Author |
Message |
x30Jx
|
Posted: Mon Feb 23, 2009 9:03 am Post subject: how to guarantee non-matching Rand.Ints ? |
|
|
Working on a program for school and I need to create a poker hand. However, a deck cannot contain more than one card of a king (ie. NOT 2x Jack of Spades). I need to input some kind of code to ensure that my two Rand.Int statements will NOT repeat values.
Any help?
Many Thanks. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
DemonWasp
|
Posted: Mon Feb 23, 2009 9:18 am Post subject: RE:how to guarantee non-matching Rand.Ints ? |
|
|
The simplest way is to just keep track of the values you've already generated. When you generate each number (card) after the first, check against all the previous cards to make sure it's not equal. If it isn't, add it to the hand; if it is, discard it and try again. |
|
|
|
|
 |
x30Jx
|
Posted: Mon Feb 23, 2009 9:26 am Post subject: RE:how to guarantee non-matching Rand.Ints ? |
|
|
NICE SIG MAN!!
but uh.... how do I discard and try again? can you post an example? |
|
|
|
|
 |
Zren

|
Posted: Mon Feb 23, 2009 9:31 am Post subject: Re: how to guarantee non-matching Rand.Ints ? |
|
|
You could also have the deck contain cards 1-52 in order then randomly swap card a with b lots of times. Then have your person draw from there. This helps if your game has a deck since you just have to keep the remainder in the array. This way the deck won't double either. |
|
|
|
|
 |
DemonWasp
|
Posted: Mon Feb 23, 2009 10:12 am Post subject: RE:how to guarantee non-matching Rand.Ints ? |
|
|
Zren's solution is actually better if you're going for a card game. If this is just an assignment - "generate a random poker hand" - then my way will be easier.
Sample code:
code: |
var cards : array 1..5 of int % Or some other number, I don't play poker
var card, numCards : int := 0
loop
% Randomly choose a card
card := Rand.Int ( 1, 52 )
% Check all the other cards to make sure we aren't duplicating any of them...
var duplicate : boolean := false
% You'll need to figure out this part for yourself
% If the card isn't already in the hand, then we can add it (and remember to increase the number of cards we have in the hand). If not, we ignore it and try again at the top of the loop.
if not duplicate then
numCards += 1
cards ( numCards ) := card
end if
% We're done when we hit 5 cards (assuming that's how many you have in a poker hand...)
exit when numCards = 5
end loop
|
|
|
|
|
|
 |
Zeroth
|
Posted: Mon Feb 23, 2009 12:58 pm Post subject: Re: how to guarantee non-matching Rand.Ints ? |
|
|
Theres actually a few bugs in DemonWasp's code.
1) numCards is incremented before its used for the first card will be blank.
2) doesn't Rand.int generate a number between 1 and 52, but not including 52? Then we'd need to increase it to 53.
Now, about generating a hand. Easiest way is to enumerate an array of 52 consecutive integers, then generate a random index. Remove the card by making that card at that index 0. Then it becomes extremely easy to check if its a duplicate card, without an O(n) speed algorithm. Shouldn't be more than 4-5 lines. |
|
|
|
|
 |
Tony
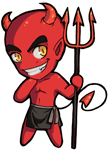
|
Posted: Mon Feb 23, 2009 1:04 pm Post subject: Re: how to guarantee non-matching Rand.Ints ? |
|
|
Zeroth @ Mon Feb 23, 2009 12:58 pm wrote: it becomes extremely easy to check if its a duplicate card
You don't even need to check for duplicates, if the index is selected from a set of cards not yet chosen. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
DemonWasp
|
Posted: Mon Feb 23, 2009 1:08 pm Post subject: RE:how to guarantee non-matching Rand.Ints ? |
|
|
@Zeroth: Arrays are indexed from 1 in Turing, numCards is initialised to 0, so the increment should happen first. Secondly, Rand.Int(a, b) returns a random integer in the range [ a, b ], not [ a, b ).
Yes, your method is better in general, but it sounds like this is an introductory assignment, which is why I went for the simpler route. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
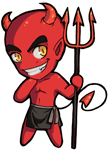
|
Posted: Mon Feb 23, 2009 1:22 pm Post subject: Re: RE:how to guarantee non-matching Rand.Ints ? |
|
|
DemonWasp @ Mon Feb 23, 2009 1:08 pm wrote: @Zeroth: Arrays are indexed from 1 in Turing
Clearly, arrays are indexed from 2
Turing: |
var foo : array 2 .. 3 of string := init("first", "last")
put foo(2)
|
 |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
DemonWasp
|
Posted: Mon Feb 23, 2009 1:49 pm Post subject: RE:how to guarantee non-matching Rand.Ints ? |
|
|
Yes yes - I guess you could also index from 0 or -1 or whatever you'd like, really. Point stands though because my code clearly has the array starting at 1. |
|
|
|
|
 |
Zeroth
|
Posted: Mon Feb 23, 2009 3:37 pm Post subject: Re: how to guarantee non-matching Rand.Ints ? |
|
|
Apologies. Like I said, I don't know Turing. They looked like bugs to me, because that behaviour defies everything pretty much every C-based language does  |
|
|
|
|
 |
Insectoid

|
Posted: Mon Feb 23, 2009 4:17 pm Post subject: RE:how to guarantee non-matching Rand.Ints ? |
|
|
What I did for my shuffler was have a second array and go through the deck, assigning each cord to a random unused index in the second array. I think I like the swap method more, reverse bubble sort .
EDIT: Demonwasp, your code is initializing a variable in a loop. Did you run before posting? or was this hastily typed straight into the post? . I know, I've done it too. |
|
|
|
|
 |
x30Jx
|
Posted: Fri Feb 27, 2009 9:11 am Post subject: RE:how to guarantee non-matching Rand.Ints ? |
|
|
wow. I cannot believe that was so easy... you know how it is though when you get turing blocks. lol  |
|
|
|
|
 |
Clayton

|
Posted: Fri Feb 27, 2009 11:37 am Post subject: RE:how to guarantee non-matching Rand.Ints ? |
|
|
Personally I like the method of just removing the card that you've picked from the array entirely, and then just picking from the new array that you get. |
|
|
|
|
 |
|