Assistance on some 'loop' and 'for loop' questions, please.
Author |
Message |
Godess The Hyper Fangirl
|
Posted: Tue Mar 10, 2009 12:03 pm Post subject: Assistance on some 'loop' and 'for loop' questions, please. |
|
|
There are a few questions I have been assigned that I am having some issues with, so I hope someone can help. Keep in mind these are questions from An Introduction to Programming in Turing, which is the textbook from my grade 10 Programming class. So if you start getting extremely complicated it may or may not be helpful And yes, I did read the tutorial but it didn't do much for me. Feel free to delete/lock/move/whatever if this is not appropriate. There are 6 questions(out of 16, not including part a's and b's) I need assistance with, however any help on any question is appreciated.
Q: Write a program to output a table of values of intergers starting at 1 and their squares. Label the table at the top of the columns.
I have done this part like so:
Quote: put "Number", " ", " ", " ", " ", "Square"
var num:int:=0
var square: int
loop
put num
num:= num + 1
square:=num*num
put square:13
delay(500)
exit when num = 101
end loop
However, there is a part b: Modify the program so that in one for loop you output a 8 row table, with 'num' and 'square' like you did before. The first row will start at 1 and continue to 20, the second will start at 21 and continue to 40, and the third will start at 61 and end at 80, each group with their appropriate squares beside it. I have no idea where to start with this one.
Q: Write a program to compute the bank balance at the end of each year for 10 years, resulting from an initial deposit of $1000 and an annual interest rate of 6%. Output for each year end the number of the year, the initial balance, the interest for the year, and the balance at the end of the year.
Currently, I have this:
Quote: var interest, initial, balance : real
initial:=1000
interest:=0.06
put "Here is the interest of an initial depost of $1000 at 6% over 10 years."
for count: 1 .. 10
put count, " ", count * initial * interest
end for
The issue here is I don't know how to go about putting in all of the information that they want.
Q: A homeowner takes out a mortgage for $120 000 at 7.75% per year. At the end of each year and amount of $24 000 is payed. Write a program to show how the mortgage is paid off, year by year, until nothing is owing.
Currently, I have this:
Quote: const interest:=0.0775
const payment:=2400
var left:int:=0
put "This is how an interst of $120 000 at 7.75%,"
put "being paid at $24 000 a year gets paid."
put " "
loop
put left
left:=(interest*120000)+(12000-payment)
delay (500)
exit when left=0
end loop
However, no matter what goes on the end of 'left:=' It tells me the assigned value is the wrong type and I cannot get it to tell me otherwise. I cannot do part b (assume that each month in the year has 30.5 days in it. Give the number of the month and the day in the month which the mortgage is paid), which also seems a bit difficult(so if you can help with that bit, that would be great), until I get past the first part.
Q:Write a program to simulate the playing of a simple dice game (played with one die). Roll the die to get a value from 1 to 6. This we will call your point. Now keep rolling until you get the same value (your point) again and see how many rolls it takes. Program it so you can play this game repeatedly.
Currently I have:
Quote: var roll, num, rolled, ans : int
var reply : string (1)
put "Roll the dice! It will give you a number between 1 and 6."
put "Then, roll to see how many tries it takes to get that number again"
loop
var count : int := 0
put "Do you want to play? Answer y or n."
get reply
exit when reply = "n"
randint (num, 1, 6)
loop
put "Enter 'roll' to roll" ..
get ans
if ans="roll" then
count := count + 1
if num < rolled then
put "You are low"
elsif num > rolled then
put "You are high"
else
put "you got it in ", count, " guesses"
exit
end if
end if
end loop
end loop
at 'if ans="roll" then' it tells me 'Operands of comparison operators must be of the same type.' But yet again, no matter how much I change things around the error message remains unchanged. I assumed this program would be very similar to the Guess Number program, which looks like this(this is in the book):
Quote: var hidden, guess : int
var reply : string (1)
put "See if you can guess the hidden number"
put "It is between 1 and 99 inclusive"
loop
var count : int := 0
put "Do you want to play? answer y or n " ..
get reply
exit when reply = "n"
%Choose a random number between 1 and 99
randint (hidden, 1, 99)
loop
put "Enter your guess (any number between 1 and 99) " ..
get guess
count := count + 1
if guess < hidden then
put "You are low"
elsif guess > hidden then
put "You are high"
else
put "you got it in ", count, " guesses"
exit
end if
end loop
end loop
The only difference being that the number is not hidden.
Q: Write a program to keep inputting integers until a perfect square(for example 64) between 40 and 100 is entered(This is a difficult one!)
Currently I have:
Quote: var num, x, y : real
loop
get num
x := sqrt (num)
y := round (x)
exit when
end loop
I don't know how to go about their between 40 and 100 qualification.
Q: Write a program to generate 10 random real numbers between x and y, where x and y are integer inputs.
*Note, I was also asked to make it work for a through c, which are
4 and 5
0 and 10
20 and 30
Currently I have:
Quote: var num1, num2 : int
var numnum : int
var reply : string (1)
put "Enter two variables. Please enter variable one."
get num1
put "Please enter variable 2."
get num2
for count: 1 .. 10
randint (numnum, num1, num2)
put count, numnum
end for
The issue I have here is that it generates random numbers, but not between my inputs. Also, because I cannot declare them as reals if I put 4 and 5 it will not work properly.
Thanks! |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
DemonWasp
|
Posted: Tue Mar 10, 2009 2:07 pm Post subject: RE:Assistance on some \'loop\' and \'for loop\' questions, please. |
|
|
Program #1: There's a slight problem that I see: the output is wrong. 0 ^ 2 != 1, 1 ^ 2 != 4, etc. You need to move the num := num + 1 bit to just before the "end loop". Alternately, you could learn the very useful "for" loop:
Turing: |
for num : 1 .. 100
% Do your stuff here. You can do this in a single put statement, see below.
end for
|
It would also be helpful to know that there's better ways of outputting stuff. For integers, you can use the following syntax to force them to use up a given number of spaces and to be right-aligned within that:
Turing: |
var myInteger : int := 37
put myInteger : 12
|
produces:
code: | 37 (note: there are 10 spaces there) |
You also don't have to do this:
Turing: |
put "Number", " ", " ", " ", " ", "Square"
|
instead you can do:
Turing: |
put "Number Square"
|
You can also get your program to put both the number and its square on the same line if you use commas in your put statement, though I'll leave the how of that to you.
Part B requires that you know the following:
Turing: |
put "My name is "..
put "Fred"
|
gives "My name is Fred". The .. part tells Turing that you don't want to end the line just yet.
Question 2:
Your solution is nearly right, but you need to think more about what math needs to be done to get the result. Here's a clearer problem statement:
At the end of every year, the balance of the account increases by the interest rate. For example, $1000 at 6% should give the following values: 1060, 1123.6, 1191.02, 1262.48.
The interest for each year is the amount of money gained. The balance at the end of the year will be the balance from the beginning of THAT year, plus the interest for that year.
Question 3:
This will become easier after you get Q2 down. The reason it's telling you you have the wrong type is because Turing has assumed that you wanted "left" to be an integer, because you didn't tell it what "left" was.
Turing: |
% If you do this, Turing knows exactly what you mean:
var myInteger : int := 5
var myReal : real := 3.1415
% If you do this, Turing has to guess:
var myInteger := 5 % Turing correctly assumes this is an integer
var myReal := 3.1415 % Turing correctly assumes this is a real number
var cost := 15 % Even though you meant 15 dollars, or 15.00, Turing assumes you meant an integer, which isn't right.
|
To be safe, ALWAYS specify the types of your variables (in Turing; other languages have different rules).
Question 4:
The first big problem here is that you're trying to compare the integer in the variable 'ans' to the string "roll". This isn't what you want to do. Read the problem statement again, carefully. You've got the right idea with the loop - end loop around the program (so it can be repeated), but the inside needs some work.
Here's the problem statement, simplified: Roll a first die. Count the number of rolls on a second die until they are equal.
Question 5:
This is where you have to think about what you need to do at each step. So far, you've:
1. Gotten the number from the user (num).
2. Determined the square root of the number.
For step 3, you need to check whether the input (num) was a perfect square. You can do this in a few different ways; try a few examples on a piece of paper and you should be able to figure it out.
For step 4, you need to check whether the input (num) was between 40 and 100. For this, you can use something like:
Turing: |
if num > 39 and num < 101 then
% Do some stuff in here...
% ...
end if
|
Question 6:
Actually, this program is working right, except that its output is confusing. You are outputting count, followed by the random number, and it looks like they're all one (much larger) number.
For random numbers, you need to use rand. Better yet, you should be using the following instead:
Turing: |
var myRandomInteger : int := Rand.Int ( minimum, maximum )
var myRandomReal : real := Rand.Real()
|
Rand.Real() and rand() will give you a number between 0 and 1 (including 0, but not 1). You need to figure out how to get that to cover the range given (think about it, try it out on paper).
For future reference:
CompSci.ca supports an extension to BBCode: Syntax tags! You can use the following to highlight your code properly
[ syntax = "Turing" ] [ / syntax ]
(minus all the spaces). Use these instead of code tags where appropriate.
Please indent your code properly. Press F2 in Turing and Turing will do it for you. It makes the code much, much easier to read and write, and makes it easier for people to help you (which means they're more likely to). |
|
|
|
|
 |
Tony
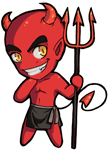
|
Posted: Tue Mar 10, 2009 2:11 pm Post subject: RE:Assistance on some \'loop\' and \'for loop\' questions, please. |
|
|
1. you can use .. to keep output on the same line
code: |
put "one "..
put "two"
|
2. you already figured out how much interest is earned, so just add that to the balance.
3. what variable type is "left"? what variable type is the result of the expression?
also, are you sure that "left" will end up exactly 0?
4. once again, what variable type is "ans"?
5. you should compare "num" to 40 and 100
6. Rand.Real |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
|
|