Random Statements non repeating?
Author |
Message |
Fenrisulfr

|
Posted: Mon Mar 16, 2009 10:33 pm Post subject: Random Statements non repeating? |
|
|
Hi, I know there's array for generating random numbers without repeating the same number, but can anyone tell me how to apply that to statements? For example, I have 3 put statements and I want them to appear in random order without repeating.
Thanks! |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Zren

|
Posted: Mon Mar 16, 2009 10:45 pm Post subject: Re: Random Statements non repeating? |
|
|
Turing: |
%Put the random numbers into an array a.
for i : 1 .. 3
put a(i)
end for
|
:/ Is that what you need? |
|
|
|
|
 |
corriep
|
Posted: Mon Mar 16, 2009 10:46 pm Post subject: RE:Random Statements non repeating? |
|
|
What you could do is have an array of strings, swap them around a couple of times and then put them to the screen.
If you need me to go more in-depth just say the word  |
|
|
|
|
 |
Tony
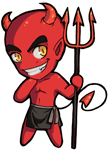
|
Posted: Mon Mar 16, 2009 11:06 pm Post subject: Re: RE:Random Statements non repeating? |
|
|
corriep @ Mon Mar 16, 2009 10:46 pm wrote: swap them around a couple of times
That does not produce a good random order. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Fenrisulfr

|
Posted: Mon Mar 16, 2009 11:12 pm Post subject: Re: Random Statements non repeating? |
|
|
I actually wanted to ask around 10 questions in random order lol, don't know if a string will hold
what I have no idea is how to assign each statement, or question in my case, to a number to be generated randomly
Can anyone give me an example of just like 2 put statements appearing in random order without repeating?
sry about my slow understanding just started turing, programming for that matter around 2 weeks ago so yah... |
|
|
|
|
 |
Tony
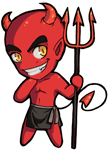
|
Posted: Mon Mar 16, 2009 11:16 pm Post subject: Re: Random Statements non repeating? |
|
|
Fenrisulfr @ Mon Mar 16, 2009 10:33 pm wrote: Hi, I know there's array for generating random numbers without repeating the same number
It sounds like you already know how to get a list of random numbers. Can you think of a way to map those numbers to statements that you want to display? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Fenrisulfr

|
Posted: Mon Mar 16, 2009 11:24 pm Post subject: Re: Random Statements non repeating? |
|
|
I didnt fully understand the concept so eh, I only found out how to get the list from your other post. I tried making each put statement a procedure and numbering the procedures but again, can't think of any way to "map" them on to the generated numbers  |
|
|
|
|
 |
Tony
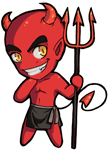
|
Posted: Mon Mar 16, 2009 11:33 pm Post subject: RE:Random Statements non repeating? |
|
|
look up tutorials for arrays in Turing Walkthrough. Although, it's not really a "2 weeks" material -- you might want to consult your teacher, to see what the expected approach is. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
copthesaint

|
Posted: Tue Mar 17, 2009 10:10 am Post subject: Re: Random Statements non repeating? |
|
|
If you want to do this efficiently then you may want to make a type.
Ex.
Turing: | type Names/*Can be any thing not just 'Names'*/ :
record /*Records the types for Names*/
FirstName,LastName : string /*There can be as many identifiers*/
end record /*There is no end*/ /*as you want and it doesn't just */
/*for type*/ /*have to be a string*/
var GetNames : Names /*This GetNames has the type of the identifiers*/
GetNames.FirstName:= "Joe" /*You should by now get what this is doing*/
GetNames.LastName:= "Blow"
put ("First Name: "+ GetNames.FirstName)
put ("Last Name: "+ GetNames.LastName)
|
Now what this can do is give a variable subscripts, witch is good to shorten code.
So what you may want to do first is make a type for your questions. Make sure you
have a Identifier that will be Boolean, a identifier that will be the string to
hold your question, and lastly another identifier that will hold your answer. Once you have
done this, look at this.
Turing: | type Names/*Can be any thing not just 'Names'*/ :
record /*Records the types for Names*/
FirstName,LastName : string /*There can be as many identifiers*/
end record /*There is no end*/ /*as you want and it doesn't just */
/*for type*/ /*have to be a string*/
var GetNames : array 1..2 of Names /*Now this an array of Name, and this is*/
GetNames(1).FirstName:= "Joe" /*where things get more complicated.*/
GetNames(2).FirstName:= "Sara"
GetNames(1).LastName:= "Blow"
GetNames(2).LastName:= "Bean"
put ("First Name: "+ GetNames(Rand.Int (1,2)).FirstName)/*See how It can Show*/
put ("Last Name: "+ GetNames(Rand.Int (1,2)).LastName) /*different Names? */ |
Now what you need to do is add a boolean statement. So if you have already made a boolean
identifier in your type then good. If you havn't then because you don't know how then look at this next example.
Turing: | type Numbers :
record
Number : int /*Any Name can be made for the identifiers*/
TRUEFALSE : boolean
end record
var GetNumber : array 1 .. 5 of Numbers /*Array of 5 Number*/
var Random, AllFalse : int /*Needed conditional ints*/
put "Please type any 5 different numbers"
for i : 1 .. 5 /*This gets all 5 numbers and set them to false*/
GetNumber (i).TRUEFALSE := false
get GetNumber (i).Number
end for
loop
AllFalse := 0 /*will be needed for the exit condition */
Random := Rand.Int (1, 5) /*Give the random Number*/
if GetNumber (Random).TRUEFALSE = false then /*Checks to see if the number has been used before*/
put ("Number_"), (Random), (" is "), (GetNumber (Random).Number) /*The put statment*/
GetNumber (Random).TRUEFALSE := true /*Makes sure that the question won't be asked again*/
end if
for i : 1 .. 5
if GetNumber (i).TRUEFALSE = true then /*Exit condition*/
AllFalse += 1
end if
end for
exit when AllFalse = 5
end loop
put "Done!" |
Now with what I've shown you I'm Sure you can make This program!
If there is still something you don't understand just ask, but don't ask
until you have read this once or twice. |
|
|
|
|
 |
Tallguy

|
Posted: Mon Mar 23, 2009 9:15 am Post subject: RE:Random Statements non repeating? |
|
|
@copthesaint
thats very good man, but for someone who is new @ programming, it can be a bit diffucult to understand
i think the tutorial Tony reconmended wouldf be best for a new programmer
thats just my opion . . |
|
|
|
|
 |
|
|