Author |
Message |
oscarwu1991
|
Posted: Fri Nov 09, 2007 2:06 pm Post subject: Binary Search |
|
|
I need to make a program which is binary search
and that allows you to load the datafile
search populations and flight info
can someone help me with it?
really important
thanks |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Euphoracle

|
Posted: Fri Nov 09, 2007 3:25 pm Post subject: RE:Binary Search |
|
|
You should really attempt it yourself before asking for help. If this is for school, your teacher should have outlined the parameters you need to follow, shouldn't be that hard to at least get a trial program coded, right? |
|
|
|
|
 |
Tony
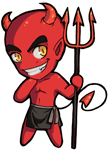
|
Posted: Fri Nov 09, 2007 3:28 pm Post subject: RE:Binary Search |
|
|
sure, what do you need help with? Reading from a file? Sorting? Searching? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
oscarwu1991
|
Posted: Sat Nov 10, 2007 1:18 pm Post subject: Re: Binary Search |
|
|
my teacher didnt show me how to do it
and now i have no clue how
so... |
|
|
|
|
 |
Tony
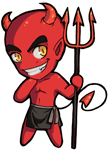
|
Posted: Sat Nov 10, 2007 3:09 pm Post subject: RE:Binary Search |
|
|
Once again, you have to be more specific. Or ask your teacher to show you.. I can only assume your teacher would know more about what's expected.
Anyway, all the binary search theory can be read about at Wikipedia |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
oscarwu1991
|
Posted: Sat Nov 10, 2007 3:55 pm Post subject: Re: Binary Search |
|
|
Sorry, but we have misplaced your code. The only way to safeguard your code is to place it in code tags. Sorry for the inconvienience.
%here is my code
but i dont know how to output the right answer when i input the correct name
i cannot use if condition
thanks |
|
|
|
|
 |
oscarwu1991
|
Posted: Sat Nov 10, 2007 4:14 pm Post subject: Re: Binary Search |
|
|
code: | var datafile:int
var population:array 1..11 of int
var country:array 1..11 of string
var sort:array 1..11 of int
var datafile1:int
proc hskg
for i : 1 .. 1
population(i):=0
get country(i)
end for
end hskg
proc data
open : datafile, "data8.3.t", get
for i : 1 .. 11
get : datafile,population(i),country(i)
end for
end data
proc data1
open : datafile1, "data8.2.t",get
for i:1..11
get:datafile1, population(i)
end for
end data1
proc load1
for i : 1 .. 11
sort (i) := population (i)
end for
end load1
proc bubble
var flag:string(1):="N"
var temp:int
loop
flag:="N"
for k:1..1
if sort (k) = sort (k+1) then
temp:=sort(k)
sort(k):=sort (k+1)
sort(k+1):=temp
flag:="Y"
end if
end for
if flag="N" then
exit
end if
end loop
end bubble
proc out
for i : 1 .. 1
put population(i)
end for
end out
%mainline
hskg
data
data1
load1
bubble
out
|
here is my code
pls help
but i dont know how to output the right answer when i input the correct name
i cannot use if condition
thanks[/quote] |
|
|
|
|
 |
RLien
|
Posted: Sat Nov 10, 2007 5:35 pm Post subject: RE:Binary Search |
|
|
umm weird question, are you oscar from mr saigals class because i have a project exactly like that too |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
oscarwu1991
|
Posted: Sat Nov 10, 2007 5:59 pm Post subject: Re: Binary Search |
|
|
someone help |
|
|
|
|
 |
Tony
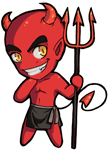
|
Posted: Sat Nov 10, 2007 6:21 pm Post subject: RE:Binary Search |
|
|
code: |
for k:1..1
...
for i : 1 .. 1
|
Is it really a loop, if the block gets executed just once?
Also
code: |
if sort (k) = sort (k+1) then
|
Are you looking for the same values in a set? Probably not, because you swap them afterwards (why, they are the same!) |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
oscarwu1991
|
Posted: Sat Nov 10, 2007 10:24 pm Post subject: Re: Binary Search |
|
|
but what should i do to complete my program?
i want to:
when i enter canada
it outputs the correct number
when i enter china
it outputs the correct number
how can i do that? |
|
|
|
|
 |
Tony
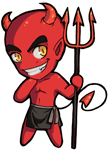
|
Posted: Sat Nov 10, 2007 10:28 pm Post subject: RE:Binary Search |
|
|
You can start by fixing your sort procedure.
when you do
code: |
proc out
for i : 1 .. 11
put population(i)
put country(i)
end for
end out
|
Make sure the numbers are put in the right order.
Also make sure that the countries have their correct population. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
oscarwu1991
|
Posted: Sat Nov 10, 2007 11:52 pm Post subject: Re: Binary Search |
|
|
no i mean
when i input china
it shows the population of china
and then cls
input other country again
output the correct population
only output one number |
|
|
|
|
 |
Tony
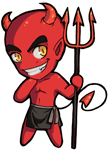
|
Posted: Sun Nov 11, 2007 4:25 am Post subject: RE:Binary Search |
|
|
well you will need an array with two pieces of data -- country name, and population. This way when you figure out the index for one, it will be the same index for another. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
oscarwu1991
|
Posted: Sun Nov 11, 2007 12:55 pm Post subject: Re: Binary Search |
|
|
I have 2 data file
one is for population
and the other is for country
can you tell me what to do to collect two parts?
i have two arrays already |
|
|
|
|
 |
|