Author |
Message |
Tony
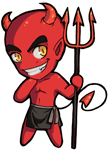
|
Posted: Fri Dec 27, 2002 11:39 pm Post subject: (No subject) |
|
|
by using chr() and ord() functions
basically
chr(ord("a")) will output "a"... now if you change it to chr(ord("a")+1) it will output "b" because of that +1
if you change that +1 to something more complex... like a math expression or something, you can get a less guessable encription. Make sure that ord(character) + expression is no greater then 255 and not less then about 15-20 or so (some characters are not printable).
You can obtain an ASCII value of any character by using
ord(character)
and convert it back to character using
chr(ASCII value)
Edit: wow, server's timing is off... or something... anyway, this suppost to be a reply |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
azndragon
|
Posted: Fri Dec 27, 2002 11:43 pm Post subject: Encoding Save File |
|
|
code: |
var text:string
var codeword : string
put "enter a word"
get text
for i:1..length(text)
put chr(ord(text(i))+5)..
end for
[Insert something that sets the converted value to variable codeword]
put "Coded word: ",codeword,"" |
Let's say I want to encrpyt a string with the above code. How would I use this code so that the converted text is set as another variable?
Ex. "Enter a word" hi
Coded word: mn |
|
|
|
|
 |
azndragon
|
Posted: Fri Dec 27, 2002 11:44 pm Post subject: (No subject) |
|
|
I know how to do it, but I'm just missing the part where the encoded value is set as another variable. Once that's set, I can get my entire encryption to work. |
|
|
|
|
 |
Tony
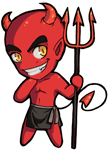
|
Posted: Fri Dec 27, 2002 11:48 pm Post subject: (No subject) |
|
|
in that case it should be something like this:
code:
var text : string := "tony"
var textnew : string := ""
for i:1.. length(text)
textnew := textnew + chr(ord(text(i))+1)
end for
put textnew
--------
textnew now holds "upoz" which will result back to "tony" if +1 is replaced with an opposite action (-1) |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Izzy
|
Posted: Fri Dec 27, 2002 11:49 pm Post subject: (No subject) |
|
|
Take a look at this
code:
var text:string
var codeword : string := ""
var newletter : string
put "enter a word"
get text
for i:1..length(text)
newletter := chr(ord(text(i))+5)
codeword := codeword + newletter
end for
put "Coded word: ",codeword,""
-------
- Izzy
Edited by Tony: hmm... '['code']' doesn't seem to work anymore... So I replaced it to make text visible
Edited by Tony again : nevermind... I think its just for me...  |
|
|
|
|
 |
azndragon
|
Posted: Sat Dec 28, 2002 12:16 am Post subject: (No subject) |
|
|
Thanks for the help guys. Okay, let me make sure I have this right before I start, because it's a pretty time consuming process to encrypt and decrypt 100+ variables . First, I will start off with the declaring variables statement.
code: | var character_name : string := ""
var character_name_code : string := ""
var character_agility : int
var character_agility_code : string
|
Encrypting Text
code: | for i : 1 .. length (character_name)
character_name_code := character_name_code + chr (ord (character_name (i)) + 8)
end for
|
Encrypting Numbers
code: | character_agility_code := chr (character_agility - 3)
|
Decrypting Text
code: | for i : 1 .. length (character_name_code)
character_name:= character_name + chr (ord (character_name_code (i)) - 8)
end for
|
Decrypting Numbers
code: | character_agility:= ord (character_agility_code)
character_agility := character_agility + 3
|
|
|
|
|
|
 |
Tony
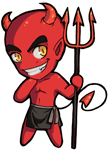
|
Posted: Sat Dec 28, 2002 12:22 am Post subject: (No subject) |
|
|
ya, you got that right...
though if you have 100+ variables, I sujest making a function instead of rewriting the code 100+ times  |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
azndragon
|
Posted: Sat Dec 28, 2002 10:02 am Post subject: (No subject) |
|
|
How would I use a function to encrypt multiple variables? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
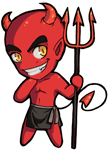
|
Posted: Sat Dec 28, 2002 1:39 pm Post subject: (No subject) |
|
|
you write a function to encript string
something like
code: |
function encript(text:string):string
var textNew:string :=""
for i : 1 .. length (text)
textNew:= textNew+ chr (ord (text(i)) + 8)
end for
result textNew
end encript
|
now just call this function every time you need to encript something, such as
put encript("tony")
or
var_text_encripted := encript(var_text) |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
azndragon
|
Posted: Sat Dec 28, 2002 1:42 pm Post subject: (No subject) |
|
|
code: | var character_skill2_uses : int := 0
var character_skill2_uses_code : string |
I'm getting a error:
"Value passed to 'chr' is <0 or > 255." under the line of:
code: | character_skill2_uses_code := chr (character_skill2_uses + 70) |
It works when I run it by itself in a separate file, but when I try to add it to my RPG, it gives that error. |
|
|
|
|
 |
Tony
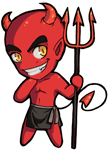
|
Posted: Sat Dec 28, 2002 10:00 pm Post subject: (No subject) |
|
|
thats because there're only 255 ASCII characters... so if your skill_level + 70 adds up to more then 255, there's no character with that value and nothing can be outputed.
thats why I worrned you to make sure that after math you result is >0 and <256 |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
azndragon
|
Posted: Sun Dec 29, 2002 10:04 am Post subject: (No subject) |
|
|
Yeah, I was aware of that. In the beginning of my RPG, you only get a level in 1 skill, which is character_skill, and the other skills, character_skill2, character_skill3, etc, are all set to 0. Obviously, a 0 would cause some errors in the ASCII conversion, so I added 70 to it, so that the output would be more practical. Problem is, it crashes in my game, but works when it's by itself with no other commands. Weird. If you want, I can send it to either you or Dan. |
|
|
|
|
 |
Tony
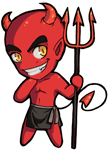
|
Posted: Sun Dec 29, 2002 5:32 pm Post subject: (No subject) |
|
|
if you can catch me online on MSN, you can send it over. Or try Dan... I haven't talked to him in a while though. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sie_Kensou
|
Posted: Sat Jan 04, 2003 8:36 pm Post subject: Weak Encryption? |
|
|
Hmm, the whole point of the encryption of the variables was because it's suppose to "hide" the value, right?
Using the Caesar Shift encryption you are using (that's what is called if I am not mistaken) is quite weak... could be decoded in less than a minute with another program (or maybe even guessing). |
|
|
|
|
 |
Tony
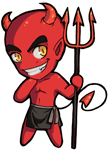
|
Posted: Sat Jan 04, 2003 9:18 pm Post subject: (No subject) |
|
|
thats true... and I think you're right about the name
but this is also the easiest encription to understand.
I mean come on, if we set up a 1024 bite encription (that is, output is 1024 times larger then input) + additional 512 bite control number against number guessing... I mean noone gonna break the code, but its not as easy to understand  |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
|