Author |
Message |
ella

|
Posted: Wed Dec 02, 2009 6:04 pm Post subject: How to swap letters? |
|
|
Basically, I have to open a file (words.txt), which contains all my data (string). So, after I finished opening the file, its contents (let's say, four words), I must swap the letters that composed of that word (e.g. TURING when swap: UTGNIR). This program should be "procedure" (swapping of letters). Can someone help me to start since I only have a few basic knowledge about Turing. I really need help. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
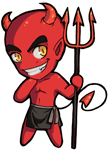
|
Posted: Wed Dec 02, 2009 6:16 pm Post subject: RE:How to swap letters? |
|
|
You can build this in parts, and then connect them.
Start with reading from a file, and then just printing the words to screen.
Then create a procedure that takes a string as an argument.
Then have the procedure swap the letters in the string.
Finally go back to the part where you were reading words from a file, and instead of printing to screen, direct those words into the procedure instead. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
ella

|
Posted: Wed Dec 02, 2009 6:27 pm Post subject: RE:How to swap letters? |
|
|
How do I call the words in a file individually? |
|
|
|
|
 |
Tony
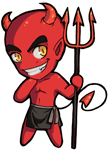
|
|
|
|
 |
ella

|
Posted: Thu Dec 03, 2009 12:34 pm Post subject: RE:How to swap letters? |
|
|
Thanks. But, can you please show some sample programming structure on how to swap the letters using 'for' loops/loops and/or 'if' structures? |
|
|
|
|
 |
Tony
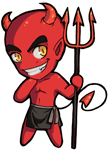
|
Posted: Thu Dec 03, 2009 3:14 pm Post subject: RE:How to swap letters? |
|
|
Here's an example of working with letters within a string. This should give you enough tools to figure out swapping.
code: |
var word : string := "tony"
put word(2)
word := 'T' + word(2..*)
put word
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
ella

|
Posted: Thu Dec 03, 2009 8:04 pm Post subject: RE:How to swap letters? |
|
|
Can I have another question? It's not really related to swapping letters.
I have these codes which convert the user's inputted guess to uppercase.
Turing: |
var guess : string
function uppercase : string
var letter : string
var asciinum : int
var letters : array 1 .. 26 of string
for b : 1 .. length (guess )
letter := guess (b )
% use chr and ord to convert to uppercase
asciinum := ord (letter )
% check if its already uppercase
if asciinum <= 90 and asciinum >= 65 then
letters (b ) := letter
else
asciinum := asciinum - 32
letters (b ) := chr (asciinum )
end if
end for
result letters
end uppercase
|
And I also have these codes in which the user will input his guess.
Turing: |
procedure guessing
put "Enter your guess"
get guess
if guess = words then
put "You're right!"
else
put "Wrong word."
put "The correct word is: ", words
end if
end guessing
|
My problem is, how would I call the uppercase function in that guessing procedure? If the user will enter his guess, his guess will change into uppercase. Help. |
|
|
|
|
 |
Tony
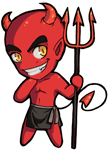
|
Posted: Thu Dec 03, 2009 9:29 pm Post subject: RE:How to swap letters? |
|
|
Because the scope of the variable guess is outside of the function, then any changes to it will also persist outside of the function (thus you see it as the user's actual guess being modified). You want to use an argument instead
code: |
function uppercase (guess : string) : string
...
|
Then
code: |
get user_guess
if uppercase(user_guess) = words then
...
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
|