So this will repeat the second for loop 400 times, which is not good because then instead of moving diagonally, it will show the sprite moving vertically, shifting 1 pixel, moving vertically all the way again, shifting 1 pixel, and so on..
So please, can anyone tell me how I would shift a sprite diagonally? ANY help is HIGHLY appreciated, I already thought this over for at least 2 hours.. can't figure it out. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
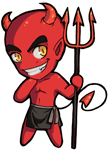
|
Posted: Wed Dec 16, 2009 6:42 pm Post subject: RE:Moving a sprite image diagonally? |
|
|
Here's what I want you to do. Start writing out the locations at which you draw your sprite. In the first example, it would be
100,300
101,300
102,300
...
499,300
500,300
How would the numbers look for diagonal movement? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
threatbinder
|
Posted: Wed Dec 16, 2009 6:57 pm Post subject: Re: RE:Moving a sprite image diagonally? |
|
|
Tony @ Wed Dec 16, 2009 6:42 pm wrote: Here's what I want you to do. Start writing out the locations at which you draw your sprite. In the first example, it would be
100,300
101,300
102,300
...
499,300
500,300
How would the numbers look for diagonal movement?
100, 50
101, 51
102, 52
103, 53
104, 54
...
499, 299
500, 300
well.. that means the x and y increases by 1 each time, but the problem is... the range for the first loop has to be the same as the x start, x end coordinates and the amount of times you want to loop..
thanks for the quick reply |
|
|
|
|
 |
Tony
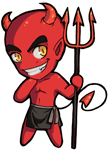
|
Posted: Wed Dec 16, 2009 7:10 pm Post subject: RE:Moving a sprite image diagonally? |
|
|
Well not quite. Because if both x and y are incremented by the same amount, same number of times, then the difference between x_final y_final should be the same as x_start y_start
That is: 500, 450
Are you talking about diagonals that are not at 45 degree angle? (That is, x/y increments are not 1/1)? You could keep x and y as "real" variables, and increment by fractions, but you'd need to remember to use round() before drawing the points. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
threatbinder
|
Posted: Wed Dec 16, 2009 7:23 pm Post subject: Re: RE:Moving a sprite image diagonally? |
|
|
Tony @ Wed Dec 16, 2009 7:10 pm wrote: Well not quite. Because if both x and y are incremented by the same amount, same number of times, then the difference between x_final y_final should be the same as x_start y_start
That is: 500, 450
Are you talking about diagonals that are not at 45 degree angle? (That is, x/y increments are not 1/1)? You could keep x and y as "real" variables, and increment by fractions, but you'd need to remember to use round() before drawing the points.
Kind of.
I'm talking about integer diagonals, and how does the angle affect the for loop?
hmm.. so this is what my program needs-
a sprite that moves diagonally - it will go a horizontal direction by the same amount as the vertical direction, however, the sprite's x start is not the same as the x increment. same with the y. did that make sense? it's kind of hard to explain.. think about it as a ball that can only be kicked diagonally..
or even better: think of it as chess.. you know how queens, bishops, (sometimes) pawns have to move diagonally? if the sprite of a bishop is located at 60x60, and i have to move it to the right 50 times and up 50 times, my start location is not the same as the increment. |
|
|
|
|
 |
Tony
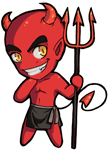
|
Posted: Wed Dec 16, 2009 7:39 pm Post subject: RE:Moving a sprite image diagonally? |
|
|
Ok, I see what you are saying. Would it help to know that you can use expressions in place of variables? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
threatbinder
|
Posted: Wed Dec 16, 2009 7:47 pm Post subject: Re: RE:Moving a sprite image diagonally? |
|
|
Tony @ Wed Dec 16, 2009 7:39 pm wrote: Ok, I see what you are saying. Would it help to know that you can use expressions in place of variables?
I kind of tried using expressions like variable - 1 inside the for loop already and it doesn't work. Could you further explain? Your advice is kind of too vague and I'm too dumb to figure that out.
Ok, here's a specific example:
I want to move the ball sprite from 0 to 100 horizontally, while doing so, I want to move the ball sprite from 50 to 100 vertically as well. So how would an expression work in this case? |
|
|
|
|
 |
Tony
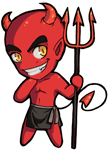
|
Posted: Wed Dec 16, 2009 7:51 pm Post subject: RE:Moving a sprite image diagonally? |
|
|
code: |
for i:1..10
put 5 + i
end for
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
threatbinder
|
Posted: Wed Dec 16, 2009 8:19 pm Post subject: Re: RE:Moving a sprite image diagonally? |
|
|
Tony @ Wed Dec 16, 2009 7:51 pm wrote: code: |
for i:1..10
put 5 + i
end for
|
Okay.. if I take this scenario for example, "Move a ball from 0 to 100 horizontally, whilst moving the ball from 50 to 100 vertically.", the x will start at 0 and end at 100, while the y has to move from 50 to 100.
code: |
for x:0..100
for i:0..50
Sprite.SetPosition (ball, x, i+50, false)
delay (10)
end for
end for
|
hmm.. I guess that will work but that kind of limits things.. I'll try that with my current program and see if it works.
thanks for the help btw |
|
|
|
|
 |
|
|