History in a text file
Author |
Message |
WooFerPPK

|
Posted: Wed Dec 18, 2002 4:29 pm Post subject: History in a text file |
|
|
Im making a Quiz program. I would like to make a text file that keeps track of all the scores at the end.
How would i be able to make turing create a .txt file, make it read it and put the name and score on the screen ranging from the best to the worst? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
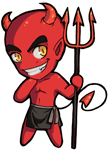
|
|
|
|
 |
FizixMan
|
Posted: Wed Dec 18, 2002 5:17 pm Post subject: (No subject) |
|
|
regarding file input/output, you need a couple things:
1) variables to store the file number (an integer; you use this number to tell turing which file to use), a file name (a string; the path and name of the file)
2) you need an "open" statement along with some extra parameters to tell turing what you'll be doing with that file (like "put" or "get", there are some others too)
3) similar statements to "put" and "get" with a bit of a change
4) a "close" statement to close the file once you're done with it.
code: |
var scores:array 1..10 of int %an array to store the scores
var fileno:int:=1
var filename:string:="textfile.txt" %remember, if you need to include a path, it'd be like "c:\\turing\\textfile.txt", you have to include that double \\
open:fileno, filename, get %this tells the computer to open the file with the name/path of "filename", and when you are going to use it, you'll be referring to it with the integer "fileno". The "get" tells the computer you'll be reading in information.
for count:1..10
get:fileno, scores(count) %this will read in the current line up to the first space and assign the scores(count) to it. if you had get:fileno, scores(count):* instead, it would read in the entire line
end for
close:fileno %this will close the text file, while possibly not required sometimes, it's good procedure. And sometimes if you don't do this, your program will not function properly *knows from personal experience... and took two days to figure out that was the reason a program wasn't working*
%then you can use a simple sorting algorithm to sort the numbers; if you want to store them in order, use an array of same length.
var orderedscores:array 1..10 of int
var highest, scorecount:int
%I didn't want to show the entire code, but I'm a bit tired and busy right now to explain it... you should be able to figure it out I hope
for count1:1..10
highest:=0
for count2:1..10
if scores(count2)>highest then
highest:=scores(count2)
scorecount:=count2
end if
end for
oderedscores(count1):=highest
scores(scorecount):=0
end for
%Then outputing back to a .txt file is similar to get:
open:fileno,filename, put %notice the "put" this time instead of "get"
for count3:1..10
put:fileno, orderedscores(count3)
end for
close:fileno %DON'T FORGET TO CLOSE! :P
|
More code than what I usually show, but I hope it helps. Try to understand what is written here and write your own code from scratch (even if it is similar to this) rather than just copying and pasting it. If you don't understand parts of it, PLEASE ask us. Otherwise you might be back here asking for the same help again. |
|
|
|
|
 |
FizixMan
|
Posted: Wed Dec 18, 2002 5:20 pm Post subject: (No subject) |
|
|
Darn you Tony!! DARN YOU!!!! lol, always posting at the same time as me! grrrrrrrrrrrrrrrrrrrrrrrrrrr  |
|
|
|
|
 |
Tony
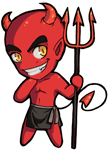
|
Posted: Wed Dec 18, 2002 5:21 pm Post subject: (No subject) |
|
|
lol, this time you got here a bit faster... I was just gonna say
*waits for FizixMan to get pissed* but then I saw your 2nd message
Hey, its cool... You got that sorting thing there  |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
WooFerPPK

|
Posted: Wed Dec 18, 2002 9:18 pm Post subject: (No subject) |
|
|
thanks alot |
|
|
|
|
 |
|
|