Author |
Message |
amir20001
|
Posted: Mon Mar 30, 2009 5:20 pm Post subject: need help with processes |
|
|
is there to make a processes return a value or effect a variable for example
code: |
var a :int
a:=0
process b
a:=5
end b
fork b
put a
|
would return a as 0 not 5 |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
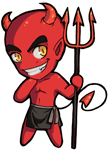
|
|
|
|
 |
Insectoid

|
Posted: Mon Mar 30, 2009 6:59 pm Post subject: RE:need help with processes |
|
|
Processes execute code pseudo-simultaneously with the other code. So 'put A' happens before process B can modify A. Procedures are similar, but they do not run at the same time. They run like regular code, one after the other. Functions are like procedures, but they return a value.
Also, post #1000! I can use da VIP lounge now! You should feel special that I thought this thread worthy of my 1000'd post. Not really. Unless you want to. |
|
|
|
|
 |
DemonWasp
|
Posted: Mon Mar 30, 2009 8:04 pm Post subject: RE:need help with processes |
|
|
Better question: WHY do you want a process to return a value? We can probably give you some other solution that's a bit more thread-safe. |
|
|
|
|
 |
amir20001
|
Posted: Mon Mar 30, 2009 9:18 pm Post subject: Re: need help with processes |
|
|
i'm sure i'm going about this wrong but i have to make a game don't wanna bore you with details but its pretty much a series of quick time events this the part i have for getting the keys pressed
code: |
var a: string
process chars
if hasch then
a:=getchar
put a
Input.Flush
end if
end chars
loop
delay (10)
fork chars
end loop
|
but i want to be able to compare the a value outside the process( if the key pressed is 'w' or something like that). the only way i can see this being done is by having this in the background constantly witch is why i used a process. if you can tell me how this can be done or let me know of a more efficient way of doing this.
thanks in advance |
|
|
|
|
 |
DanielG
|
Posted: Mon Mar 30, 2009 9:42 pm Post subject: RE:need help with processes |
|
|
just use a function in a loop, the loop should be repeating often enough and it won't give you the potential for errors due to it running simulatinously. The only thing you should use turing processes for is music. |
|
|
|
|
 |
amir20001
|
Posted: Mon Mar 30, 2009 9:52 pm Post subject: RE:need help with processes |
|
|
ok thx just one more question what is the difference between functions and procedure |
|
|
|
|
 |
Tony
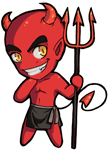
|
Posted: Mon Mar 30, 2009 10:20 pm Post subject: RE:need help with processes |
|
|
a procedure is a function that does not return a value. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
SNIPERDUDE

|
Posted: Tue Mar 31, 2009 11:19 am Post subject: RE:need help with processes |
|
|
example:
Turing: | % The procedure changes a global variable without returning a value
procedure test (stuff : int)
a := stuff
end test
% The function returns a value, but runs in a similar way to the procedure
function test2 (stuff : int) : int %<- the extra : int on the outside means it returns an integer value
% you can do calculations in here
result stuff * 2
end test2
% This also makes it easier for checking values within an if statement
if test2 (5) = 10 then
put "Grats!"
end if |
|
|
|
|
|
 |
DemonWasp
|
Posted: Tue Mar 31, 2009 12:48 pm Post subject: RE:need help with processes |
|
|
The standard design for a game actually doesn't use any processes. Playing music in Turing aside (stupid blocking API...), you don't need to use processes at all. It looks like this.
You will have a "main" game loop. Nothing in this loop "blocks", meaning nothing waits for input before proceeding. Anything you do returns immediately, with no delays. Basically, this means you can't use get from the screen, or hasch, or getch, or getchar, all of which will wait until the user gives input. This loop will go as fast as possible, often around 30-60 times per second. Each time, it will look for any input and will draw the complete scene.
The main game loop looks like this:
Turing: |
View.Set ( "offscreenonly" ) % This is used for double-buffered graphics. See the tutorial at http://compsci.ca/v3/viewtopic.php?t=12533
var keys : array char of boolean
loop
% Capture the current state of the keyboard - a set of boolean variables that are true if the key is currently pressed.
Input.KeyDown ( keys )
% Handle the input here. I don't know exactly what you need to do here for your game, but if your read the documentation for Input.KeyDown(), you should be able to figure it out.
% Draw the scene here. This probably involves a Draw.Cls() followed by some Pic.Draw() and Draw.Something() lines.
View.Update () % Draw all of our updates to the screen.
end loop
|
|
|
|
|
|
 |
|