Author |
Message |
TomCool
|
Posted: Sun Dec 13, 2009 3:42 pm Post subject: Flexible Arrays in a Type Record... |
|
|
Doesn't work when I do this...
Turing: |
type SHIP :
record
x_pos : int
y_pos : int
size : int
direction : boolean
piece : flexible array 1 .. 0 of int
end record
|
... Just highlights 'flexible' and tells me its a syntax error.
Works when I just do...
Turing: |
var piece : flexible array 1 .. 0 of int
|
So can flexible arrays just not be put into the type record thing, or what..?
Thanks~ |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
TheGuardian001
|
Posted: Sun Dec 13, 2009 3:48 pm Post subject: Re: Flexible Arrays in a Type Record... |
|
|
as far as I know, Turing simply doesn't allow for it. You either have to use fixed dimensions on your array, or not use types. There may be some way to work around this, but if there is I don't know it. |
|
|
|
|
 |
Tony
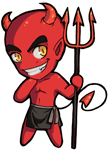
|
Posted: Sun Dec 13, 2009 4:02 pm Post subject: RE:Flexible Arrays in a Type Record... |
|
|
A type needs to know its size, so that a proper amount of space could be allocated for it. If you are changing the size from within some types, then you can't have an array of them, so a compiler doesn't let you do it.
You could though have a pointer to a flexible array. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Insectoid

|
Posted: Sun Dec 13, 2009 4:54 pm Post subject: RE:Flexible Arrays in a Type Record... |
|
|
Turing sucks; it's too limited. Records are just a panzy imitation of a data object with half the functionality. |
|
|
|
|
 |
Tony
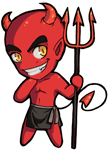
|
Posted: Sun Dec 13, 2009 4:56 pm Post subject: RE:Flexible Arrays in a Type Record... |
|
|
It's not just Turing. You can't have variable length structs in C++ either. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Insectoid

|
Posted: Sun Dec 13, 2009 5:22 pm Post subject: RE:Flexible Arrays in a Type Record... |
|
|
Well, we all know how backward C++ is. How I love languages where each element of an array is individually typed and they are dynamic by default, and can change the type at a keystroke. |
|
|
|
|
 |
Tony
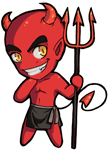
|
Posted: Sun Dec 13, 2009 5:36 pm Post subject: RE:Flexible Arrays in a Type Record... |
|
|
All I'm saying is that there is a valid design reasons for now allowing variable size within types. I know that everybody likes to dislike Turing, but this is not a valid argument against it. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
DtY

|
Posted: Sun Dec 13, 2009 7:00 pm Post subject: RE:Flexible Arrays in a Type Record... |
|
|
So do flexible arrays actually reallocate memory when add elements to them?
I thought they were linked lists |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
mirhagk
|
Posted: Sun Dec 13, 2009 11:35 pm Post subject: RE:Flexible Arrays in a Type Record... |
|
|
wow tony i never even thought of using a pointer to a flexible array in a record, does it all work out? |
|
|
|
|
 |
DemonWasp
|
Posted: Sun Dec 13, 2009 11:48 pm Post subject: RE:Flexible Arrays in a Type Record... |
|
|
@Tony: You can have variable-length structs in C/C++, you just have to cheat (forgive my syntax fail):
code: |
struct {
int length;
char[1] data;
} vls ; // vls = "variable-length struct"
vls s = (vls) malloc ( 4+20 );
s->data[10] = 'm';
|
It's not pretty (and generally not a good idea) but it is possible.
@insectoid: Turing's record is pretty equivalent to C's struct, much as Turing's union is pretty similar to C's union. This is not a failing of the language, it's by design (and a relatively good design). Generally, data objects are fixed-length at creation time; the only reason that classes let you have variable-length elements in them is that these are implemented as pointers to variable-length elements, and the pointer itself is of fixed length. The reason is this problem: how does one allocate memory for objects which may have variable length without fragmenting objects?
There are also some pretty serious downsides to having array elements with varying types (though they are occasionally useful). There's very little to say that you can't have " each element of an array is individually typed and they are dynamic by default, and can change the type at a keystroke" in languages like Java or C++, you just have to have declare the array as an array of "SuperClass" and then any entries can actually be objects of "XSubClass" or "YSubClass", whatever ends up getting put into the array (or list...). Unless I'm missing something, that's exactly what you're talking about.
@DtY: Although flexible arrays could be implemented as linked lists (or array lists, or any other list structure), they are almost certainly implemented as arrays in the Holtsoft Turing implementation. Such an implementation has a fixed-length array and an upper and lower bound.
This implies that if you new them to a smaller size, their bounds change but nothing else happens. If you new to a bigger size, then the following happens:
1. A new array of size (new_size) is allocated.
2. Elements in the old array are copied to the new array.
3. The old array is deallocated and the new array is used in its place.
4. Bounds are updated.
You can imagine that step 2 might be more than a little bit slow. This is why there are heuristics to improve the performance of such an implementation. A simple one is to avoid increasing size by 1 each time, instead doubling the size each time - this vastly decreases the number of reallocations, making those operations increasingly infrequent as the size of the list increases. See also my implementation of the ArrayList collection type. |
|
|
|
|
 |
Tony
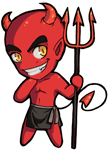
|
Posted: Sun Dec 13, 2009 11:52 pm Post subject: RE:Flexible Arrays in a Type Record... |
|
|
If you know how to use pointers, yes. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
|