Bubble sorting?!
Author |
Message |
m84uily
|
Posted: Tue Mar 10, 2009 3:50 pm Post subject: Bubble sorting?! |
|
|
The question is commented in the code so here it is:
code: |
/* Question
The MEDIAN of an ordered set of numbers is the middle number if the set contains an odd number of elements. It is the average of the two middle numbers if the set contains an
even number of elements. Write a program to find the median of a set of numbers. Start by making a list of random numbers. Also choose the length of the list randomly.
Then sort it from largest to smallest. Display the original list and the sorted list, side by side, as well as the median. */
var num, flag, temp, count, a, b, c, d, e, f, g, h, j, k : int
count := 0
a := 0
b := 0
c := 0
d := 0
e := 0
f := 0
g := 0
h := 0
j := 0
k := 0
for i : 1 .. Rand.Int (1, 10)
count := count + 1
randint (num, 1, 9)
if count = 1 then
a := num
end if
if count = 2 then
b := num
end if
if count = 3 then
c := num
end if
if count = 4 then
d := num
end if
if count = 5 then
e := num
end if
if count = 6 then
f := num
end if
if count = 7 then
g := num
end if
if count = 8 then
h := num
end if
if count = 9 then
j := num
end if
if count = 10 then
k := num
end if
end for
var yes : array 1 .. count of int
var random : boolean := true
for last : 1 .. count %loops for the number of times the user specified
for i : 1 .. count - 1 %loops one less then user defined because its comaring #1 and #1+1, so it has to end at one less then usder defined because the program adds 1
if yes (i) > yes (i + 1) then %compares the first mark to the second mark
random := false %if the above statement is true the whole eqiuation is 'false' so it has to do it again, and again until it is 'true' so it ends
const temp := yes (i) %here the two numbers are swamped so that #1 becomes #2, #2 becomes #1, using a tempoary variable to store the first number so itrs not overwrittem
yes (i) := yes (i + 1) %see above
yes (i + 1) := temp %see above
end if
end for
exit when random %exit when this statement is true
end for
|
This is my first time using bubble sort and I'm really quite confused. I copied the bubble sort part from another post on the forums, and, not surprisingly it's not working out for me.
My first and foremost problem would be the error I get when I try to run this code " 'temp' has been previously declared" which I really don't know how to solve.
My second problem is, once I get bubble sort working I'm not entirely sure of how to display the numbers in the sorted order.
Thanks in advance and all help is very much appreciated. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Insectoid

|
Posted: Tue Mar 10, 2009 4:10 pm Post subject: RE:Bubble sorting?! |
|
|
What are the A, b, c, d, e... variables for?
the variable 'temp' is being declared more than once. You have
in there more than once, or in a loop/conditional.
First, make an variable to hold the array length.
code: |
var arrayLength : int := Rand.Int
|
Now, declare your array or random numbers.
code: |
var bleh : array 1..arrayLength of int
|
Use a for loop to initialize the array
now, a bubble sort works like so.
code: |
for x : 1..arrayLength
for y : 1..arrayLength - 1
if bleh (y) > bleh (y + 1) then
%swap bleh (y) with bleh (y+1)
end if
end for
end for
|
then use a for loop to display the result!
You'll have to figure out the %swap part yourself. |
|
|
|
|
 |
m84uily
|
Posted: Tue Mar 10, 2009 4:37 pm Post subject: Re: Bubble sorting?! |
|
|
code: |
var arrayLength : int := count
var bleh : array 1 .. arrayLength of int
for last : 1 .. arrayLength %loops for the number of times the user specified
for i : 1 .. arrayLength - 1 %loops one less then user defined because its comaring #1 and #1+1, so it has to end at one less then usder defined because the program adds 1
if bleh (i) > bleh (i + 1) then %compares the first mark to the second mark
random := false %if the above statement is true the whole eqiuation is 'false' so it has to do it again, and again until it is 'true' so it ends
const temp := bleh (i) %here the two numbers are swamped so that #1 becomes #2, #2 becomes #1, using a tempoary variable to store the first number so itrs not overwrittem
bleh (i) := bleh (i + 1) %see above
bleh (i + 1) := temp %see above
end if
end for
exit when random %exit when this statement is true
end for
|
I think I understand what you mean by swap, and I'm trying to use temp to do so. However at the line
code: | if bleh (i) > bleh (i + 1) then |
I receive the error "Variable has no value"
How can I fix this?
Also! variables a - k are so I can work with them outside the for, because the question asks me to display the unordered numbers also. |
|
|
|
|
 |
Tony
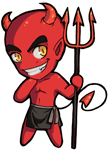
|
Posted: Tue Mar 10, 2009 5:12 pm Post subject: RE:Bubble sorting?! |
|
|
start by fixing your variable names. Then actually assign some value to it. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
m84uily
|
Posted: Tue Mar 10, 2009 5:36 pm Post subject: Re: Bubble sorting?! |
|
|
Quote: start by fixing your variable names. Then actually assign some value to it.
Well, if by fixing variable names you mean, they're silly, then I understand, otherwise I don't really. Could you please expand a little more on this?
And I'd assumed I'd assigned a value to variable bleh in the lines:
code: | var arrayLength : int := count
var bleh : array 1 .. arrayLength of int |
and count is defined in the previous for statement in the lines:
code: | for i : 1 .. Rand.Int (1, 10)
count := count + 1
randint (num, 1, 9) |
So, I'm not entirely sure where the missing value is, although I'm certain you're right as that is the problem.
Here is my code as a whole as it is now (including comments I have for myself):
code: |
/* Question
The MEDIAN of an ordered set of numbers is the middle number if the set contains an odd number of elements. It is the average of the two middle numbers if the set contains an
even number of elements. Write a program to find the median of a set of numbers. Start by making a list of random numbers. Also choose the length of the list randomly.
Then sort it from largest to smallest. Display the original list and the sorted list, side by side, as well as the median. */
var num, flag, count, a, b, c, d, e, f, g, h, j, k : int
count := 0
a := 0
b := 0
c := 0
d := 0
e := 0
f := 0
g := 0
h := 0
j := 0
k := 0
for i : 1 .. Rand.Int (1, 10)
count := count + 1
randint (num, 1, 9)
if count = 1 then
a := num
end if
if count = 2 then
b := num
end if
if count = 3 then
c := num
end if
if count = 4 then
d := num
end if
if count = 5 then
e := num
end if
if count = 6 then
f := num
end if
if count = 7 then
g := num
end if
if count = 8 then
h := num
end if
if count = 9 then
j := num
end if
if count = 10 then
k := num
end if
end for
var arrayLength : int := count
var bleh : array 1 .. arrayLength of int
for last : 1 .. arrayLength %loops for the number of times the user specified
for i : 1 .. arrayLength - 1 %loops one less then user defined because its comaring #1 and #1+1, so it has to end at one less then usder defined because the program adds 1
if bleh (i) > bleh (i + 1) then %compares the first mark to the second mark
random := false %if the above statement is true the whole eqiuation is 'false' so it has to do it again, and again until it is 'true' so it ends
const temp := bleh (i) %here the two numbers are swamped so that #1 becomes #2, #2 becomes #1, using a tempoary variable to store the first number so itrs not overwrittem
bleh (i) := bleh (i + 1) %see above
bleh (i + 1) := temp %see above
end if
end for
exit when random %exit when this statement is true
end for
/*
**to display numbers in order they were randomized**
if count = 1 then
put a
end if
if count = 2 then
put a, " ", b
end if
if count = 3 then
put a, " " , b ," ", c
end if
if count = 4 then
put a, " ",b, " ", c, " ", d
end if
if count = 5 then
put a, " ",b, " ", c, " ", d, " ", e
end if
if count = 6 then
put a, " ",b, " ", c, " ", d, " ", e, " ", f
end if
if count = 7 then
put a, " ",b, " ", c, " ", d, " ", e, " ", f, " ", g
end if
if count = 8 then
put a, " ",b, " ", c, " ", d, " ", e, " ", f, " ", g, " ", h
end if
if count = 9 then
put a, " ",b, " ", c, " ", d, " ", e, " ", f, " ", g, " ", h, " ", j
end if
if count = 10 then
put a, " ",b, " ", c, " ", d, " ", e, " ", f, " ", g, " ", h, " ", j, " ", k
end if
==SORT METHODS==
BUBBLE SORT
loop
flag := 0
for i : 1 .. n - 1
if name ( i ) > name (i + 1) then
temp := name (i + 1)
name (i + 1) := name ( i )
name ( i ) := temp
flag := 1
end if
end for
exit when flag := 0
end loop
VERY LONG IF STATEMENT SORT
if a > b and a > c and a > d and a > e and a >f and a > g and a > h and a > j and a > k then
a := a1
end if
if b > a and b > c and b > d and b > e and b > f and b >g and b > h and b > j and b > k then
b := a1
end if
if c > a and c > b and c> d and c > e and c > f and c >g and c > h and c > j and c > k then
c := a1
end if
if d > a and d > b and d> c and d > e and d > f and d >g and d > h and d > j and d > k then
d := a1
end if
etc...
*/
|
|
|
|
|
|
 |
Tony
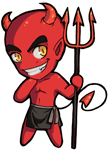
|
Posted: Tue Mar 10, 2009 5:43 pm Post subject: Re: Bubble sorting?! |
|
|
Yes, make your variable names meaningful. I don't know what you are talking about, when you use variable "bleh"
m84uily @ Tue Mar 10, 2009 5:36 pm wrote:
And I'd assumed I'd assigned a value to variable bleh in the lines:
code: | var arrayLength : int := count
var bleh : array 1 .. arrayLength of int |
What's the value of bleh(1) then? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
m84uily
|
Posted: Tue Mar 10, 2009 6:01 pm Post subject: Re: Bubble sorting?! |
|
|
Tony @ Tue Mar 10, 2009 5:43 pm wrote: Yes, make your variable names meaningful. I don't know what you are talking about, when you use variable "bleh"
m84uily @ Tue Mar 10, 2009 5:36 pm wrote:
And I'd assumed I'd assigned a value to variable bleh in the lines:
code: | var arrayLength : int := count
var bleh : array 1 .. arrayLength of int |
What's the value of bleh(1) then?
Thank you! I understand now. Heh, just took a simple question to make it click.
The sorter looks like this now:
code: |
var arrayLength : int := count
var bleh : array 1 .. arrayLength of int
if count = 1 then
bleh (1) := a
end if
if count = 2 then
bleh (1) := a
bleh (2) := b
end if
if count = 3 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
end if
if count = 4 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
bleh (4) := d
end if
if count = 5 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
bleh (4) := d
bleh (5) := e
end if
if count = 6 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
bleh (4) := d
bleh (5) := e
bleh (6) := f
end if
if count = 7 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
bleh (4) := d
bleh (5) := e
bleh (6) := f
bleh (7) := g
end if
if count = 8 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
bleh (4) := d
bleh (5) := e
bleh (6) := f
bleh (7) := g
bleh (8) := h
end if
if count = 9 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
bleh (4) := d
bleh (5) := e
bleh (6) := f
bleh (7) := g
bleh (8) := h
bleh (9) := j
end if
if count = 10 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
bleh (4) := d
bleh (5) := e
bleh (6) := f
bleh (7) := g
bleh (8) := h
bleh (9) := j
bleh (10) := k
end if
for last : 1 .. arrayLength %loops for the number of times the user specified
for i : 1 .. arrayLength - 1 %loops one less then user defined because its comaring #1 and #1+1, so it has to end at one less then usder defined because the program adds 1
if bleh (i) > bleh (i + 1) then %compares the first mark to the second mark
const temp := bleh (i) %here the two numbers are swamped so that #1 becomes #2, #2 becomes #1, using a tempoary variable to store the first number so itrs not overwrittem
bleh (i) := bleh (i + 1) %see above
bleh (i + 1) := temp %see above
end if
end for
end for
|
Which executes and such, although I'm not 100% it's working. I had to take out the random that use to be there, shown in the code here:
code: |
var yes : array 1 .. count of int
var random : boolean := true
for last : 1 .. count %loops for the number of times the user specified
for i : 1 .. count - 1 %loops one less then user defined because its comaring #1 and #1+1, so it has to end at one less then usder defined because the program adds 1
if yes (i) > yes (i + 1) then %compares the first mark to the second mark
random := false %if the above statement is true the whole eqiuation is 'false' so it has to do it again, and again until it is 'true' so it ends
const temp := yes (i) %here the two numbers are swamped so that #1 becomes #2, #2 becomes #1, using a tempoary variable to store the first number so itrs not overwrittem
yes (i) := yes (i + 1) %see above
yes (i + 1) := temp %see above
end if
end for
exit when random %exit when this statement is true
end for
|
Whether or not it needs to be there I'm not sure. Ultimately, to decipher if it's working properly I need to know how to display the ordered results? (Don't worry, I'll change the variables at the end Tony ) |
|
|
|
|
 |
m84uily
|
Posted: Tue Mar 10, 2009 6:24 pm Post subject: Re: Bubble sorting?! |
|
|
It can now execute with the random in it:
code: |
/* Question
The MEDIAN of an ordered set of numbers is the middle number if the set contains an odd number of elements. It is the average of the two middle numbers if the set contains an
even number of elements. Write a program to find the median of a set of numbers. Start by making a list of random numbers. Also choose the length of the list randomly.
Then sort it from largest to smallest. Display the original list and the sorted list, side by side, as well as the median. */
var num, flag, count, a, b, c, d, e, f, g, h, j, k : int
count := 0
a := 0
b := 0
c := 0
d := 0
e := 0
f := 0
g := 0
h := 0
j := 0
k := 0
for i : 1 .. Rand.Int (1, 10)
count := count + 1
randint (num, 1, 9)
if count = 1 then
a := num
end if
if count = 2 then
b := num
end if
if count = 3 then
c := num
end if
if count = 4 then
d := num
end if
if count = 5 then
e := num
end if
if count = 6 then
f := num
end if
if count = 7 then
g := num
end if
if count = 8 then
h := num
end if
if count = 9 then
j := num
end if
if count = 10 then
k := num
end if
end for
var arrayLength : int := count
var bleh : array 1 .. arrayLength of int
if arrayLength = 1 then
bleh (1) := a
end if
if arrayLength = 2 then
bleh (1) := a
bleh (2) := b
end if
if arrayLength = 3 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
end if
if arrayLength = 4 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
bleh (4) := d
end if
if arrayLength = 5 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
bleh (4) := d
bleh (5) := e
end if
if arrayLength = 6 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
bleh (4) := d
bleh (5) := e
bleh (6) := f
end if
if arrayLength = 7 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
bleh (4) := d
bleh (5) := e
bleh (6) := f
bleh (7) := g
end if
if arrayLength = 8 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
bleh (4) := d
bleh (5) := e
bleh (6) := f
bleh (7) := g
bleh (8) := h
end if
if arrayLength = 9 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
bleh (4) := d
bleh (5) := e
bleh (6) := f
bleh (7) := g
bleh (8) := h
bleh (9) := j
end if
if arrayLength = 10 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
bleh (4) := d
bleh (5) := e
bleh (6) := f
bleh (7) := g
bleh (8) := h
bleh (9) := j
bleh (10) := k
end if
var random : boolean := true
for last : 1 .. arrayLength %loops for the number of times the user specified
for i : 1 .. arrayLength - 1 %loops one less then user defined because its comaring #1 and #1+1, so it has to end at one less then usder defined because the program adds 1
if bleh (i) > bleh (i + 1) then %compares the first mark to the second mark
random := false
const temp := bleh (i) %here the two numbers are swamped so that #1 becomes #2, #2 becomes #1, using a tempoary variable to store the first number so itrs not overwrittem
bleh (i) := bleh (i + 1) %see above
bleh (i + 1) := temp %see above
exit when random = true
end if
end for
end for
/*
**to display numbers in order they were randomized**
if count = 1 then
put a
end if
if count = 2 then
put a, " ", b
end if
if count = 3 then
put a, " " , b ," ", c
end if
if count = 4 then
put a, " ",b, " ", c, " ", d
end if
if count = 5 then
put a, " ",b, " ", c, " ", d, " ", e
end if
if count = 6 then
put a, " ",b, " ", c, " ", d, " ", e, " ", f
end if
if count = 7 then
put a, " ",b, " ", c, " ", d, " ", e, " ", f, " ", g
end if
if count = 8 then
put a, " ",b, " ", c, " ", d, " ", e, " ", f, " ", g, " ", h
end if
if count = 9 then
put a, " ",b, " ", c, " ", d, " ", e, " ", f, " ", g, " ", h, " ", j
end if
if count = 10 then
put a, " ",b, " ", c, " ", d, " ", e, " ", f, " ", g, " ", h, " ", j, " ", k
end if
==SORT METHODS==
BUBBLE SORT
loop
flag := 0
for i : 1 .. n - 1
if name ( i ) > name (i + 1) then
temp := name (i + 1)
name (i + 1) := name ( i )
name ( i ) := temp
flag := 1
end if
end for
exit when flag := 0
end loop
VERY LONG IF STATEMENT SORT
if a > b and a > c and a > d and a > e and a >f and a > g and a > h and a > j and a > k then
a := a1
end if
if b > a and b > c and b > d and b > e and b > f and b >g and b > h and b > j and b > k then
b := a1
end if
if c > a and c > b and c> d and c > e and c > f and c >g and c > h and c > j and c > k then
c := a1
end if
if d > a and d > b and d> c and d > e and d > f and d >g and d > h and d > j and d > k then
d := a1
end if
etc...
*/
|
Still not sure how to display, though. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
m84uily
|
Posted: Tue Mar 10, 2009 6:39 pm Post subject: Re: Bubble sorting?! |
|
|
HAH! Got it! Hail Satan!
Here's the nearly completed code (still need to change variables and do some other stuff the question asks of me) :
code: |
/* Question
The MEDIAN of an ordered set of numbers is the middle number if the set contains an odd number of elements. It is the average of the two middle numbers if the set contains an
even number of elements. Write a program to find the median of a set of numbers. Start by making a list of random numbers. Also choose the length of the list randomly.
Then sort it from largest to smallest. Display the original list and the sorted list, side by side, as well as the median. */
var num, flag, count, a, b, c, d, e, f, g, h, j, k : int
count := 0
a := 0
b := 0
c := 0
d := 0
e := 0
f := 0
g := 0
h := 0
j := 0
k := 0
for i : 1 .. Rand.Int (1, 10)
count := count + 1
randint (num, 1, 9)
if count = 1 then
a := num
end if
if count = 2 then
b := num
end if
if count = 3 then
c := num
end if
if count = 4 then
d := num
end if
if count = 5 then
e := num
end if
if count = 6 then
f := num
end if
if count = 7 then
g := num
end if
if count = 8 then
h := num
end if
if count = 9 then
j := num
end if
if count = 10 then
k := num
end if
end for
var arrayLength : int := count
var bleh : array 1 .. arrayLength of int
if arrayLength = 1 then
bleh (1) := a
end if
if arrayLength = 2 then
bleh (1) := a
bleh (2) := b
end if
if arrayLength = 3 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
end if
if arrayLength = 4 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
bleh (4) := d
end if
if arrayLength = 5 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
bleh (4) := d
bleh (5) := e
end if
if arrayLength = 6 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
bleh (4) := d
bleh (5) := e
bleh (6) := f
end if
if arrayLength = 7 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
bleh (4) := d
bleh (5) := e
bleh (6) := f
bleh (7) := g
end if
if arrayLength = 8 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
bleh (4) := d
bleh (5) := e
bleh (6) := f
bleh (7) := g
bleh (8) := h
end if
if arrayLength = 9 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
bleh (4) := d
bleh (5) := e
bleh (6) := f
bleh (7) := g
bleh (8) := h
bleh (9) := j
end if
if arrayLength = 10 then
bleh (1) := a
bleh (2) := b
bleh (3) := c
bleh (4) := d
bleh (5) := e
bleh (6) := f
bleh (7) := g
bleh (8) := h
bleh (9) := j
bleh (10) := k
end if
var random : boolean := true
for last : 1 .. arrayLength %loops for the number of times the user specified
for i : 1 .. arrayLength - 1 %loops one less then user defined because its comaring #1 and #1+1, so it has to end at one less then usder defined because the program adds 1
if bleh (i) > bleh (i + 1) then %compares the first mark to the second mark
random := false
const temp := bleh (i) %here the two numbers are swamped so that #1 becomes #2, #2 becomes #1, using a tempoary variable to store the first number so itrs not overwrittem
bleh (i) := bleh (i + 1) %see above
bleh (i + 1) := temp %see above
exit when random = true
end if
end for
end for
for last : 1 .. arrayLength
put bleh (last)
end for
/*
**to display numbers in order they were randomized**
if count = 1 then
put a
end if
if count = 2 then
put a, " ", b
end if
if count = 3 then
put a, " " , b ," ", c
end if
if count = 4 then
put a, " ",b, " ", c, " ", d
end if
if count = 5 then
put a, " ",b, " ", c, " ", d, " ", e
end if
if count = 6 then
put a, " ",b, " ", c, " ", d, " ", e, " ", f
end if
if count = 7 then
put a, " ",b, " ", c, " ", d, " ", e, " ", f, " ", g
end if
if count = 8 then
put a, " ",b, " ", c, " ", d, " ", e, " ", f, " ", g, " ", h
end if
if count = 9 then
put a, " ",b, " ", c, " ", d, " ", e, " ", f, " ", g, " ", h, " ", j
end if
if count = 10 then
put a, " ",b, " ", c, " ", d, " ", e, " ", f, " ", g, " ", h, " ", j, " ", k
end if
==SORT METHODS==
BUBBLE SORT
loop
flag := 0
for i : 1 .. n - 1
if name ( i ) > name (i + 1) then
temp := name (i + 1)
name (i + 1) := name ( i )
name ( i ) := temp
flag := 1
end if
end for
exit when flag := 0
end loop
VERY LONG IF STATEMENT SORT
if a > b and a > c and a > d and a > e and a >f and a > g and a > h and a > j and a > k then
a := a1
end if
if b > a and b > c and b > d and b > e and b > f and b >g and b > h and b > j and b > k then
b := a1
end if
if c > a and c > b and c> d and c > e and c > f and c >g and c > h and c > j and c > k then
c := a1
end if
if d > a and d > b and d> c and d > e and d > f and d >g and d > h and d > j and d > k then
d := a1
end if
etc...
*/
|
|
|
|
|
|
 |
Tony
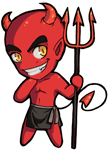
|
Posted: Tue Mar 10, 2009 6:39 pm Post subject: RE:Bubble sorting?! |
|
|
I'm not quite sure -- what are you trying to display (and where, in your program, would that data be found)? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Insectoid

|
Posted: Tue Mar 10, 2009 7:37 pm Post subject: RE:Bubble sorting?! |
|
|
To display the list in original order, just us a for loop and put statements before you sort it. |
|
|
|
|
 |
Tallguy

|
|
|
|
 |
|
|