Making my character land on an object (making an object solid)
Author |
Message |
drummaboy
|
Posted: Fri Nov 27, 2009 10:25 pm Post subject: Making my character land on an object (making an object solid) |
|
|
I'm trying to make this game called Super Ball and I got the ball to move and suttf
but I want to know how to make an object solid, so that the ball cant go through it.
As you can see in my game , there's a black platforma thingy (drawfillbox) I want to make it so that the ball can't go through it
and if the ball lands on it, it stays on it and it the ball hits it from underneath , it doesn't go through the platform.
Thanks.
Here's the code.
% Super Box Game
%Made by Peter Henry
%First procedure for the background
procedure background
drawfillbox (0, 0, maxx, maxy, brightblue)
drawfillbox (0, 0, maxx, 10, green)
var fnt : int
fnt := Font.New ("Bookman Old Style:25")
Draw.Text ("Super Ball", 1, 350, fnt, brightred)
const bar_height := 30
const bar_length := 50
const bar_height_2 := 50
const bar_length_2 := 90
drawfillbox (bar_length, bar_height, bar_length_2, bar_height_2, black)
end background
%characters movement
procedure maincharacter
%different constants
const GROUND_HEIGHT := 20
const RUN_SPEED := 2
const JUMP_SPEED := 15
const GRAVITY := 2
%position of character and velocity
var posx, posy : int
var velx, vely : real
var chars : array char of boolean
posx := 5
posy := 21
velx := 0
vely := 0
loop
Input.KeyDown (chars) % character moving left
if chars (KEY_LEFT_ARROW) then
velx := -RUN_SPEED
elsif chars (KEY_RIGHT_ARROW) then %character moving right
velx := RUN_SPEED
else
velx := 0
end if
%if you press z , you jump
if chars ('z') and posy = GROUND_HEIGHT then
vely := JUMP_SPEED
end if
vely -= GRAVITY %subtracting the gravity from the velocity going upwards
posx += round (velx)
posy += round (vely)
if posy < GROUND_HEIGHT then
posy := GROUND_HEIGHT
vely := 0
end if
drawoval (posx, posy, 4, 4, brightred)
delay (50)
drawoval (posx, posy, 4, 4, brightblue)
const bar_height_2 := 50
if posx = bar_height_2 then
posx := bar_height_2
end if
end loop
end maincharacter
background
maincharacter
Can you please change the code but still explain what you did because i want to learn. Thanks
Oh and I'm using the latest Turing 4.1.1 (which i think is just 4.1) |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
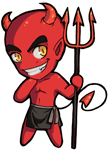
|
Posted: Sat Nov 28, 2009 2:25 pm Post subject: Re: Making my character land on an object (making an object solid) |
|
|
drummaboy @ Fri Nov 27, 2009 10:25 pm wrote: Can you please change the code...
No, it doesn't work like that.
But this should be easy. You've already implemented code to not fall through the ground. You can use similar ideas to not fall through the platform as well (think of "ground" as a really large platform"). |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Kharybdis

|
Posted: Sun Nov 29, 2009 12:05 am Post subject: RE:Making my character land on an object (making an object solid) |
|
|
Well, let's say you have circle A trying to horizontally move across a line where Circle B is on that line.
You have to find out when Circle A is touching Circle B so it doesn't go through it.
So, if circle a starts at x = 0 and y = 0 (where y never changes) and circle b is at x = 50 and y =0, then if Circle A is at x=50, then it means it's touching Circle B, and thus Circle A has to be immediately assigned to x = 49 because it can't go through Circle B. You should change the parameter of movement that Circle A has to go through because if you continue to increase the value of x, you're going to have Circle A jumping to x=50 and x=49 constantly.
It's the same principle as if you had to change the y vector (position) of Circle A. |
|
|
|
|
 |
drummaboy
|
Posted: Sun Nov 29, 2009 12:44 pm Post subject: Re: Making my character land on an object (making an object solid) |
|
|
Thanks Tony... You actually made me think of it in a different way. I finally got my character to jump on the platform.
But I don't know how to make him fall when he gets off the platform.
Could you please help me with that?
code: |
% Super Box Game
%Made by Peter Henry
%First procedure for the background
procedure background
drawfillbox (0, 0, maxx, maxy, brightblue)
drawfillbox (0, 0, maxx, 10, green)
var fnt : int
fnt := Font.New ("Bookman Old Style:25")
Draw.Text ("Super Ball", 1, 350, fnt, brightred)
const bar_height := 30
const bar_length := 50
const bar_height_2 := 50
const bar_length_2 := 90
drawfillbox (bar_length, bar_height, bar_length_2, bar_height_2, black)
end background
%characters movement
procedure maincharacter
%different constants
const GROUND_HEIGHT := 20
const GROUND_HEIGHT2 := 55
const GROUND_LENGTH := 50
const GROUND_LENGTH2 := 90
const RUN_SPEED := 2
const JUMP_SPEED := 15
const GRAVITY := 2
%constants from background procedure
const bar_height := 30
const bar_length := 50
const bar_height_2 := 50
const bar_length_2 := 90
%position of character and velocity
var posx, posy : int
var velx, vely : real
var chars : array char of boolean
posx := 5
posy := 21
velx := 0
vely := 0
loop
Input.KeyDown (chars) % character moving left
if chars (KEY_LEFT_ARROW) then
velx := -RUN_SPEED
elsif chars (KEY_RIGHT_ARROW) then %character moving right
velx := RUN_SPEED
else
velx := 0
end if
%if you press z , you jump
if chars ('z') and posy = GROUND_HEIGHT or posy = GROUND_HEIGHT2 then
vely := JUMP_SPEED
end if
vely -= GRAVITY %subtracting the gravity from the velocity going upwards
posx += round (velx)
posy += round (vely)
if posy < GROUND_HEIGHT then
posy := GROUND_HEIGHT
vely := 0
end if
if posy > GROUND_HEIGHT2 and posx > GROUND_LENGTH then
posy := GROUND_HEIGHT2
vely :=0
elsif posy >= GROUND_HEIGHT2 and posx >= GROUND_LENGTH2 then
posy := GROUND_HEIGHT
vely -= GRAVITY
end if
drawoval (posx, posy, 4, 4, brightred)
delay (50)
drawoval (posx, posy, 4, 4, brightblue)
end loop
end maincharacter
background
maincharacter |
Mod Edit: added [ code ] tags. |
|
|
|
|
 |
Tony
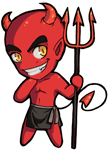
|
Posted: Sun Nov 29, 2009 3:07 pm Post subject: RE:Making my character land on an object (making an object solid) |
|
|
You're getting closer. A couple of points to help you figure things out:
code: |
if chars ('z') and posy = GROUND_HEIGHT or posy = GROUND_HEIGHT2 then
|
and takes precedence over or. The code above is the same as
code: |
if (chars ('z') and posy = GROUND_HEIGHT) or posy = GROUND_HEIGHT2 then
|
And I'm certain that is not what you've meant to do.
Constant names such as "GROUND_HEIGHT2" and "GROUND_LENGTH2" make it more difficult to follow what is going on. I suspect that it's not so much about all of the ground anymore (as oppose to GROUND_HEIGHT), but about some regions that behave in a special way.
For the platform, you should be checking against "bar_height", etc. -- same constants you've used to draw the platform.
Another note regarding bar_length/bar_length_2 -- you are using them as start_x_position and end_x_position, for the same platform. Your current naming convention alludes that there are 2 platforms of a particular length, which is not the case. You might have been told that the compiler doesn't care what names you pick, but if you expect anyone else to ever read your code (myself, others on the forums, your teacher), good variable (and procedure) naming is important. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
drummaboy
|
Posted: Wed Dec 02, 2009 12:48 pm Post subject: Re: Making my character land on an object (making an object solid) |
|
|
Thanks. But I have a question. I heard that whatdotcolor could make it easier for me. I read about it in Turing help and I sort of understand it. But how could I make it so that when my ball detects the platform it stays on it? |
|
|
|
|
 |
drummaboy
|
Posted: Wed Dec 02, 2009 12:49 pm Post subject: Re: Making my character land on an object (making an object solid) |
|
|
also when you go under the platform and jump, it goes through the platform, how do I make it so that it doesnt't happen with whatdotcolor |
|
|
|
|
 |
Tony
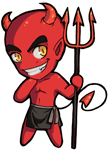
|
Posted: Wed Dec 02, 2009 2:58 pm Post subject: RE:Making my character land on an object (making an object solid) |
|
|
The problem with whatdotcolour is that it's using the same memory space for drawing images and figuring out the locations. That forces you to use only simple distinct colours; and whenever you draw anything, that also change the shape of the level.
An example of such problem would be not properly checking some corner, allowing a character to partially overlap a wall (maybe just by a pixel). The character would then be able to erase the wall's image and walk through that.
Though if you insist, then you'd have to check the colours all around your ball, and use that colour information to guess as to where on the level the ball is, and how it should react. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
|
|