Randomize Variable?
Author |
Message |
doubletime23
|
Posted: Thu Jan 03, 2008 10:28 pm Post subject: Randomize Variable? |
|
|
Hey, i was in the process of making a Black Jack game for my computer engineering class. I need to know how to randomize the 52 variables of cards i have. Example:
var hea2 : int
hea2 := 2
var hea3 : int
hea3 := 3
var hea4 : int
hea4 := 4
These are the variables for the 2, 3, and 4 of hearts. I did this for all 52 cards. How do u randomize these?? haha
Thanks alot for any help!  |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
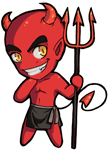
|
Posted: Thu Jan 03, 2008 10:38 pm Post subject: RE:Randomize Variable? |
|
|
I've love to know how you even think of building a Black Jack with variables such as hea4. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
doubletime23
|
Posted: Thu Jan 03, 2008 10:51 pm Post subject: Re: RE:Randomize Variable? |
|
|
Tony @ Thu Jan 03, 2008 10:38 pm wrote: I've love to know how you even think of building a Black Jack with variables such as hea4.
Its to stand for The 4 or Hearts. Why is that hard to understand. I really just need to know how to randomize a list of variables lol.  |
|
|
|
|
 |
HeavenAgain

|
Posted: Thu Jan 03, 2008 11:13 pm Post subject: RE:Randomize Variable? |
|
|
try using a for loop from 1 to however many cards you have (assuming you are using array) you can do this in 1 for loop from 0 to 51(52?)
code: | deck[ counter ] = new Card( faces[ counter % 13 ], suits[ counter div 13 ] ); |
this is where the first card is 0 and its on the top of our deck, and now 0 mod by 13 you get 0, (since Ace to King are 13 "values", and anything moded by x shall never be equal or greater than x) and the suit is 1 div 13, which returns an integer of 0, and as this loop goes on, all the cards will be assigned with a face value and a suit value
hope this helps  |
|
|
|
|
 |
doubletime23
|
Posted: Thu Jan 03, 2008 11:40 pm Post subject: Re: RE:Randomize Variable? |
|
|
HeavenAgain @ Thu Jan 03, 2008 11:13 pm wrote: try using a for loop from 1 to however many cards you have (assuming you are using array) you can do this in 1 for loop from 0 to 51(52?)
code: | deck[ counter ] = new Card( faces[ counter % 13 ], suits[ counter div 13 ] ); |
this is where the first card is 0 and its on the top of our deck, and now 0 mod by 13 you get 0, (since Ace to King are 13 "values", and anything moded by x shall never be equal or greater than x) and the suit is 1 div 13, which returns an integer of 0, and as this loop goes on, all the cards will be assigned with a face value and a suit value
hope this helps 
Thanks! Hopefully i can get er going. I'll put it up if i get it workin  |
|
|
|
|
 |
doubletime23
|
Posted: Thu Jan 03, 2008 11:45 pm Post subject: Re: RE:Randomize Variable? |
|
|
HeavenAgain @ Thu Jan 03, 2008 11:13 pm wrote: try using a for loop from 1 to however many cards you have (assuming you are using array) you can do this in 1 for loop from 0 to 51(52?)
code: | deck[ counter ] = new Card( faces[ counter % 13 ], suits[ counter div 13 ] ); |
this is where the first card is 0 and its on the top of our deck, and now 0 mod by 13 you get 0, (since Ace to King are 13 "values", and anything moded by x shall never be equal or greater than x) and the suit is 1 div 13, which returns an integer of 0, and as this loop goes on, all the cards will be assigned with a face value and a suit value
hope this helps 
O sorry, yea i already have all of my variables, i wrote out all 52 and gave them all values from 1/11(Ace) to 10, such as 2 of spades is worth 2 lol. But how do u randomize a list of things like that? Sorry, im new to turing Thanks! |
|
|
|
|
 |
HeavenAgain

|
Posted: Thu Jan 03, 2008 11:53 pm Post subject: RE:Randomize Variable? |
|
|
well, you shouldnt even have make 52 variables in the first place o.o
if you understand how to use array... it would be much simpler....
(still assuming you are using array)
the following code 99% will not work, but the concept is just like shovel the cards, you take the first card and put it somewhere in the deck, and take the next and etc etc till you reach the end
code: | for i : 1..52
deck[i] = swap(i, randint(1,52))
end for |
so click here |
|
|
|
|
 |
Tony
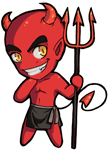
|
Posted: Fri Jan 04, 2008 1:09 pm Post subject: Re: RE:Randomize Variable? |
|
|
doubletime23 @ Thu Jan 03, 2008 11:45 pm wrote: But how do u randomize a list of things like that?
That is not a list. You cannot randomize what you have... I'm not even sure how you expect to play with that, thus my original question.
You know what hea4 has a value of 4... actually that's not even a variable, because I'm pretty sure that 4 of Hearts will always have a value of 4. So what exactly are you trying to randomize here? You don't even have a deck. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
Gooie
|
Posted: Sat Jan 05, 2008 1:03 am Post subject: Re: RE:Randomize Variable? |
|
|
Change the way you store variables. |
|
|
|
|
 |
|
|