Insertion Sort For Words
Author |
Message |
Civik
|
Posted: Wed Feb 27, 2008 1:14 pm Post subject: Insertion Sort For Words |
|
|
Im trying to make a sort that sorts a list into alphabetical order, but also moves the other pieces of data that fit with the word thats being organized to be moved to the same location.
Basically what i mean is i am making a song database and i want to organize by name, but i want the artist, album, and year to also be moved to the same spot that the name is put to, so if the name goes to spot 5 the other info gets moved to spot 5 as well.
I have got the sort started based on other sorts on the site, im having problems though. As of right now im stuck.
Heres my code :
code: |
var name, artist, album, year : string
var fileout, filein, entries, x : int
x := 0
entries := 0
type musicrecord :
record
name : string
artist : string
album : string
year : int
end record
var musiclibrary : array 1 .. 100 of musicrecord
procedure openfile
open : filein, "songdatabase.txt", get
loop
x := x + 1
get : filein, musiclibrary (x).name
get : filein, musiclibrary (x).artist
get : filein, musiclibrary (x).album
get : filein, musiclibrary (x).year
entries := entries + 1
exit when eof (filein)
end loop
close : filein
end openfile
procedure switch (i, j : string)
const temp := musiclibrary (j).artist
for decreasing k : j .. i + 1
musiclibrary (k).artist := musiclibrary (k - 1).artist
end for
musiclibrary (x).year (i) := temp
end switch
procedure insertionSort ()
for j : 2 .. upper (musiclibrary.artist)
var i := 1
loop
exit when i = j or musiclibrary (i).artist >= musiclibrary (j).artist
i += 1
end loop
switch (i, j)
end for
end insertionSort
openfile
insertionSort (musiclibrary(x).year)
for i : 1 .. 10
put musiclibrary (i).artist : 5 ..
end for
|
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
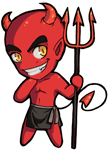
|
Posted: Wed Feb 27, 2008 1:20 pm Post subject: RE:Insertion Sort For Words |
|
|
the problem is that right now you are sorting only the artist entries, but what you want to do is to sort the entire musicrecord records.
a simple solution would be to shift records around within the musiclibrary array. Though if you are comfortable enough with arrays, it might make more sense to keep the library intact, and sort just the order indexes. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Civik
|
Posted: Wed Feb 27, 2008 1:22 pm Post subject: Re: Insertion Sort For Words |
|
|
Is it easier to just sort the whole record at once? How would i go about that? Ive been trying for a few days to get this to work so im down to try whatever. |
|
|
|
|
 |
Clayton

|
Posted: Wed Feb 27, 2008 1:27 pm Post subject: RE:Insertion Sort For Words |
|
|
Instead of sorting just artists, sort the entire record. Your swap would change from this:
Turing: |
var temp : string := musiclibrary (j).artist
%the rest
...
|
To:
Turing: |
var temp : musicrecord := musiclibrary (j) |
See the difference? Just be sure that you are still comparing the fields of the record against each other, so that you can still sort by some value. |
|
|
|
|
 |
Civik
|
Posted: Wed Feb 27, 2008 1:32 pm Post subject: RE:Insertion Sort For Words |
|
|
So my sort should look like this ?
code: |
procedure switch (i, j : string)
var temp : musicrecord := musiclibrary (j)
for decreasing k : j .. i + 1
musiclibrary (k) := musiclibrary (k - 1)
end for
musiclibrary (x).year (i) := temp
end switch
procedure insertionSort ()
for j : 2 .. upper (musiclibrary)
var i := 1
loop
exit when i = j or musiclibrary (i) >= musiclibrary (j)
i += 1
end loop
switch (i, j)
end for
end insertionSort
openfile
insertionSort (musiclibrary)
for i : 1 .. 10
put musiclibrary : 5 ..
end for
|
|
|
|
|
|
 |
Clayton

|
Posted: Wed Feb 27, 2008 3:37 pm Post subject: RE:Insertion Sort For Words |
|
|
One minor edit, see if you can spot it:
Turing: |
procedure insertionSort ()
for j : 2 .. upper (musiclibrary )
var i := 1
loop
exit when i = j or musiclibrary (i ).artist >= musiclibrary (j ).artist
i + = 1
end loop
switch (i, j )
end for
end insertionSort |
|
|
|
|
|
 |
Civik
|
Posted: Wed Feb 27, 2008 10:01 pm Post subject: RE:Insertion Sort For Words |
|
|
Im confused, you added .artist but am i not supposed to sort the whole record not just artist?
Also, the way i have my code currently, will that move the other parts of data to the same spot as well? like artist album and year get put in the right spot with the names as they are organized |
|
|
|
|
 |
Tony
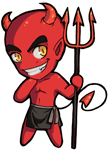
|
Posted: Thu Feb 28, 2008 12:02 am Post subject: RE:Insertion Sort For Words |
|
|
I think you need to step back and revisit the basics of records.
what is a record?
what does it mean that record_1 > record_2 ?
what does it mean that record_1.artist > record_2.artist ? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
|
|