Dice Game
Author |
Message |
GamingFusion
|
Posted: Thu Oct 29, 2009 11:15 am Post subject: Dice Game |
|
|
I am trying to make a Turing Dice Game.
i want it so when the user presses the ENTER KEY, it will quit my loop for roll the dice images and displays the random dice images. I dont have the random cide number yet but thats easy enough.
I want it to check until the ENTER KEY is pressed
I have tried putting in a double loop and a single loop checking for a key press after every image but both didnt work
Turing: |
% The "Pic.FileNew" program.
var dice : array 1 .. 6 of int
var x, y : int
const delayy := 100
var chars : array char of boolean
dice (1) := Pic.FileNew ("H:/Turing/Dice/Images/dice1.jpg")
dice (2) := Pic.FileNew ("H:/Turing/Dice/Images/dice2.jpg")
dice (3) := Pic.FileNew ("H:/Turing/Dice/Images/dice3.jpg")
dice (4) := Pic.FileNew ("H:/Turing/Dice/Images/dice4.jpg")
dice (5) := Pic.FileNew ("H:/Turing/Dice/Images/dice5.jpg")
dice (6) := Pic.FileNew ("H:/Turing/Dice/Images/dice6.jpg")
x := (maxx div 2 - 1) % Random x
y := (maxy div 2 - 1) % Random y
proc RollDie ()
loop
Pic.Draw (dice (1), x, y, picCopy)
delay (delayy )
Input.KeyDown (chars )
Pic.Draw (dice (2), x, y, picCopy)
delay (delayy )
Input.KeyDown (chars )
Pic.Draw (dice (3), x, y, picCopy)
delay (delayy )
Input.KeyDown (chars )
Pic.Draw (dice (4), x, y, picCopy)
delay (delayy )
Input.KeyDown (chars )
Pic.Draw (dice (5), x, y, picCopy)
delay (delayy )
Input.KeyDown (chars )
Pic.Draw (dice (6), x, y, picCopy)
end loop
end RollDie
proc Roll ()
loop
Input.KeyDown (chars )
exit when chars (chr (10))
RollDie
end loop
end Roll
Roll ()
|
I am Using Turing 4.1.1 |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
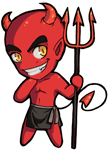
|
Posted: Thu Oct 29, 2009 11:26 am Post subject: RE:Dice Game |
|
|
you have the right idea for exiting the Roll loop. Now, how would you exit the RollDie loop? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
GamingFusion
|
Posted: Thu Oct 29, 2009 11:33 am Post subject: RE:Dice Game |
|
|
i tried that with a exit when chars (chr (10))
but it doesnt check always only when it is checking and thats in between each Pic.Draw |
|
|
|
|
 |
Tony
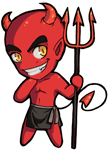
|
Posted: Thu Oct 29, 2009 11:43 am Post subject: RE:Dice Game |
|
|
you have no exit statement from the loop. Also, you are doing 6 different things in there. So either you will need to have 6 separate checks and exits (ugly) or figure out how to not have nested loops, and draw just one picture at a time (preferred solution).
Here's a hint
code: |
var i : int := 2
Pic.Draw (dice (i), x, y, picCopy)
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
GamingFusion
|
Posted: Fri Oct 30, 2009 9:45 am Post subject: Re: Dice Game |
|
|
OK i fixed it, the way did it was the way i should have done it in the first place
Heres my new code
Turing: |
% The "Pic.FileNew" program.
var dice : array 1 .. 6 of int
var x, y : int
const delayy := 100
var chars : array char of boolean
var die : int := 1
dice (1) := Pic.FileNew ("H:/Turing/Dice/Images/dice1.jpg")
dice (2) := Pic.FileNew ("H:/Turing/Dice/Images/dice2.jpg")
dice (3) := Pic.FileNew ("H:/Turing/Dice/Images/dice3.jpg")
dice (4) := Pic.FileNew ("H:/Turing/Dice/Images/dice4.jpg")
dice (5) := Pic.FileNew ("H:/Turing/Dice/Images/dice5.jpg")
dice (6) := Pic.FileNew ("H:/Turing/Dice/Images/dice6.jpg")
x := (maxx div 2 - 1) % Random x
y := (maxy div 2 - 1) % Random y
proc Roll ()
loop
Input.KeyDown (chars )
exit when chars (chr (10))
Pic.Draw (dice (die ), x, y, picCopy)
delay (delayy )
die + = 1
if die = 7 then
die := 1
end if
end loop
end Roll
Roll ()
|
|
|
|
|
|
 |
|
|