Author |
Message |
dc116
|
Posted: Fri Dec 12, 2008 9:00 pm Post subject: How do you output the number and sum of the digits? |
|
|
Ask the user for an integer. Output the number of digits in the integer. Then output the sum of the digits. (i.e. 1234 has 4 digits and their sum is 10)
Any help would be greatly appreciated.  |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
The_Bean

|
Posted: Fri Dec 12, 2008 9:12 pm Post subject: Re: How do you output the number and sum of the digits? |
|
|
Make an attempt at it before asking a question about it, and show us what you have done so far.
You'll need to use:
|
|
|
|
|
 |
Dan
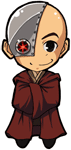
|
Posted: Fri Dec 12, 2008 9:15 pm Post subject: RE:How do you output the number and sum of the digits? |
|
|
Well i would do it by inputing the number as a string. Then the number of digits (assuming the input is vaild) is the length of the string.
Finding the sum is just a matter of looping threw the contents of the string and adding them to a counter. |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
ecookman

|
Posted: Fri Dec 12, 2008 9:16 pm Post subject: RE:How do you output the number and sum of the digits? |
|
|
hehe as i was kinda saying before on the other thread...another thing to keep in mind when asking for help try it yourself (yes,yes i am not the most faithful a that either) saves you from people saying well what do you have done so far.
if you have no clue ask what would i need to do this or could someone start me off.
you are lucky that The_Bean did so usually you get the first line |
|
|
|
|
 |
dc116
|
Posted: Fri Dec 12, 2008 10:46 pm Post subject: Re: How do you output the number and sum of the digits? |
|
|
This is what I have so far.
code: |
var number : string
var counter : int
counter := 0
put "Please enter an integer."
get number
put ""
put length(number), " digits"
|
I'm not sure how to use intstr() and strint() and counters to get the sum.
Can someone give me a hint on that part? |
|
|
|
|
 |
Tony
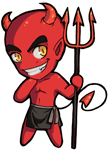
|
Posted: Fri Dec 12, 2008 11:01 pm Post subject: RE:How do you output the number and sum of the digits? |
|
|
given an integer, how would you get a digit in it's Nth place?
Here's an example: the number is 123. What is the digit in the 10s place? It's 2. Try to explain how you got to that answer though.
If you are simply reading "123" as a word, and taking a specific "letter" in the proper place (finding that place by counting the number of letters), then you'll find intstr() and strint() useful.
If you are using Math to isolate the digit, then div, mod and powers of 10 are helpful tools. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
dc116
|
|
|
|
 |
Tony
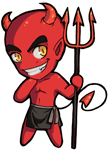
|
Posted: Sat Dec 13, 2008 9:25 pm Post subject: RE:How do you output the number and sum of the digits? |
|
|
Use better variable names. When I'm reading "a" or "p", I have no idea what they are. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
DanielG
|
Posted: Sat Dec 13, 2008 11:12 pm Post subject: RE:How do you output the number and sum of the digits? |
|
|
I believe a slightly more difficult version of this question was asked as the second question of the first dwite this year, you could look there for ideas on how to make yours better. |
|
|
|
|
 |
dc116
|
Posted: Sun Dec 14, 2008 6:16 pm Post subject: Re: How do you output the number and sum of the digits? |
|
|
Thanks for the help everyone. This is my final version.
code: |
var digits : int
var number : string
put "Please enter an integer."
get number
put ""
digits := length(number)
put digits, " digits"
var digit_value,counter1,counter2 : int
counter1 := strint(number)
counter2 := 0
for decreasing count: digits..1
digit_value := counter1 div (10**(count-1))
counter2 := counter2 + digit_value
counter1 := counter1 - (10**(count-1))*digit_value
end for
put "The digits sum is ",counter2
|
|
|
|
|
|
 |
|