Irregular shape.
Author |
Message |
MossyMossy
|
Posted: Mon Nov 09, 2009 1:58 pm Post subject: Irregular shape. |
|
|
I need to write a turing program that takes 4 random points to form an irregular shape. It will then fill in the shape wiht asterisks so it becomes solid.
A loop is required.
example:
please enter the row cordinates for pt 1 (upper left):
please enter the col cordinates for pt 1 (upper left):
please enter the row cordinates for pt 2 (upper right):
please enter the col cordinates for pt 2 (upper right):
please enter the row cordinates for pt 1 (lower right):
please enter the col cordinates for pt 1 (lower right):
please enter the row cordinates for pt 1 (lower left):
please enter the col cordinates for pt 1 (lower left):
assume that the 4 points entered will form a proper irregular shape.
like this:
This is a valid shape and must be filled with asterisks.
I will be working on this after school is finished, but I need help with this,
its too confusing atm, and i will be looking threw tutorials when i get home.
thanks. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
apomb

|
Posted: Mon Nov 09, 2009 2:02 pm Post subject: RE:Irregular shape. |
|
|
since you're dealing with coordinates, Text.Locate() will be useful.
if you have any code to begin with, that would be beneficial to others who want to help you. |
|
|
|
|
 |
MossyMossy
|
Posted: Tue Nov 10, 2009 3:53 pm Post subject: Re: Irregular shape. |
|
|
MossyMossy @ Mon Nov 09, 2009 1:58 pm wrote: I need to write a turing program that takes 4 random points to form an irregular shape. It will then fill in the shape wiht asterisks so it becomes solid.
A loop is required.
example:
please enter the row cordinates for pt 1 (upper left):
please enter the col cordinates for pt 1 (upper left):
please enter the row cordinates for pt 2 (upper right):
please enter the col cordinates for pt 2 (upper right):
please enter the row cordinates for pt 3 (lower right):
please enter the col cordinates for pt 3 (lower right):
please enter the row cordinates for pt 4 (lower left):
please enter the col cordinates for pt 4 (lower left):
assume that the 4 points entered will form a proper irregular shape.
like this:
This is a valid shape and must be filled with asterisks.
I will be working on this after school is finished, but I need help with this,
its too confusing atm, and i will be looking threw tutorials when i get home.
thanks.
I followed up another code (the draw box one). and played around with it,
Turing: |
var upleftrow, upleftcol, uprightrow, uprightcol : int
put "Enter row of the cordinates for pt1 (upper left): " ..
get upleftrow
put "Enter coloum of the cordinates for pt1(upper left): " ..
get upleftcol
put "Enter row of the cordinates for pt2 (upper right): " ..
get uprightrow
put "Enter coloum of the cordinates for pt1 (upper right): " ..
get uprightcol
setscreen ("graphics")
drawline (uprightrow, uprightcol, uprightcol + uprightcol, uprightcol ) % Base of box
drawline (uprightrow + uprightrow, uprightcol, uprightrow + uprightrow, uprightcol + upleftcol ) % Right side
drawline (uprightrow + upleftrow, upleftrow + upleftcol, uprightrow, uprightcol + upleftcol ) % Top
drawline (uprightrow, upleftcol + upleftcol, upleftcol, uprightcol ) % Left side
|
I still get an error, and its really frustating.
please help....
ontop of that. Its so confusing to fill it with asterisks. (lines) |
|
|
|
|
 |
MossyMossy
|
Posted: Wed Nov 11, 2009 12:03 am Post subject: Re: Irregular shape. |
|
|
still cant get it, please help  |
|
|
|
|
 |
Tony
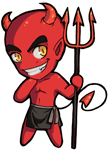
|
Posted: Wed Nov 11, 2009 1:00 am Post subject: RE:Irregular shape. |
|
|
Here's the thing, your assignment is talking about rows and columns, as in
code: |
var row, col:int
row := 2
col := 3
locate(row, col)
put "*"..
|
You are trying to Draw.Line with pixel positions. You are not going to draw any lines, only use locate and put "*"..
(.. prevents the newline character)
You can loop over your entire screen using maxrow and maxcol (see documentation) constants, check if that point is inside the shape or not, and draw the asterisk. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
MossyMossy
|
Posted: Thu Nov 12, 2009 8:59 am Post subject: Re: Irregular shape. |
|
|
Alright, Got it so far,
BUT the draw.line function, I created a new set of variables, just for the draw line, and times it by 10. because of the pixels
Turing: |
locate (21, 1)
put "Number of columns on the screen is ", maxrow
locate (22, 1)
put "Number of rows on the screen is ", maxrow
var numrow, numcol : int
var numrow2, numcol2 : int
var numrow3, numcol3 : int
var numrow4, numcol4 : int
put "please enter your first row"
get numrow
put "please enter your first coloumn"
get numcol
put "please enter your second row"
get numrow2
put "please enter your second coloumn"
get numcol2
put "please enter your third row"
get numrow3
put "please enter your third coloumn"
get numcol3
put "please enter your fourth row"
get numrow4
put "please enter your fourth coloumn"
get numcol4
cls
var row, col: int
row := numrow
col := numcol
locate(row, col )
put "*"..
var row2, col2: int
row2 := numrow2
col2 := numcol2
locate(row2, col2 )
put "*"..
var row3, col3: int
row3 := numrow3
col3 := numcol3
locate(row3, col3 )
put "*"..
var row4, col4: int
row4 := numrow4
col4 := numcol4
locate(row4, col4 )
put "*"..
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
% Draw.line Variables %
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
var drawrow, drawcol: int
drawrow := numrow * 10
drawcol := numcol * 10
var drawrow2, drawcol2: int
drawrow2 := numrow2 * 10
drawcol2 := numcol2 * 10
var drawrow3, drawcol3: int
drawrow3 := numrow3 * 10
drawcol3 := numcol3 * 10
var drawrow4, drawcol4: int
drawrow4 := numrow4 * 10
drawcol4 := numcol4 * 10
Draw.Line(drawcol,drawrow,drawcol2,drawrow2, 5)
|
|
|
|
|
|
 |
|
|