Big huge frigging problem
Author |
Message |
rcbhs
|
Posted: Thu Dec 18, 2008 1:15 pm Post subject: Big huge frigging problem |
|
|
So we are trying to make a FF/Zelda style game here. Now what we did to clean up our code is:
We made seperate files for every procedure (well every relaated procedure) and a seperate file for every variable in the game. Now, we are running into issues. For example:
We are running procedure A, then within procedure A we run procedure B. Then within procedure B we need to run procedure A. Now our issues is we cant call some of these again because well, they don't exist yet. So our issue is...is there a way to have acess to all procedures at any point, regardles of when it was delcared. IE.
code: |
GUI.createbutton ( 1, 1,"string", A)
procedure A
B
end A
procedure B
loop
move char
exit when collision
end loop
C
end B
procedure C
battleScreen
GUI.Button (1,1,"runFromBattle", B)
end C
|
Now I am not sure if this makes total sense. Regardless our problem is that we have multiple procedures that need to run off of each other, however we run into problems because we need to run certain ones at ceritain times and no matter where in the code we place them we get errors of them not being declared. We can't simply place them in new spots because then other procedures become un declared.
Anyways, I've read a bit about body procedures and forwarding and such but not entirely sure how it works. I've also looked at using classes and pointers to do it but again not sute entirely how it works.
If anybody could offer me some advice or help I would really appreciate it.
[/code] |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
DemonWasp
|
Posted: Thu Dec 18, 2008 1:30 pm Post subject: RE:Big huge frigging problem |
|
|
You're on the right track with body functions and forward declarations. The problem you're encountering is that you need both A and B to be declared before you can define the contents of either of them, since each calls the other (or a similar problem with more functions. It boils down to the same problem).
It works like this:
1. Declare A. This tells the Turing interpreter what a call to procedure / function A looks like. It gives the method name, argument list, and return type, but no implementation details (it's just like writing a regular procedure, but without the body:
Turing: | forward procedure A( var someArg: real ) |
2. Declare B. This is similar to step 1.
3. Define B. This can be done together with step 2, since your normal method definition also defines the method. For example:
Turing: | procedure B ( var someArg: real )
...uses A() somehow...
end B
|
OR
Turing: | body procedure B % with a corresponding forward procedure declaration above
...uses A() somehow...
end B
|
4. Define A. Now that we have declared B, we can use it in the method body for A. This looks like:
Turing: | body procedure A
...uses B() somehow...
end A
|
The technical term for this is "Declaration Before Use" (DBU) meaning that you must tell the interpreter or compiler what a function LOOKS like before using it, though you don't necessarily have to tell it WHAT it does (defining the function). |
|
|
|
|
 |
Tony
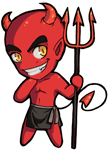
|
Posted: Thu Dec 18, 2008 1:39 pm Post subject: RE:Big huge frigging problem |
|
|
you are looking to use forward.
Although if I understand your example correctly, then you are doing it wrong.
You have a procedure to "walk around" (B) and sometimes you may encounter a battle (C), except that you can run from the said battle and spawn a new "walk around" procedure inside the existing battle (which in turn is inside that original walk procedure)(instead of simply exiting the battle and returning to the previous walk). |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
|
|